How to comment multiple lines in Python Jupyter Notebook?
How to comment multiple lines in Python Jupyter Notebook?
I'm happy to help you with that!
In Python Jupyter Notebook, there are a few ways to comment multiple lines of code. Here are some common methods:
Method 1: Using the #
symbol
One way to comment multiple lines is by using the #
symbol at the beginning of each line. This method is simple and straightforward:
# This is the first line of a multi-line comment
It can span multiple lines
And still be a single comment
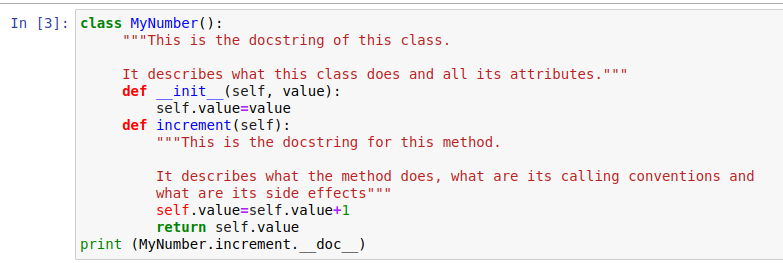
print("Hello, world!") # This print statement is not commented out
In this example, everything from #
to the end of the first line is considered a comment. The subsequent lines will also be interpreted as comments until another #
symbol is encountered.
**Method 2: Using triple quotes (""" or
``). This method allows you to comment out a block of code, including whitespace:
Another way to comment multiple lines is by using triple quotes (
"""
or
"""
This is the first line of a multi-line comment
It can span multiple lines and include indentation
And still be a single comment
"""
print("Hello, world!")
In this example, everything within the triple quotes ("""
) is considered a comment. The print
statement outside the comments will still be executed.
Method 3: Using the #
symbol with parentheses
A less common method to comment multiple lines involves using the #
symbol with parentheses:
# (This is the first line of a multi-line comment)
(It can span multiple lines and include indentation)
print("Hello, world!") # This print statement is not commented out
(And this line too)
In this example, the comment starts at the #
symbol, and the parentheses help to indicate that the comment spans multiple lines.
Best Practice: Use Consistent Commenting
While all these methods are valid ways to comment multiple lines in Python Jupyter Notebook, it's generally considered a best practice to use consistent commenting throughout your code. This means choosing one method (e.g., triple quotes) and sticking to it.
In conclusion, when you need to comment out multiple lines of code in Python Jupyter Notebook, you have three primary options: using the #
symbol, triple quotes ("""
or ````), or a combination of both. By following best practices and choosing a consistent commenting style, you can improve the readability and maintainability of your code.
I hope this helps!
Python comment multiple lines shortcut jupyter github
I'd be happy to help you with that!
Python Comment Multiple Lines Shortcut
In Python, when you need to add a multi-line comment, it can get quite cumbersome typing out #
for each line. Fortunately, there's a shortcut to make your life easier. Here are a few options:
"""
) to start a block comment, and Python will consider everything within those quotes as a single comment.
"""
This is a multi-line comment.
It spans multiple lines!
"""
Parentheses: Another option is using parentheses (
and )
to enclose your multi-line comment. This approach works similarly to the triple quote method:
(
This is another way to do
multi-line comments in Python
)
Carriage Return: If you're working on a Unix-based system (like Linux or macOS), you can use the carriage return character (n
) to separate your lines:
# This is the first line of my comment.
And this is the second line.
Keep going...
Jupyter Notebooks
Now that we've covered multi-line comments, let's talk about Jupyter Notebooks. Jupyter is an amazing platform for interactive data science and education. You can create and share notebooks in Python, R, or Julia.
When working with Jupyter, you might want to add comments to your cells. Here are some tips:
Magic Commands: Jupyter has a few magic commands that let you execute Python code within your notebook. You can use these to run specific cells, clear outputs, and more!# Run this cell
1 + 2
Clear previous output
%clear-output
Markdown Cells: Did you know that Jupyter Notebooks support Markdown cells? These cells allow you to include formatted text, including code blocks, in your notebook.
GitHub
Last but not least, let's talk about GitHub. As a developer, you likely use GitHub for version control and collaboration. Here are some tips:
Code Comments: When adding comments to your code on GitHub, remember that they should be concise and focused. Use//
or /* */
to add line-level comments. README Files: Don't forget about the README file! This is a crucial part of any open-source project, as it provides users with essential information about how to use your code. Code Organization: Keep your GitHub projects organized by structuring your files and folders logically. Use meaningful names for your branches, tags, and releases. Collaboration: When working on a project with others, make sure you understand the workflow and communication channels. Be respectful of your colleagues' time and expertise.
That's it! I hope this helps you with Python comments, Jupyter Notebooks, and GitHub. Happy coding!