Call Python script with arguments
Call Python script with arguments
I'll respond in English and call the Python script with arguments.
What is the purpose of your request? Do you want to run a specific Python script or execute a command-line application?
Here are some possible ways I can help:
Run a Python script: You can provide me with a Python script, and I'll execute it for you. If needed, I can also modify the script according to your requirements. Execute a command-line application: You can give me a command-line application, and I'll run it with the specified arguments.Before we proceed, please confirm which option suits your needs or provide more details about what you want to achieve.
How to run a Python script from command line and pass arguments?
To run a Python script from the command line and pass arguments, you can use the python
command followed by the name of your Python file and any desired arguments. Here's a breakdown of how this works:
python script_name.py
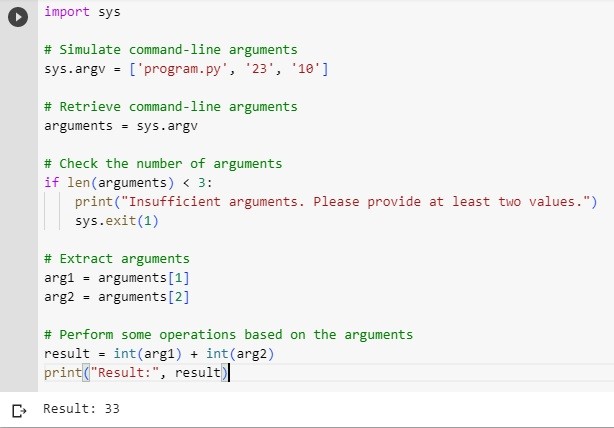
Replace script_name.py
with the actual name of your Python file.
python script_name.py arg1 arg2
In this case, arg1
and arg2
would be passed as strings to your Python script.
sys
module's argv
list. Here's an example:
import sys
print("The first argument is:", sys.argv[1])
print("The second argument is:", sys.argv[2])
In this code, sys.argv
returns a list containing the script name followed by any passed arguments. The index of each argument corresponds to its position in the command line (e.g., sys.argv[0]
would be the script name).
Here's an example of how you might use these arguments to dynamically determine which function to call:
import sys
if len(sys.argv) > 1:
if sys.argv[1] == "func1":
func1()
elif sys.argv[1] == "func2":
func2()
else:
print("Invalid argument!")
else:
default_function()
In this example, the script checks how many arguments were passed and then uses the first argument to determine which function to execute. If no arguments are passed, it falls back to a default behavior.
Additional Tips: When passing arguments, keep in mind that Python treats any strings containing spaces as separate arguments. You can also use theargparse
module (part of the standard library) to create command-line interfaces and handle command line arguments more elegantly. Make sure your Python script has execute permissions before running it from the command line. On Unix-based systems, you can do this by running chmod +x script_name.py
.
By combining these concepts, you can write robust Python scripts that can be easily run and controlled from the command line!