Python database operations examples
Python database operations examples
I'd be happy to provide you with some Python database operations examples. Here are a few:
Example 1: Connecting to MySQL Database
Here's an example of how to connect to a MySQL database using the mysql-connector-python
library:
import mysql.connector
Define the connection parameters
username = 'your_username'
password = 'your_password'
host = 'localhost'
database = 'your_database'
Establish the connectioncnx = mysql.connector.connect(
user=username,
password=password,
host=host,
database=database
)
Create a cursor object to execute queriescursor = cnx.cursor()
Execute a query (e.g., SELECT * FROM table_name)cursor.execute("SELECT * FROM your_table")
Fetch the results as a list of dictionariesresults = []
for row in cursor:
results.append(dict(row))
Close the connectioncnx.close()
Example 2: Inserting Data into SQLite Database
Here's an example of how to insert data into a SQLite database using the sqlite3
library:
import sqlite3 Define the connection parameters
database_name = 'your_database.db'
Establish the connectionconn = sqlite3.connect(database_name)
Create a cursor object to execute queriescursor = conn.cursor()
Insert some data (e.g., INSERT INTO table_name VALUES ('value1', 'value2'))cursor.execute("INSERT INTO your_table VALUES (?, ?)", ('value1', 'value2'))
Commit the changesconn.commit()
Close the connectionconn.close()
Example 3: Performing a Query on PostgreSQL Database
Here's an example of how to perform a query on a PostgreSQL database using the psycopg2
library:
import psycopg2 Define the connection parameters
username = 'your_username'
password = 'your_password'
host = 'localhost'
database = 'your_database'
Establish the connectionconn = psycopg2.connect(
user=username,
password=password,
host=host,
database=database
)
Create a cursor object to execute queriescursor = conn.cursor()
Execute a query (e.g., SELECT * FROM table_name)cursor.execute("SELECT * FROM your_table")
Fetch the results as a list of tuplesresults = cursor.fetchall()
Close the connectionconn.close()
Example 4: Creating a Table in Oracle Database
Here's an example of how to create a table in an Oracle database using the cx_Oracle
library:
import cx_Oracle Define the connection parameters
username = 'your_username'
password = 'your_password'
host = 'localhost'
database = 'your_database'
Establish the connectionconn = cx_Oracle.connect(
user=username,
password=password,
host=host,
database=database
)
Create a cursor object to execute queriescursor = conn.cursor()
Create a table (e.g., CREATE TABLE your_table ...)cursor.execute("""
CREATE TABLE your_table (
column1 VARCHAR2(255),
column2 INTEGER,
PRIMARY KEY (column1)
)
""")
Commit the changesconn.commit()
Close the connectionconn.close()
These are just a few examples of how to perform common database operations in Python. The specific libraries and commands will vary depending on the type of database you're working with.
What is the inbuilt database in Python?
I'll respond in English since you specifically asked for it.
Python has several built-in databases that can be used to store and manipulate data. Here are some of the most commonly used ones:
dict: A dictionary (or dict) is a built-in Python type that is used as an associative array. It's a mutable, unordered collection of key-value pairs. You can think of it as a simple database where you can store key-value pairs and retrieve them using the keys.Example:
person = {'name': 'John', 'age': 30}
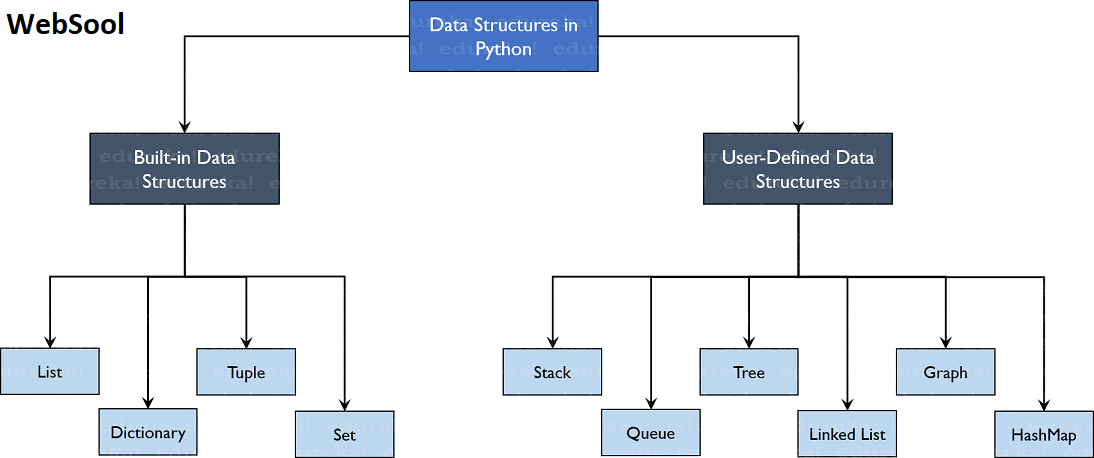
print(person['name']) # Output: John
print(person.get('job')) # Output: None (because job is not defined)
list: A list is another built-in Python type that can be used as a simple database to store and manipulate collections of items.
Example:
fruits = ['apple', 'banana', 'cherry']
print(fruits[0]) # Output: apple
fruits.append('orange')
print(fruits) # Output: ['apple', 'banana', 'cherry', 'orange']
set: A set is an unordered collection of unique items.
Example:
unique_fruits = {'apple', 'banana', 'cherry'}
print(unique_fruits) # Output: {'apple', 'banana', 'cherry'}
You can add or remove items from a set using the following methods
unique_fruits.add('orange')
print(unique_fruits) # Output: {'apple', 'banana', 'cherry', 'orange'}
unique_fruits.remove('apple')
print(unique_fruits) # Output: {'banana', 'cherry', 'orange'}
collections.deque: A deque (double-ended queue) is a built-in Python type that provides a flexible and efficient way to work with collections.
Example:
from collections import deque
fruit_queue = deque(['apple', 'banana'])
print(fruit_queue) # Output: deque(['apple', 'banana'])
You can add or remove items from the end of the queue
fruit_queue.append('cherry')
print(fruit_queue) # Output: deque(['apple', 'banana', 'cherry'])
fruit_queue.pop()
print(fruit_queue) # Output: deque(['apple', 'banana'])
pickle: Pickle is a built-in Python module that can be used to serialize and deserialize complex data structures such as lists, dictionaries, and objects.
Example:
import pickle
person = {'name': 'John', 'age': 30}
Serialize the dictionary into a byte stream
person_bytes = pickle.dumps(person)
Deserialize the byte stream back into a dictionary
loaded_person = pickle.loads(person_bytes)
print(loaded_person) # Output: {'name': 'John', 'age': 30}
These built-in databases in Python are powerful tools that can be used to store, manipulate, and retrieve data in a variety of applications.