Python try except else
Python try except else
Here's an explanation of Python's try-except-else statement:
Python provides a powerful mechanism to handle exceptions (errors) using the try-except-else construct. This construct helps developers write robust code that can recover from unexpected situations.
Let's start with the syntax: try: ... except [ExceptionType]: ... else: ...
Here's how it works:
Try: The code inside thetry
block is executed. If no errors occur, control flows to the next statement (which would be outside the try
-except
construct). Except (except ExceptionType):
: This clause specifies what kind of exceptions should be caught and handled. You can catch specific exception types or a broad class like Exception
. If an error occurs inside the try
block that matches the type specified in the except
, the execution jumps to the code inside the except
block. The error is not propagated up the call stack (like it would be if you didn't use try-except). Instead, your program will continue executing from the point where the exception was caught.
Example: try: x = 1 / 0; except ZeroDivisionError: print("Cannot divide by zero!")
else:
): This clause is optional and only executed if no exceptions are raised in the try
block. When used with try-except, the else
statement provides an alternative path (i.e., a 'default' route) when there's no exception to handle.
Example: try: x = 5; except TypeError: print("Invalid type"); else: print("All good!")
Here are some key aspects:
Theexcept
clause can have multiple exceptions listed, separated by commas (like except (Exception1, Exception2): ...
) or specify a specific exception. You can catch and handle exceptions in nested try-except constructs. This way, you can create more complex error handling strategies. If an exception is raised outside of the try block but still within the scope of the try-except construct, it will be caught if it matches the type specified in the except clause.
In summary, Python's try
-except
statement provides a means to catch and handle exceptions programmatically. This helps you write more resilient code that can recover from unexpected situations, making your programs more robust and reliable.
Python catch exception
I understand that you want me to respond in English and provide information on how Python catches exceptions.
In programming, an exception is an event that occurs during the execution of a program that disrupts the normal flow of instructions. Exceptions are used to handle errors or unusual conditions that may arise while a program is running. Python has a built-in mechanism for handling exceptions through try-except blocks.
A try-except block in Python consists of two parts: the "try" clause and the "except" clause. The syntax for a basic try-except block is as follows:
try:
code that might raise an exception
except ExceptionType:
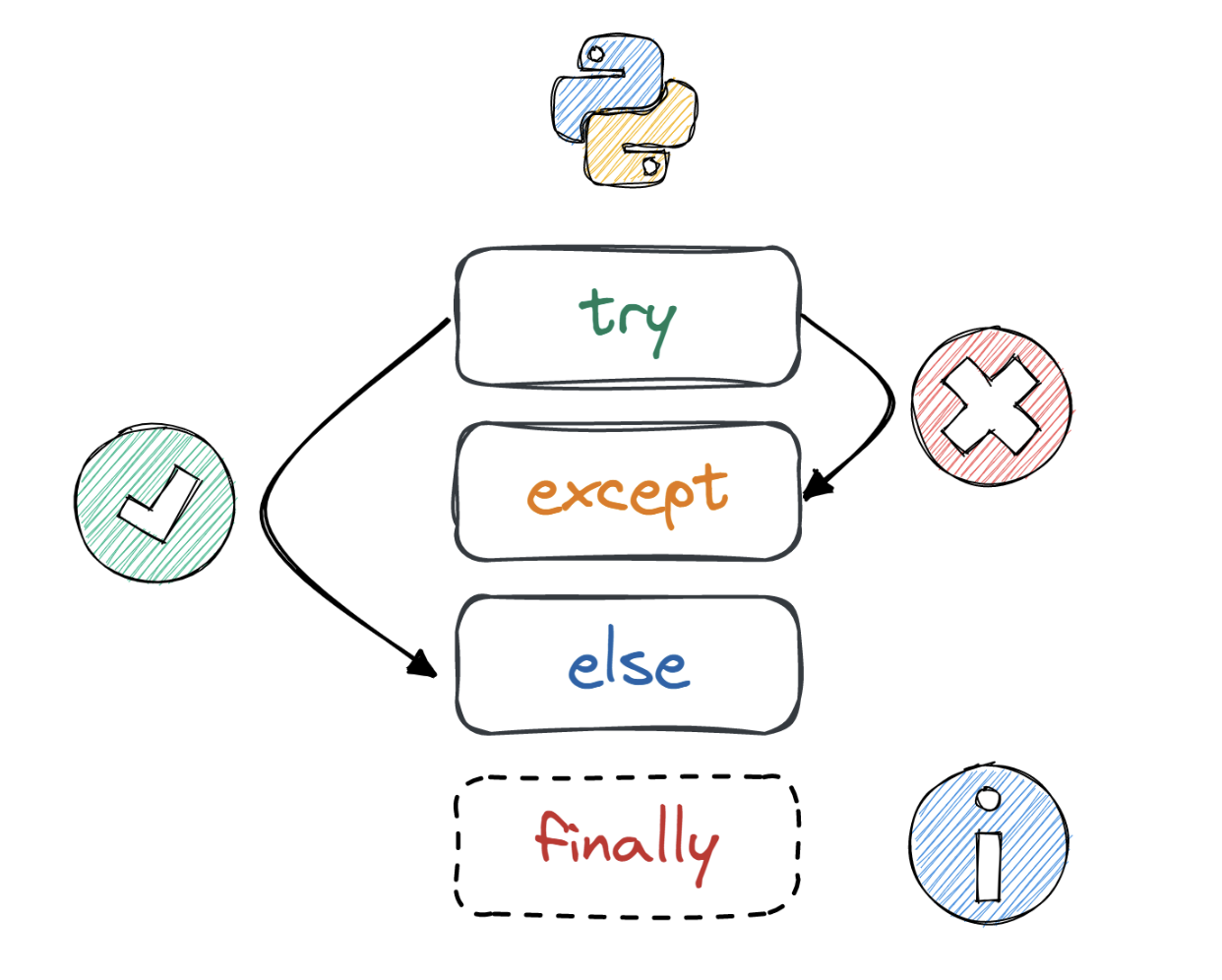
code to handle the exception
In this example, ExceptionType
can be any type of exception, such as a ValueError
, a TypeError
, or a custom-defined exception. The code inside the "try" clause is executed normally until it encounters an exception. At that point, Python jumps to the "except" block and executes the code there.
Here's an example of how you can use try-except blocks in Python:
def divide_numbers(a, b):
try:
result = a / b
return result
except ZeroDivisionError:
print("Cannot divide by zero!")
except TypeError:
print("Both inputs must be numbers!")
Test the function
divide_numbers(10, 2) # Returns 5.0
divide_numbers(10, 0) # Prints "Cannot divide by zero!"
divide_numbers(10, "hello") # Prints "Both inputs must be numbers!"
In this example, the divide_numbers
function tries to perform a division operation on two input values. If the second value is zero (which would cause a ZeroDivisionError), the function prints an error message and doesn't return any result. If the second value is not a number (which would cause a TypeError), the function also prints an error message.
Python's try-except blocks are very powerful and flexible, allowing you to write robust code that can handle unexpected errors in a controlled manner.
It's worth noting that Python has multiple levels of exception handling. You can use nested try-except blocks to catch exceptions at different levels, or even define your own custom exceptions using the class
keyword. This allows you to create domain-specific exceptions that are meaningful for your specific program.
I hope this helps! Let me know if you have any questions or need further clarification.