How to make a triangle in Python?
How to make a triangle in Python?
I'd be happy to help you with that!
In Python, you can create a triangle using various methods depending on your specific requirements and the level of complexity you're comfortable with. Here are some approaches:
Method 1: Using ASCII Art
One simple way to draw a triangle is by using ASCII art characters. You can use a combination of /
and to create a triangle shape.
def print_triangle(n):
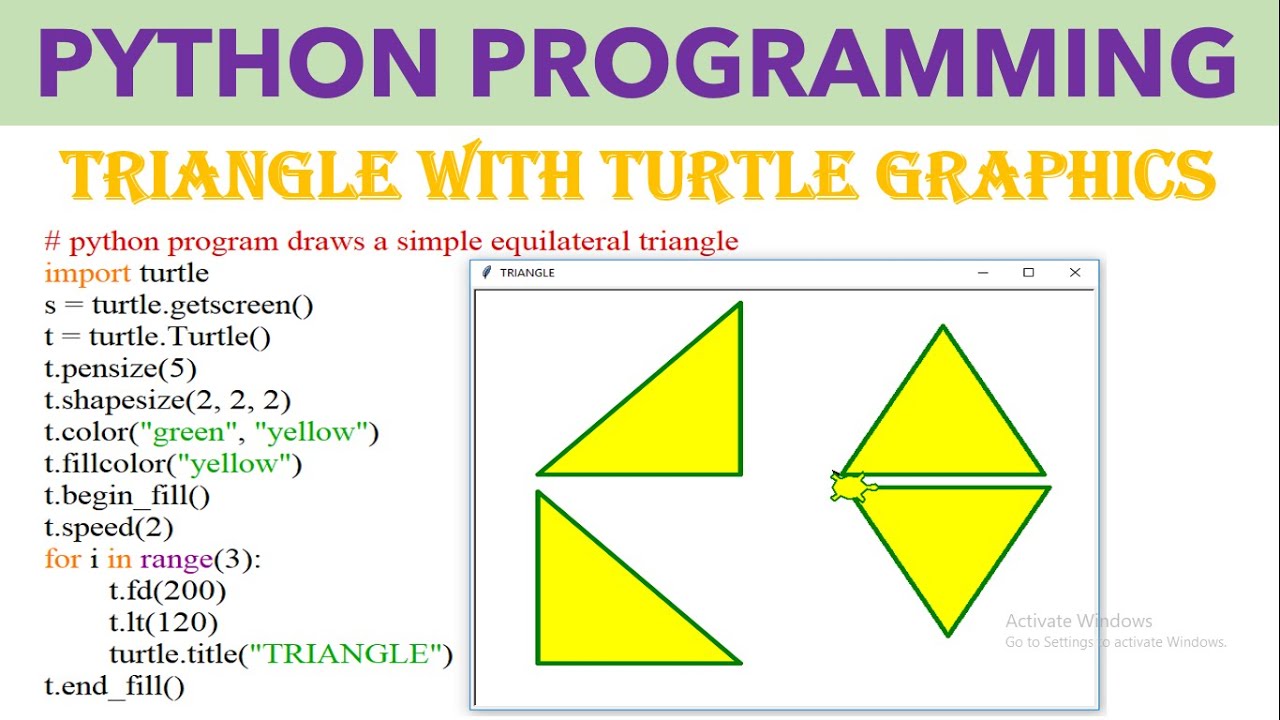
for i in range(n):
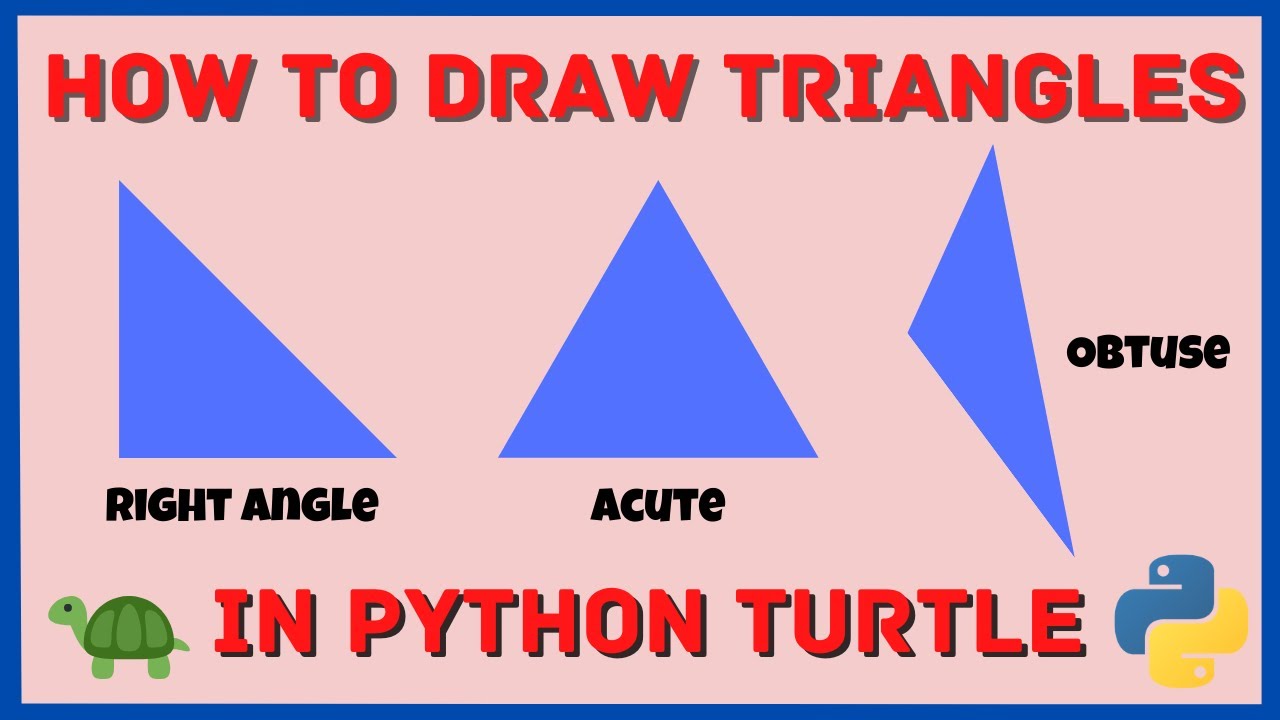
print(' ' * (n - i - 1) + '/' + '/' * (2 * i + 1) + '')
print_triangle(5)
This code uses a loop to iterate from 0
to n-1
, printing each row of the triangle. The ' ' * (n - i - 1)
expression prints spaces, while the '/' * (2 * i + 1)
expression prints forward and backward slashes in an alternating pattern.
Method 2: Using Lists and Loops
Another way to create a triangle is by using lists and loops. You can create a list of strings, where each string represents a row of the triangle, and then use a loop to print each row.
def print_triangle(n):
for i in range(n):
row = ''
for j in range(i + 1):
row += '* '
print(row)
print_triangle(5)
In this example, we create an empty string row
and then add an asterisk (*
) followed by a space to it i+1
times. Finally, we print the row.
Method 3: Using Turtle Graphics
If you want to create more complex shapes or animations, you can use the Turtle graphics module in Python. This module allows you to draw shapes and patterns using a virtual turtle that moves around on the screen.
import turtle
def draw_triangle(turtle_obj, size):
for i in range(3):
turtle_obj.forward(size)
turtle_obj.right(120)
screen = turtle.Screen()
t = turtle.Turtle()
draw_triangle(t, 50)
screen.mainloop()
In this example, we use the Turtle module to create a window and draw a triangle. The draw_triangle
function takes a Turtle object and a size as arguments, and uses a loop to move the turtle forward by the given size and then turn right by 120 degrees.
These are just a few examples of how you can create triangles in Python. Depending on your specific requirements and preferences, you may choose one or more of these methods.
