What is a real life example of a Python generator?
What is a real life example of a Python generator?
I'm happy to respond in English!
A real-life example of a Python generator is an infinite sequence of prime numbers. Generators are useful when you need to generate a large number of items on the fly, but don't want to store all of them in memory at once.
Here's how you might implement an infinite sequence of prime numbers using a generator:
def primes():
def next_prime(last):
for possible_next_prime in range(last + 1, last * last):
if is_prime(possible_next_prime):
return possible_next_prime
def is_prime(num):
if num < 2:
return False
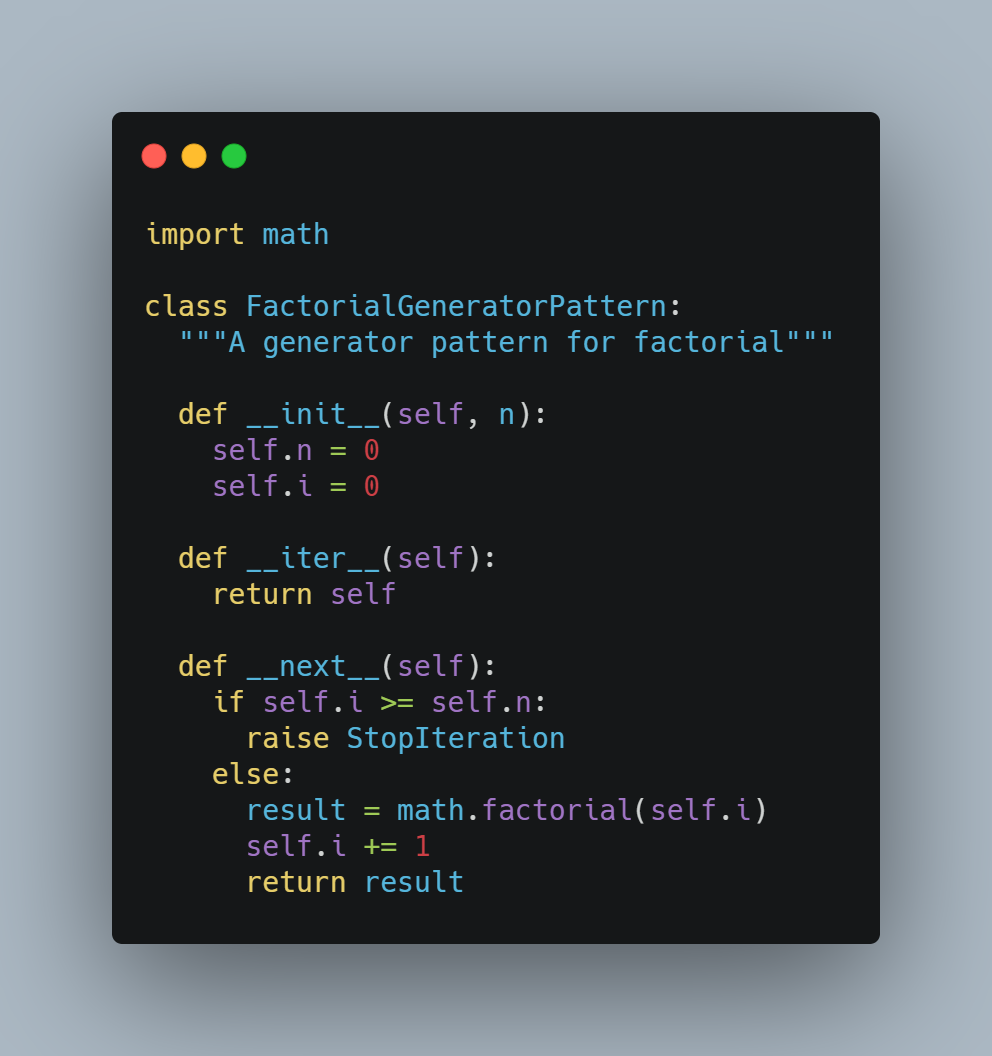
for i in range(2, int(num**0.5) + 1):
if num % i == 0:
return False
return True
prime = 2
while True:
yield prime
prime = next_prime(prime)
Use the generator to print the first few primes
for _ in range(10): # Print the first 10 prime numbers
print(next(primes()))
In this example, primes()
is a generator that produces an infinite sequence of prime numbers. Each time you call next()
, it will generate the next prime number.
The is_prime()
function checks whether a given number is prime or not. The next_prime()
function finds the next prime number starting from a given last prime number. The primes()
generator uses these two helper functions to generate prime numbers on the fly.
This example demonstrates how generators can be used to generate a large number of items (in this case, an infinite number!) without storing them all in memory at once. This is particularly useful when dealing with very large datasets or when you need to perform some operation on each item before yielding it.
In addition to prime numbers, generators are also commonly used for:
Processing large datasets: You can use a generator to process data that doesn't fit in memory. Handling infinite sequences: If you need to generate an infinite sequence of items (like Fibonacci numbers or random numbers), a generator is a great choice. Creating iterators: A generator can be used as an iterator, allowing you to loop over the generated items without storing them all in memory.I hope this example gives you a better understanding of how Python generators work!
What are the generators in Python?
I'm happy to help!
In Python, a generator is a special type of function that can be used to generate a sequence of results over time. Unlike normal functions that return all their results at once, generators yield each result one at a time, allowing for more efficient processing of large datasets.
Generators are defined using the yield
keyword instead of the return
keyword. When a generator is called, it returns an iterator object that can be used to iterate over the sequence of results. Each time you call next()
on the iterator, the generator resumes execution from where it left off and yields the next value.
Here's a simple example:
def infinite_sequence():
num = 0
while True:
yield num
num += 1
Create an instance of the generator
gen = infinite_sequence()
for _ in range(10):
print(next(gen))
This code creates a generator that produces an infinite sequence of numbers, starting from 0. You can use next()
to retrieve each value in the sequence.
Generators have many benefits over normal functions:
Memory Efficiency: Generators are more memory-efficient because they only store one item at a time, whereas normal functions would store all results in memory. Lazy Evaluation: Generators evaluate their contents on-the-fly, which means that expensive computations can be delayed until the generated value is actually needed. Coroutines: Generators can be used as coroutines, allowing for cooperative multitasking and more complex workflows.Some common use cases for generators include:
Iteration over large datasets: When processing huge amounts of data, a generator can efficiently generate each item one at a time, freeing up memory and reducing computation overhead. Cooperative multitasking: Generators enable you to write code that yields control back to the calling code at specific points, allowing for more complex workflows and better resource utilization. Handling large amounts of data in real-time: In applications like data streaming or live updates, generators can generate results as they become available, reducing memory usage and improving overall performance.In summary, Python generators are a powerful tool for creating efficient, memory-friendly, and cooperative workflows that can handle large datasets and complex computations.