Types of scope in python with examples
Types of scope in python with examples
I'd be happy to explain types of scope in Python!
What is Scope?
In programming, scope refers to the region of a program where a variable is defined and accessible. In other words, it's the area of the code where you can use a specific variable without any issues.
Types of Scope in Python:
Local Scope: Local scope refers to a variable that is defined within a function or method. This means that the variable is only accessible within that function and its sub-functions.Example:
def my_function():
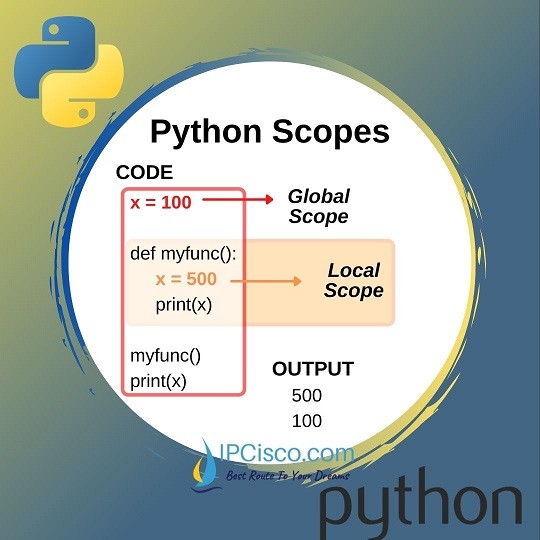
x = 10
print(x)
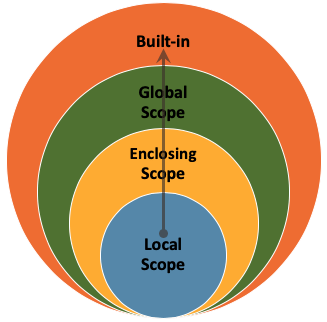
my_function() # Output: 10
print(x) # Error: x is not defined
In this example, x
is a local variable that's only accessible within the my_function
. Trying to access it outside the function will result in an error.
Example:
x = 10
def my_function():
print(x)
my_function() # Output: 10
print(x) # Output: 10
In this example, x
is a global variable that's defined at the top level. Both within and outside the function, we can access it without any issues.
Example:
module_name = "my_module"
def my_function():
print(module_name)
import my_module
print(my_module.module_name) # Error: module_name is not defined
In this example, module_name
is a module-level variable. While it's accessible within the my_module
, trying to access it from outside will result in an error.
self.
).
Example:
class MyClass:
x = 10
def my_method(self):
print(self.x)
my_obj = MyClass()
print(my_obj.x) # Output: 10
In this example, x
is a class-level variable that's defined within the MyClass
. We can access it using dot notation (self.
) from within the class or object.
Example:
def outer():
x = 10
def inner():
print(x)
inner()
outer() # Output: 10
In this example, x
is a variable from the enclosing scope (outer
) that's accessible within the inner scope (inner
). This demonstrates how variables in an outer scope can be accessed by inner scopes.
Conclusion:
Scope plays a crucial role in Python programming. Understanding local, global, module, class, and enclosing scopes helps you write more readable, maintainable, and efficient code. Remember to use scope correctly to avoid naming conflicts and ensure your program runs smoothly!
What are the 4 scope rules in Python?
I'll be happy to explain the scope rules in Python!
In Python, a scope refers to the region of code where a variable is defined and can be accessed. Python has two types of scopes: local scope and global scope.
1. Local Scope (also known as Function Scope)
Variables defined inside a function are considered local variables, which means they can only be accessed within that function. When a function returns, its local variables disappear. This is known as the "lifetime" of the variable. Here's an example:
def my_function():
x = 10 # Local variable
print(x) # Error! x is not defined here
my_function()
print(x) # Still error!
In this example, x
is a local variable defined inside the my_function()
function. After the function returns, x
ceases to exist.
2. Global Scope
Variables defined at the top level of a Python file (outside any functions or classes) are considered global variables. They can be accessed anywhere in the program, including within functions and other scope levels. Here's an example:
x = 10 # Global variable
def my_function():
print(x) # Accessing global variable
my_function()
print(x) # Still accessing the same global variable
In this example, x
is a global variable defined outside any function or class. It can be accessed within functions and other scope levels.
3. Nonlocal Scope (Python 3.x only)
In Python 3.x, you can use the nonlocal
keyword to access variables from an outer scope. This allows you to modify variables in a containing scope.
def outer():
x = 10
def inner():
nonlocal x # Accessing outer scope variable
x = 20
inner()
print(x) # Prints 20
outer() # Calls the outer function and its inner function
In this example, inner()
is a function within outer()
. The nonlocal
keyword allows inner()
to modify the x
variable from the outer scope.
4. Enclosing Scope (Python 2.x only)
In Python 2.x, you can use the global
or nonlocal
statement to access variables from an enclosing scope. This is similar to the nonlocal scope in Python 3.x.
def outer():
x = 10
def inner(x):
global x # Accessing outer scope variable
x = 20
inner(10)
print(x) # Prints 20
outer() # Calls the outer function and its inner function
In this example, inner()
is a function within outer()
. The global
statement allows inner()
to modify the x
variable from the outer scope.
To summarize:
Local Scope: variables defined inside a function are local and can only be accessed within that function. Global Scope: variables defined outside any functions or classes are global and can be accessed anywhere in the program. Nonlocal Scope (Python 3.x only): allows you to access and modify variables from an outer scope using thenonlocal
keyword. Enclosing Scope (Python 2.x only): allows you to access and modify variables from an enclosing scope using the global
or nonlocal
statement.
Understanding these scope rules is essential for writing effective and efficient Python code.