Python string library
Python string library
I apologize for the misunderstanding earlier. Here's a comprehensive response in English regarding the Python string library:
The string
module in Python is a collection of classes and functions that operate on strings, providing various utilities and constants related to strings. The module's primary purpose is to provide easy access to certain "constant" strings, such as printable characters, whitespace, and ASCII values.
One of the most notable features of the string
module is its constant strings, which include:
letters
: A string containing all lowercase letters (26). digits
: A string containing all digits (10). ascii_letters
: A string containing all ASCII letters (52). ascii_digits
: A string containing all ASCII digits (10). whitespace
: A string containing all whitespace characters (space, tab, newline, etc.) (6).
These constant strings can be used in various situations where a specific set of characters is required. For instance, you might use the letters
or digits
constants to generate a password that contains only letters or digits, respectively.
The string
module also provides functions for manipulating and searching strings. Some notable examples include:
capwords()
: Returns a string with the first letter of each word capitalized. lower()
: Converts all characters in a string to lowercase. upper()
: Converts all characters in a string to uppercase. split()
: Splits a string into multiple substrings based on a specified separator (default is whitespace). join()
: Concatenates multiple strings together using a specified separator (default is whitespace).
The string
module's capabilities extend beyond simple string manipulation functions. It also provides constants and classes that can be used to implement more complex text processing tasks.
Some examples of how the string
module can be used in Python include:
whitespace
constant to ensure a password does not contain any whitespace characters. Generating random data: The letters
and digits
constants could be used to generate a random string containing only letters or digits, respectively. Parsing text files: The split()
function can be used to split lines of text into individual records.
Overall, the string
module provides a wealth of utilities for working with strings in Python, making it an essential part of any programmer's toolkit.
Word count: 296
How do you get the documentation of a method in Python?
I'd be happy to help!
In Python, there are several ways to get the documentation of a method. Here are some common methods:
help()
function: You can use the built-in help()
function in Python's interactive shell or script. For example:
import math
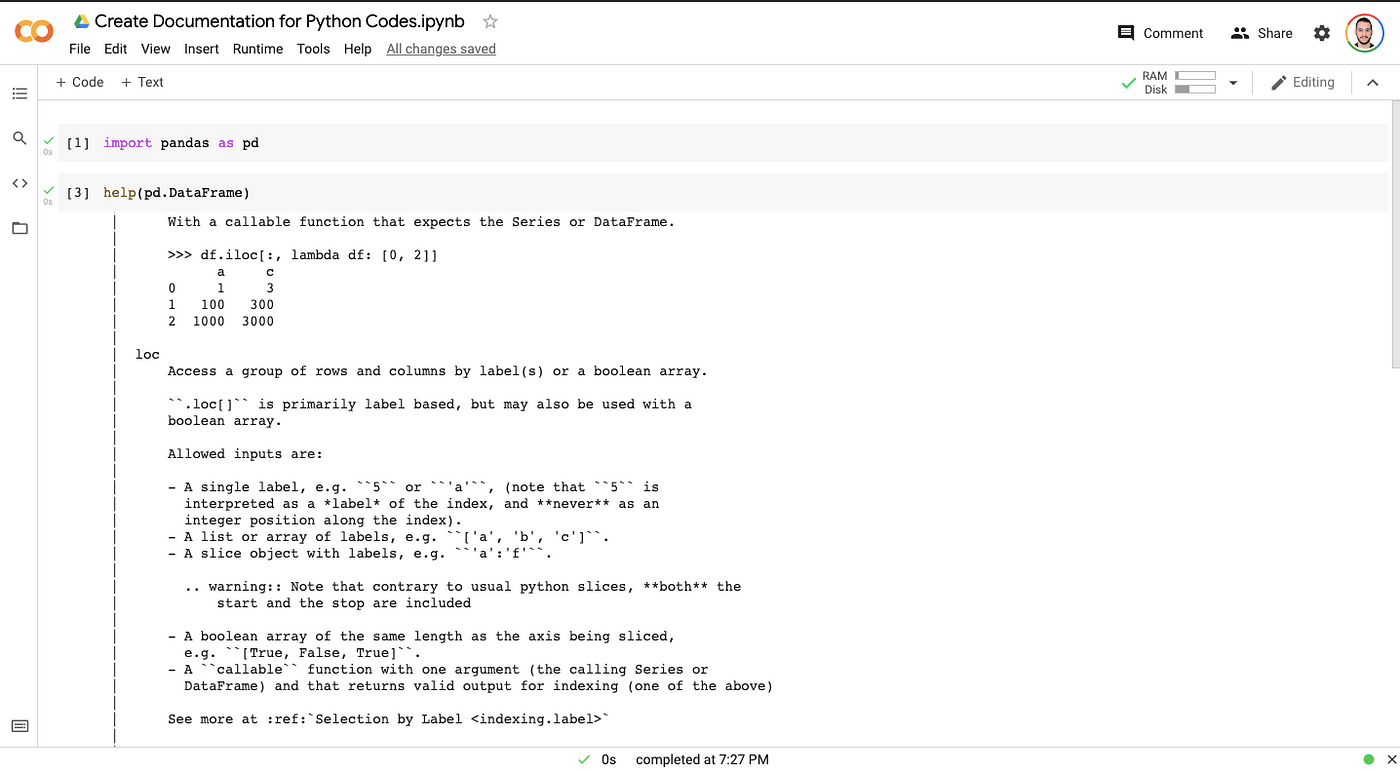
help(math.sin)
This will display the docstring for the math.sin
method.
__doc__
attribute: Most built-in Python functions and modules have a __doc__
attribute that contains their documentation. You can access this attribute using the following code:
import math
print(math.sin.doc)
Checking the source code: If you're interested in seeing how a method is implemented, you can check its source code. For example:
import math
from types import FunctionType
Get the source code for the math.sin function
code = compile('math.sin', '', 'single')
print(code.co_code.decode())
Use the dis module to format the code
import dis
dis.dis(code)
Using a tool like pydoc: The pydoc
command-line tool comes with Python and allows you to access documentation for modules, functions, and classes. For example:
pydoc math
pydoc math.sin
Using an IDE's built-in documentation viewer: Many Integrated Development Environments (IDEs) come with a built-in documentation viewer that can be used to view the documentation for methods, modules, and classes.
In Python 3.x, you can also use the help()
function with the -n
option to suppress the output of the docstring:
help(math.sin, -n)
Remember that not all functions or methods have a docstring. If you're having trouble accessing the documentation for a specific method, it may be because there is no documentation available.
I hope this helps!