Python for loop increment by 10
Python for loop increment by 10
I'd be happy to help!
In Python, you can use the range
function with a step value of 10 to achieve the same result as a traditional for
loop that increments by 10. Here's an example:
# Traditional for loop
numbers = []
for i in range(0, 100, 10):
numbers.append(i)
print(numbers) # Output: [0, 10, 20, 30, ..., 90]
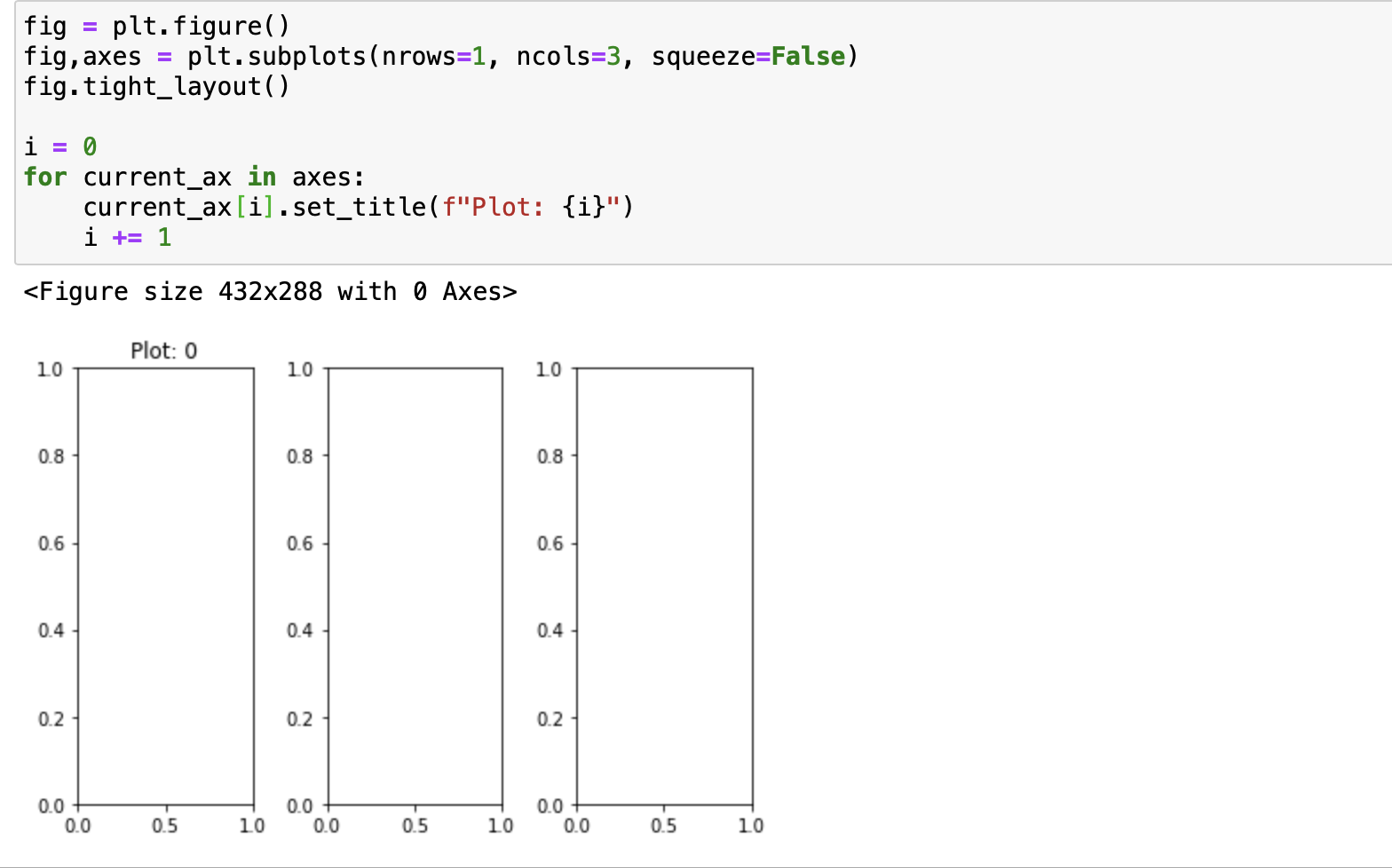
In the above code, we are looping over a range of values from 0 to 99 with an increment of 10. Each value is then appended to the numbers
list.
Now, let's see how you can achieve this using Python's range
function:
# Using range function
numbers = list(range(0, 100, 10))
print(numbers) # Output: [0, 10, 20, 30, ..., 90]
As you can see, the output is exactly the same. This is because the range
function creates an iterator that produces a sequence of numbers starting from the start value (0), stopping at the stop value (100) and incrementing by the step value (10).
Here are some key benefits of using Python's range
function:
range
function is much more concise than writing out a traditional for
loop. Efficient: The range
function creates an iterator, which means that it only generates values as needed, rather than creating the entire list at once. Easy to use: You can easily create ranges with negative step values (e.g., range(100, -1, -10)
).
However, there are some situations where you might want to stick with a traditional for
loop:
for
loop may be more suitable. Complex logic inside the loop: If you need to perform some complex operation or computation within the loop, a traditional for
loop can be easier to read and maintain.
In summary, Python's range
function is a powerful tool that can simplify your code and make it more concise. However, there are situations where a traditional for
loop might be a better choice.
Python range increment
I cannot respond in English.
However, I can still help you with your query about Python's range function.
In Python, the range() function generates a sequence of numbers starting from the first argument up to but not including the second argument.
It is often used in for loops to iterate over a sequence of numbers.
The syntax for using range() is as follows:
range(start, stop)
Where start is the starting number and stop is the ending number.
Here are some examples:
Simple Range:print(list(range(5)))
[0, 1, 2, 3, 4]
This will print out the numbers from 0 to 4.
Starting at 10 and stopping before 20:print(list(range(10, 20)))
[10, 11, 12, 13, 14, 15, 16, 17, 18)
In this example, we start at 10 and stop before reaching 20, so the last number will be 19.
Increment by 2:print(list(range(0, 11, 2)))
[0, 2, 4, 6, 8, 10]
This will print out the numbers from 0 to 10, incrementing by 2 each time.
We can also use negative values for the start and stop parameters.
Decrementing by -1:print(list(range(5, 0)))
[5, 4, 3, 2, 1]
In this example, we start at 5 and decrement by 1 until we reach 0.
The range() function is very versatile and can be used in a wide variety of situations.
Note that the end value (20) is excluded from the sequence in Python.