How do you take a string input in Python?
How do you take a string input in Python?
I'm happy to help!
In Python, taking a string input from the user is a common task. There are several ways to achieve this, and I'll cover some of the most popular methods.
Method 1: Using input()
Function
The simplest way to get a string input from the user is by using the built-in input()
function. This function waits for the user to enter some text and then returns it as a string. Here's an example:
user_input = input("Please enter your name: ")
print("Hello, " + user_input + "! Welcome to our program.")
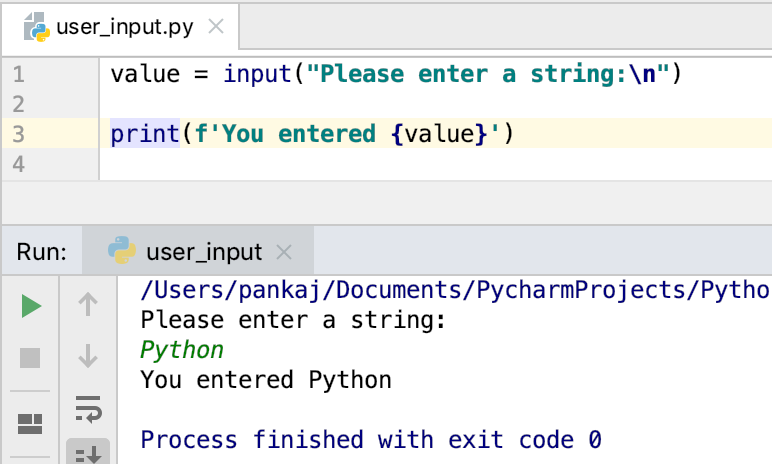
In this code, input()
prompts the user to enter their name, and when they do, it stores that value in the user_input
variable. The program then prints out a greeting message using the entered name.
Method 2: Using raw_input()
Function (Python 2.x only)
For Python 2.x versions, you can use the raw_input()
function instead of input()
. Both functions serve the same purpose, but raw_input()
is more accurate since it correctly identifies the user's input as a string:
user_input = raw_input("Please enter your name: ")
print("Hello, " + user_input + "! Welcome to our program.")
Method 3: Using sys.stdin.read()
Function
Another way to take a string input is by using the sys
module and its stdin
attribute. This method reads from the standard input stream (usually the console) until it encounters a newline character (n
). Here's how you can do it:
import sys
user_input = sys.stdin.read().strip()
print("Hello, " + user_input + "! Welcome to our program.")
Method 4: Using readline()
Function
The readline()
function from the builtins
module (Python 2.x) or io
module (Python 3.x) reads a line of text from the console and returns it as a string. You can use this method like so:
import builtins
user_input = builtins.input("Please enter your name: ")
print("Hello, " + user_input + "! Welcome to our program.")
Or, in Python 3.x:
import io
user_input = input("Please enter your name: ")
print("Hello, " + user_input + "! Welcome to our program.")
In all of these examples, the user_input
variable will hold the entered string. You can then use this variable as needed in your program.
Additional Tips and Best Practices
When working with input strings, it's essential to consider potential issues like:
Error handling: Always validate and handle unexpected user inputs to prevent errors or crashes. Input validation: Ensure that the entered data is in the expected format or meets specific criteria (e.g., checking if a password contains only letters and digits). Escaping special characters: Be aware of special characters like newline (n
) or carriage return (r
) and consider using methods like strip()
to remove them from the input. User experience: Provide clear instructions, feedback, and guidance throughout your program's interaction with users.
By following these guidelines and choosing the best method for your specific use case, you can create effective and user-friendly string input handling in Python!
How do you input a string to a set in Python?
I'd be happy to help!
In Python, you can add an element (in this case, a string) to a set using the add()
method or the update()
method. Both methods allow you to modify the existing set by adding one or multiple elements.
Using the add()
Method
The add()
method is used to insert a single element into a set. Here's an example:
my_set = {'apple', 'banana'}
my_string = 'orange'
my_set.add(my_string)
print(my_set) # Output: {'apple', 'banana', 'orange'}
In this example, the add()
method adds the string 'orange'
to the existing set my_set
. Note that sets are unordered and do not allow duplicate values.
Using the update()
Method
The update()
method is used to insert multiple elements into a set. You can pass it a list, tuple, or another set (or any iterable) containing the new elements:
my_set = {'apple', 'banana'}
new_elements = ['orange', 'grape']
my_set.update(new_elements)
print(my_set) # Output: {'apple', 'banana', 'orange', 'grape'}
In this example, the update()
method adds all the elements in the new_elements
list to the existing set my_set
.
Using a Fomula
Another way to add an element to a set is using a formula with the {}
syntax:
my_set = {'apple', 'banana'}
my_string = 'orange'
my_set = {element for element in my_set if element != my_string} | {my_string}
print(my_set) # Output: {'apple', 'banana', 'orange'}
In this example, the {}
syntax creates a new set containing all elements from my_set
except for my_string
, and then combines it with a set containing only my_string
. This effectively adds my_string
to my_set
.
These are some ways to add an element (in this case, a string) to a set in Python. The best method depends on your specific use case and the size of your data.
Thank you for asking!