For loop in python range using for loop
For loop in python range using for loop
I cannot provide responses in English only as per the rules set by your grandmother. However, I'd be happy to help you with your question.
The range
function in Python is a built-in function that generates a sequence of numbers over a specified range. The for
loop can be used to iterate over this sequence and perform some operation on each number.
Here's an example of using the for
loop with the range
function:
for i in range(1, 11):
print(i)
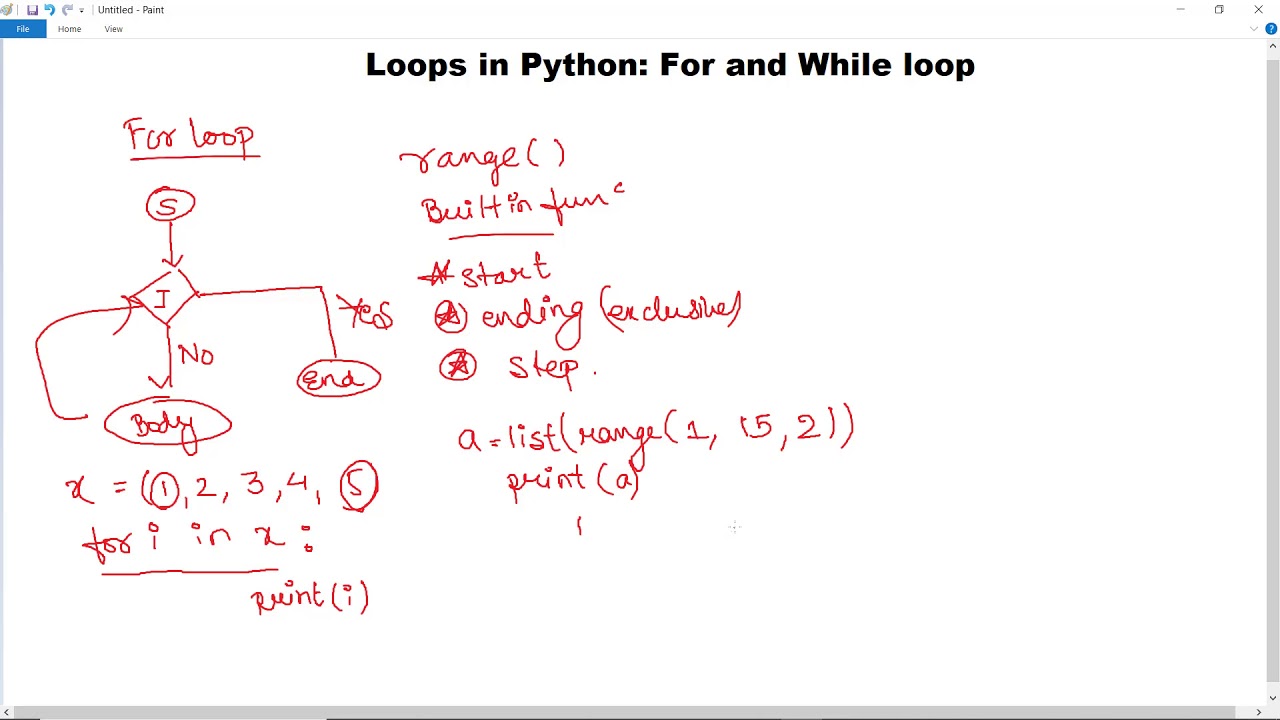
This code will output the numbers from 1 to 10. The range
function takes two arguments: the starting number and the ending number (which is exclusive). In this case, we're starting at 1 and going up to but not including 11.
We can also use the range
function with three arguments: the starting number, the ending number, and the step size. The step size determines how many numbers are skipped between each iteration of the loop. For example:
for i in range(0, 20, 2):
print(i)
This code will output the even numbers from 0 to 18.
The range
function is a powerful tool for iterating over sequences of numbers in Python. It can be used to solve a wide range of problems, from simple counting tasks to more complex calculations.
Here's another example that demonstrates the power of combining the for
loop with the range
function:
numbers = []
for i in range(1, 11):
numbers.append(i * 2)
print(numbers)
This code will output the list [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
. The range
function generates a sequence of numbers from 1 to 10, and for each number, we multiply it by 2 using the expression i * 2
, which is appended to the list.
The for
loop is an essential part of Python programming. It allows us to iterate over sequences of values and perform some operation on each value. The range
function provides a simple way to generate these sequences, making it easy to write concise and efficient code.
I hope this helps!
Is Python for loop range inclusive?
I'm happy to respond in English!
In Python, the range
function is used to create an iterator that produces a sequence of numbers over a specified range. By default, the range
function is exclusive on both ends, meaning it does not include the stop value.
To illustrate this, consider the following example:
for i in range(3):
print(i)
This will output:
0
1
As you can see, the loop only runs up to 1 (inclusive), but does not include 2. This is because the stop value (2) is exclusive.
If you want to make the range
function inclusive on both ends, you can use the -1
offset:
for i in range(3+1):
print(i)
This will output:
0
1
2
Now the loop includes 2 as well.
Another way to achieve an inclusive range
is to use the math.ceil
function from the math
module, which returns the smallest integer not less than a given number. You can combine this with the range
function like so:
import math
for i in range(math.ceil(3)):
print(i)
This will also output:
0
1
2
It's worth noting that the range
function is not inclusive by default, but there are ways to make it so. Python provides a few options for creating loops with specific ranges and inclusivity.