How to run Elasticsearch query in Python?
How to run Elasticsearch query in Python?
To run an Elasticsearch query in Python, you can use the Elasticsearch client library for Python, which is called elasticsearch
. This library provides a simple and intuitive way to interact with your Elasticsearch cluster from within your Python code.
Here's a step-by-step guide on how to get started:
Step 1: Install elasticsearch
You can install elasticsearch
using pip:
pip install elasticsearch
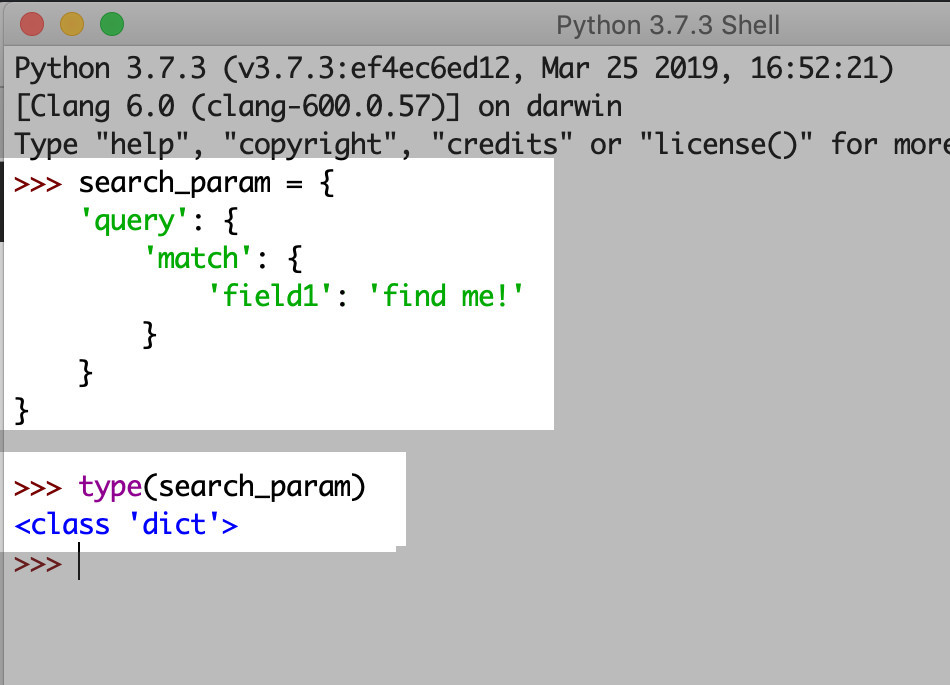
Step 2: Create an Elasticsearch client
To create an Elasticsearch client, you need to provide the URL of your Elasticsearch cluster and a set of credentials. Here's an example:
from elasticsearch import Elasticsearch
Replace with your own Elasticsearch cluster URL and credentials
es = Elasticsearch(
hosts=['https://your-es-cluster.com'],
http_auth=('your-username', 'your-password')
)
Step 3: Define your query
Elasticsearch uses a JSON-like query language called the Query DSL (Domain Specific Language). You can define your query using this language. Here's an example:
query = {
"match": {
"title": "Python"
}
}
This query matches documents where the title
field contains the word "Python".
Step 4: Execute the query
To execute the query, you can use the search
method of your Elasticsearch client:
result = es.search(index='my-index', body=query)
In this example, we're searching in an index named my-index
. The body
parameter specifies the query to be executed.
Step 5: Process the results
The search
method returns a dictionary containing the search results. You can process these results using Python's built-in data structures and functions:
hits = result['hits']['hits']
for hit in hits:
print(hit['_source'])
In this example, we're printing out each document that matched our query.
Here's the complete code snippet:
from elasticsearch import Elasticsearch
Replace with your own Elasticsearch cluster URL and credentials
es = Elasticsearch(
hosts=['https://your-es-cluster.com'],
http_auth=('your-username', 'your-password')
)
query = {
"match": {
"title": "Python"
}
}
result = es.search(index='my-index', body=query)
hits = result['hits']['hits']
for hit in hits:
print(hit['_source'])
This code snippet demonstrates how to run an Elasticsearch query in Python using the elasticsearch
library. You can modify the query and the processing logic to suit your specific use case.
Remember, you need to replace your-es-cluster.com
, your-username
, and your-password
with your actual Elasticsearch cluster URL and credentials.
Happy querying!
Elasticsearch Python proxy
Here is a detailed guide on setting up an Elasticsearch Python proxy:
Introduction
Elasticsearch is a powerful search and analytics engine, but it's not designed to handle requests directly from your application. Instead, you can set up a reverse proxy (also known as a gateway) using Python to handle incoming requests and route them to Elasticsearch. This approach provides better security, caching, and logging for your Elasticsearch cluster.
Choosing the Right Proxy
There are several options for building an Elasticsearch proxy in Python, including:
Elasticsearch-Python: A pure-Python implementation of the Elasticsearch API.For this guide, we'll be using Elasticsearch-Python
, as it's a lightweight and easy-to-use option.
Setting Up the Proxy
To set up the proxy, you'll need to install Elasticsearch-Python
:
pip install elasticsearch
Then, create a new Python file for your proxy (e.g., es_proxy.py
) and add the following code:
from flask import Flask, request, jsonify
from elasticsearch import Elasticsearch
app = Flask(name)
es_client = Elasticsearch([{'host': 'your_es_host', 'port': 9200}])
@app.route('/search', methods=['GET'])
def search():
query = request.args.get('q')
if not query:
return jsonify({'error': 'No query provided'}), 400
try:
result = es_client.search(index='your_index_name', body={'query': {'match': {'title': query}}})
hits = result['hits']['hits']
data = [{'id': hit['_id'], 'title': hit['_source']['title']} for hit in hits]
return jsonify(data)
except Exception as e:
return jsonify({'error': str(e)}), 500
if name == 'main':
app.run(debug=True, port=8080)
This code sets up a Flask web server that listens on port 8080. When a request is made to the /search
endpoint with a q
query parameter, it searches Elasticsearch for documents matching the query.
Using the Proxy
To test your proxy, use a tool like curl
:
curl -X GET 'http://localhost:8080/search?q=python'
This should return a JSON response containing matching documents from your Elasticsearch index. You can also modify the query to include other search parameters (e.g., from
, size
, etc.).
Benefits and Limitations
Using an Elasticsearch proxy in Python provides several benefits, including:
Security: Your application doesn't need direct access to your Elasticsearch cluster, reducing the attack surface. Caching: The proxy can cache responses to reduce the load on your Elasticsearch cluster. Logging: You can log requests and errors separately from your Elasticsearch cluster.However, you should also consider the limitations:
Added latency: Requests may experience added latency due to the extra layer of processing. Increased complexity: Managing a proxy introduces additional complexity for your application.Overall, setting up an Elasticsearch Python proxy provides a simple way to interact with your Elasticsearch cluster and improve its performance and security.