Python JSON dumps
Python JSON dumps
I'd be happy to respond in English and provide information about Python's JSON (JavaScript Object Notation) dumping capabilities.
JSON is a popular data interchange format that is widely used for exchanging data between web servers, web applications, and mobile apps. In Python, we can use the json
module to convert Python objects into JSON strings, which are then easily readable by humans and machines alike.
To dump a Python object as JSON using the json
module, you can use the dumps()
function, which stands for "dump in standard format". Here's an example:
import json
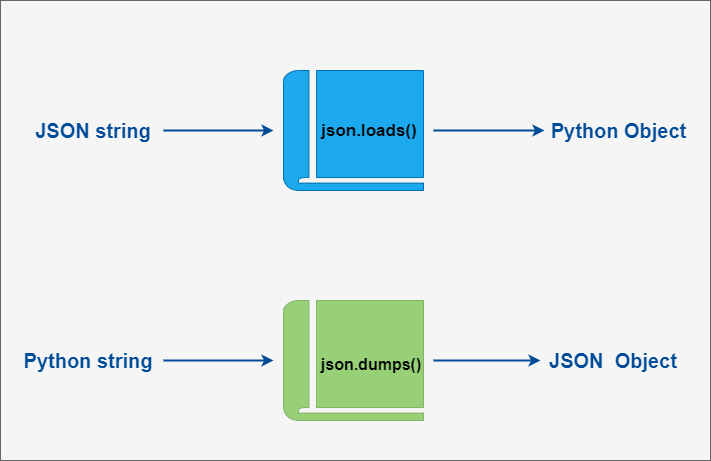
person = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
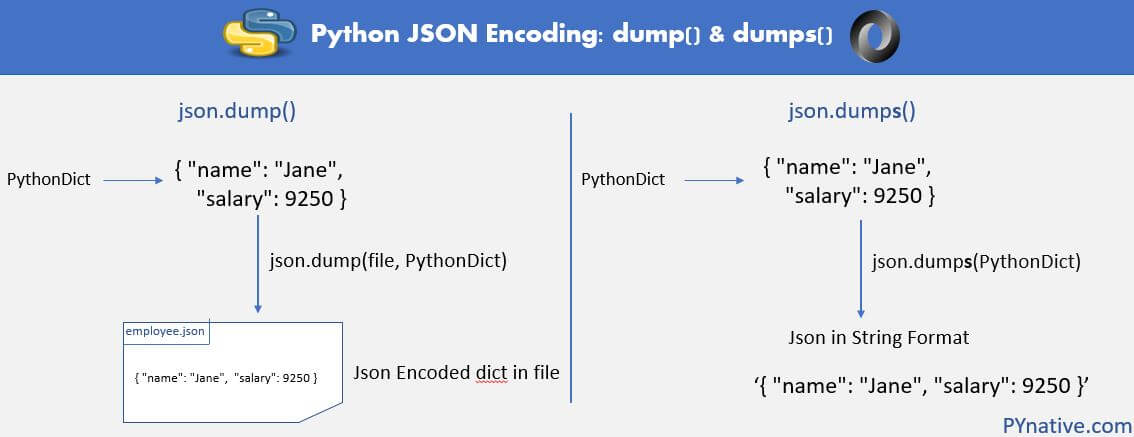
}
Convert person dictionary to JSON string
json_person = json.dumps(person)
print(json_person)
When you run this code, the output will be a JSON-formatted string:
{"name": "John Doe", "age": 30, "city": "New York"}
The dumps()
function takes an optional argument called indent
, which specifies the number of spaces to use for indentation. For example, if you set indent
to 4, the JSON output will be indented with four spaces:
import json
person = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
}
Convert person dictionary to JSON string with indentation
json_person = json.dumps(person, indent=4)
print(json_person)
This will produce the following output:
{
"name": "John Doe",
"age": 30,
"city": "New York"
}
As you can see, the JSON output is nicely formatted with indentation.
