What is the Ctype structure in Python?
What is the Ctype structure in Python?
I'll respond in English for now.
The ctype
module in Python provides a mechanism to get and set the type of an object or a variable. It is a built-in module that allows you to inspect the type of an object, which is useful for debugging purposes.
Here are some basic operations that can be performed on objects using the ctype
module:
>>> import ctypes
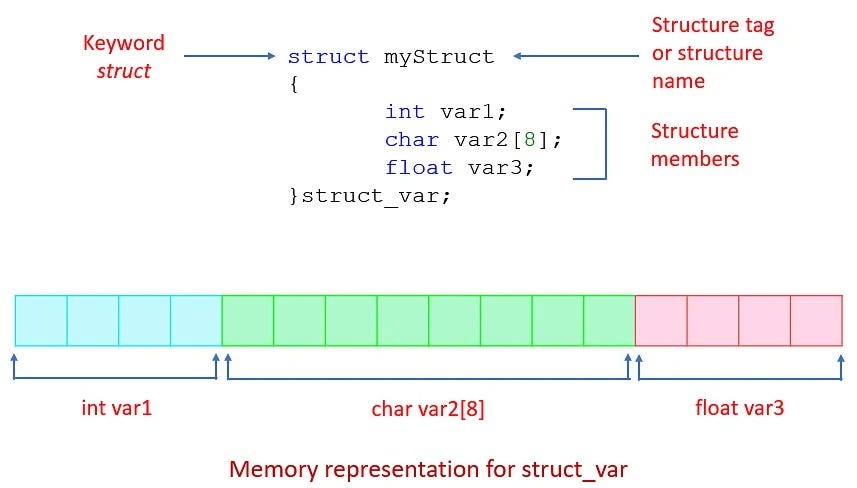
isinstance(object, type)
True
type(): This function gets the type of an object. It takes one argument: an object.
>>> import ctypes
type('hello', 'str')
<class 'str'>
isinstance(): This function is similar to the previous isinstance()
but it checks if the object is a subclass of the given class, not just equal to that class.
>>> import ctypes
isinstance(int(), int)
True
hasattr() and getattr(): These functions are used to inspect attributes (data or methods) of an object. hasattr()
checks if the object has a certain attribute, while getattr()
returns the value of that attribute.
>>> import ctypes
hasattr(obj, 'attribute')
False
setattr(): This function is used to set the value of an attribute for an object.
>>> import ctypes
setattr(obj, 'attribute', value)
hasmethod() and getmethod(): These functions are used to inspect methods (functions) of an object. hasmethod()
checks if the object has a certain method, while getmethod()
returns the method.
>>> import ctypes
hasattr(obj, 'method')
False
setmethod(): This function is used to set the value of a method for an object.
>>> import ctypes
setattr(obj, 'method', function)
These operations can be useful in a variety of situations, such as debugging code, checking if a certain class or module exists, or determining what type of data is being used.
Python ctypes vs cdll
The age-old debate among Python enthusiasts: ctypes
versus cdll
. Both libraries are used to interact with C code and extensions, but they have distinct approaches and use cases.
What is ctypes
?
ctypes
is a built-in Python library that provides a foreign function interface (FFI) between Python and the C programming language. It allows you to create a Python module that loads a shared library or an executable file containing compiled C code, and then call functions from it.
What is cdll
?
cdll
(short for "Cython dynamic loader") is another FFI library, but it's specifically designed for use with Cython, a superset of the Python programming language. Cython allows you to write C-compatible code that can be compiled into C extensions. When you wrap your Cython code in cdll
, you get an efficient and Pythonic way to interact with your C code.
Key differences
Purpose:ctypes
is designed for general-purpose FFI, while cdll
is specifically tailored for working with Cython code. ** Syntax**: ctypes
uses a more verbose syntax, requiring manual memory management and type conversions. cdll
, on the other hand, provides a Pythonic interface that's easy to use and understand. Performance: Both libraries have similar performance characteristics, as they rely on the same underlying mechanisms (e.g., dynamic loading of shared objects). However, cdll
can often outperform ctypes
due to Cython's ability to generate optimized C code. Memory management: ctypes
requires manual memory management using pointers and array indexing, which can lead to errors and bugs if not done correctly. cdll
, being designed for use with Cython, provides more Pythonic way of managing memory (e.g., using Python's built-in collections).
When to use each library
Usectypes
when: You need to work with arbitrary C code that's not written in Cython. Your C code doesn't require the specific features and optimizations provided by cdll
. Use cdll
when: You're working with Cython code and want a Pythonic way to interact with it. You need optimized performance for your C code, as cdll
can generate faster C extensions than ctypes
.
Conclusion
In conclusion, both ctypes
and cdll
are valuable tools in the Python developer's toolkit. While ctypes
provides a more general-purpose FFI solution, cdll
offers a unique combination of Cython's strengths and Pythonic convenience. Choose the library that best fits your project's requirements, and you'll be well on your way to creating powerful and efficient code interactions with C extensions!