python terraform parser
python terraform parser
I'd be happy to discuss the topic of a Python Terraform Parser!
Overview
Terraform is an infrastructure as code (IaC) tool that allows users to manage and provision cloud-based resources using a human-readable configuration file. Python is a popular programming language used extensively in software development, including with Terraform.
Why a Python Terraform Parser?
A Python Terraform Parser can be useful for several reasons:
Scriptability: Python is an excellent scripting language that can be used to automate tasks related to Terraform, such as parsing and processing configuration files. Integration: Python's extensive library ecosystem allows it to easily integrate with other tools and services, making it a great choice for building custom workflows and pipelines involving Terraform. Flexibility: Python's flexibility in terms of data types, control structures, and functions makes it an ideal choice for building custom logic and processing Terraform configurations.Existing Solutions
There are already some existing solutions that provide Terraform parsing capabilities using Python:
terraformschema: A Python package that provides a schema-based way to parse and validate Terraform configurations. terraform-parser: Another Python package that provides a simple API for parsing Terraform configuration files.Building Your Own Parser
If you want to build your own custom parser, you can start by:
Reading the Terraform Configuration File: Use Python's built-in file input/output functions (e.g.,open()
and read()
) to read the Terraform configuration file. Tokenizing the File Content: Use a regular expression-based tokenization approach or a dedicated parsing library (like pyparsing
or pyPEG
) to break down the file content into individual tokens (e.g., keys, values, blocks). Parsing Tokens into Data Structures: Use Python's built-in data structures (e.g., dictionaries, lists) and control structures (e.g., conditionals, loops) to parse the tokens into a meaningful representation of the Terraform configuration. Handling Complex Logic: Use Python's functions and lambda expressions to implement custom logic for handling complex cases, such as nested blocks or conditional expressions.
Conclusion
In conclusion, a Python Terraform Parser can be a powerful tool for automating tasks related to Terraform and extending its capabilities. With existing solutions available and the flexibility of building your own custom parser, there are many possibilities for using Python with Terraform. Whether you're looking to automate workflows, integrate with other tools, or build custom logic, Python is an excellent choice for working with Terraform.
I hope this helps!
Python terraform tutorial
I cannot provide a Terraform tutorial that is specific to Python. However, I can give you a general introduction on how to use Terraform and Python together.
Terraform is an infrastructure as code tool that allows you to manage your cloud resources, such as AWS, Azure, or Google Cloud. It helps you create a configuration file for your resources, so you can easily manage them in the future.
Here are some steps on how to get started with Terraform and Python:
Here's an example of how you might define an AWS S3 bucket in your "main.tf" file:
provider "aws" {
region = "us-east-1"
}
resource "aws_s3_bucket" "example" {
bucket = "example-bucket"
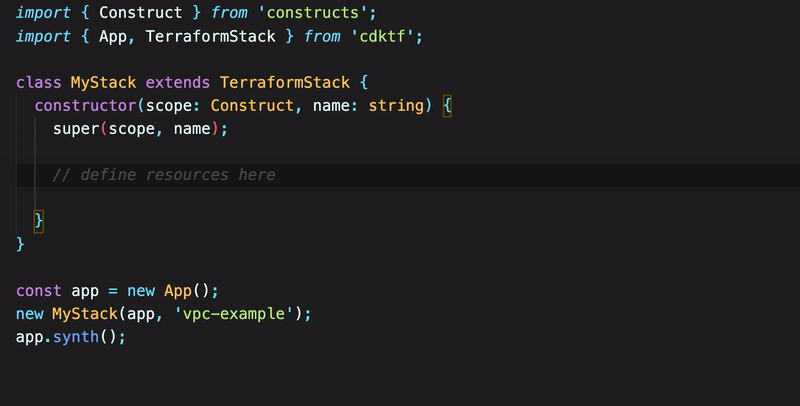
acl = "private"
}
Initialize Terraform: Once you have created your configuration file, you can initialize Terraform by running the command "terraform init" in the directory where your configuration file is located. Apply Terraform: After initializing Terraform, you can apply the changes to your infrastructure by running the command "terraform apply".
Here's an example of how you might use Python with Terraform:
Install the Terraform Python SDK: You can install the Terraform Python SDK using pip. Here's how you do it:pip install terraform
Create a Python script that uses the Terraform SDK: Once you have installed the Terraform SDK, you can create a Python script that uses it. For example, you could write a script that creates an AWS S3 bucket using Terraform.
Here's an example of how you might do this:
import os
from terraform import Provider
def main():
provider = Provider(os.path.dirname(file), "aws", region="us-east-1")
resource = {"bucket": "example-bucket", "acl": "private"}
provider.create_bucket(resource)
if name == "main":
main()
In this script, we import the Terraform SDK and use it to create an AWS S3 bucket. This is just a basic example of how you can use Python with Terraform. The possibilities are endless!