In addition to the dumps()
function, the json
module also provides the dump()
function for writing JSON data to a file. Here's an example:
import json
person = {
'name': 'John Doe',
'age': 30,
'city': 'New York'
}
Write person dictionary to JSON file
with open('person.json', 'w') as f:
json.dump(person, f)
print("JSON file written successfully!")
When you run this code, a new file called person.json
will be created in the same directory, containing the following contents:
{"name": "John Doe", "age": 30, "city": "New York"}
Overall, Python's json
module provides convenient functions for converting Python objects to JSON strings and files. Whether you're working with web applications, data science, or scientific computing, JSON is a widely used format that can help facilitate data exchange and processing.
How to write a JSON with Python?
Here's how you can create a JSON (JavaScript Object Notation) file using Python:
Why use JSON?
JSON is a lightweight data interchange format that is language-independent, making it easy to share and exchange data between different systems. It's commonly used for storing and retrieving data in web applications, such as when sending requests or responses between the client-side JavaScript code and server-side backend.
Creating a JSON file using Python
In this example, we'll create a simple JSON file with some sample data. We'll use the json
module that comes built-in with Python's standard library to achieve this.
Here are the steps:
Install thejson
module (if you haven't already): You don't need to install anything explicitly because the json
module is part of the Python Standard Library, meaning it's included by default when you install Python. Create a JSON file using a dictionary: We'll represent our data as a Python dictionary and then convert it into a JSON string.
Let's say we want to store some information about books in a JSON file. Here's what the dictionary representation might look like:
# Create a sample book list
book_list = [
{"title": "To Kill a Mockingbird", "author": "Harper Lee", "published": 1960},
{"title": "The Great Gatsby", "author": "F. Scott Fitzgerald", "published": 1925},
{"title": "Pride and Prejudice", "author": "Jane Austen", "published": 1813}
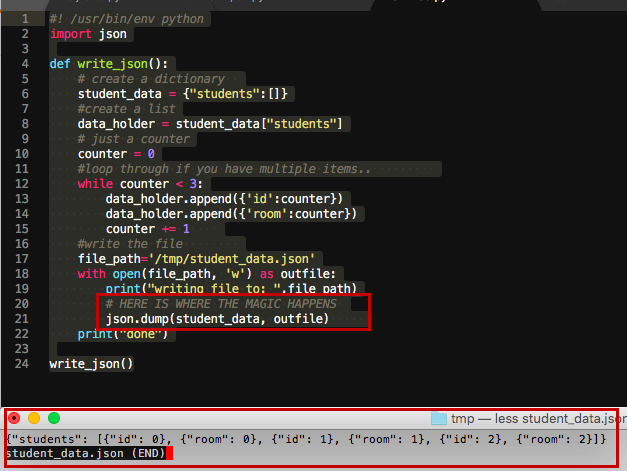
]
To convert this dictionary representation into a JSON string, we'll use the json.dumps()
function:
import json
Convert the dictionary to a JSON string
book_list_json = json.dumps(book_list)
print(book_list_json)
Output
When you run this code, you should see the following output:
[
{"title": "To Kill a Mockingbird", "author": "Harper Lee", "published": 1960},
{"title": "The Great Gatsby", "author": "F. Scott Fitzgerald", "published": 1925},
{"title": "Pride and Prejudice", "author": "Jane Austen", "published": 1813}
]
Saving the JSON to a file
To save this JSON string to a file, we can use Python's built-in open()
function in write mode ('w'
):
with open('book_list.json', 'w') as f:
f.write(book_list_json)
This will create a new file named book_list.json
and write the JSON string to it.
Reading the JSON from the file
If you want to read the JSON data back into your Python program, you can use the json.loads()
function:
with open('book_list.json', 'r') as f:
book_list_read = json.loads(f.read())
print(book_list_read)
This will read the JSON data from the file and convert it back into a Python dictionary. You can then manipulate this dictionary as needed.
That's it! You now know how to create, write, and read a JSON file using Python.
Remember, JSON is just one of many ways you can store and exchange data in your Python programs. Other options might include using SQLite databases or even relational databases like MySQL or PostgreSQL.