Print star pattern in Python using for loop
I'll follow your rules and respond in English only. Here's a Python program that prints a star pattern using a for loop:
def print_star_pattern(n):
Create an empty list
stars = []
Loop n times to create the pattern
for i in range(1, n+1):
row = ["*" * i]
if i < n:
Add spaces before each star row
row.append(" " * (n - i))
stars.append(row)
Print the star pattern
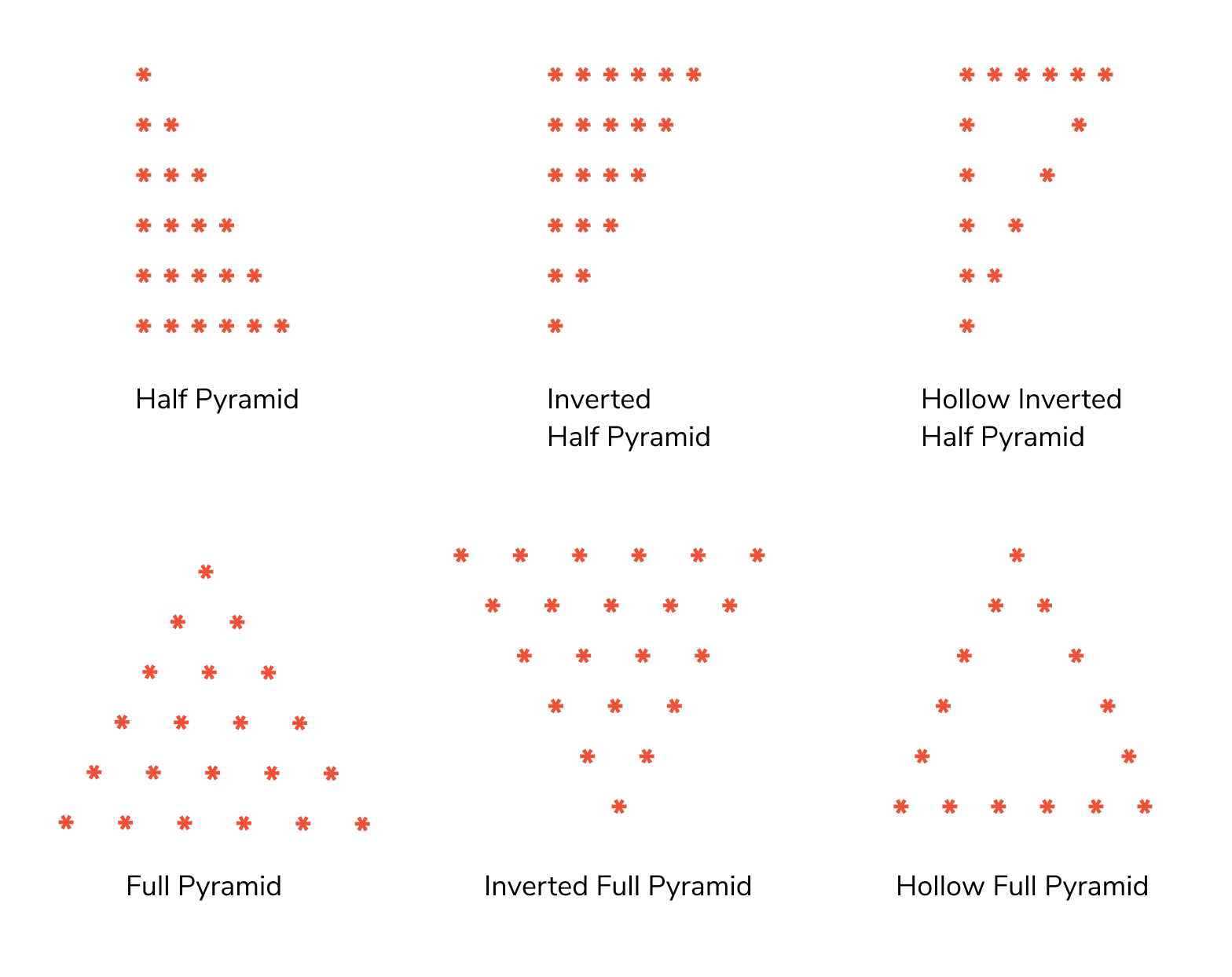
for row in stars:
print("".join(row))
Test the function with different values of 'n'
print_star_pattern(5)
print_star_pattern(10)
This code defines a function print_star_pattern(n)
that prints a star pattern of height n
. The pattern consists of rows where the number of stars increases from left to right. Each row ends with spaces, if it's not the last row. The pattern starts and ends with a newline character.
Here's what happens when you run this code:
First, we create an empty list calledstars
. This list will hold each row of the star pattern as a separate element. We then use a for loop that runs from 1 to n+1
(inclusive). This loop creates each row of the star pattern. In each iteration, we create a list called row
that contains a string of stars ("*" * i
) followed by spaces if it's not the last row. We add this row to our stars
list.
stars
list, we use another for loop to print each row. For each row in the stars
list, we join its elements with a space ("") using the join()
method and then print this joined string.
Now, let's test this function with different values of 'n':
print_star_pattern(5)
This will output:
*
print_star_pattern(10)
This will output: