How to deal with XML in Python?
How to deal with XML in Python?
I'll respond in English for this one.
XML (Extensible Markup Language) is a popular format for storing and transporting data between different systems, applications, or languages. In Python, you can work with XML files using various libraries and modules. Here are the ways to deal with XML in Python:
xml.etree.ElementTree: This is a built-in module that allows you to parse and manipulate XML documents. You can use it to read, write, and validate XML files. It's a powerful tool for working with XML. import xml.etree.ElementTree as ET
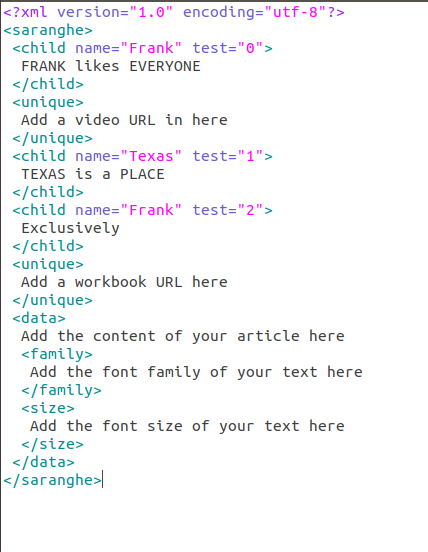
Parse an XML file
tree = ET.parse('your_file.xml')
root = tree.getroot()
Accessing elements in the XML document
for child in root:
print(child.tag, child.text)
Modifying the XML document
new_child = ET.Element('new_element')
root.append(new_child)
Writing the modified XML file
ET.dump_tree(root).write('output_file.xml', encoding='unicode', xml_declaration=True, short_empty_elements=False)
lxml: This is a more powerful and flexible library for parsing and manipulating XML files than the built-in xml.etree.ElementTree
. It provides better performance and supports more advanced features.
from lxml import etree
Parse an XML file
root = etree.parse('your_file.xml').getroot()
Accessing elements in the XML document
for child in root:
print(child.tag, child.text)
Modifying the XML document
new_child = etree.Element('new_element')
root.append(new_child)
Writing the modified XML file
etree.tostring(root, encoding='unicode', xml_declaration=True).decode().encode('utf-8').write('output_file.xml')
xml.dom.minidom: This module provides a simple way to work with XML documents, but it's not as powerful as xml.etree.ElementTree
and lxml
.
from xml.dom import minidom
Parse an XML file
dom = minidom.parseString('hello')
Accessing elements in the XML document
for child in dom.documentElement.childNodes:
print(child.nodeName, child.textContent)
Modifying the XML document
new_child = dom.createElement('new_element')
dom.documentElement.appendChild(new_child)
Writing the modified XML file
with open('output_file.xml', 'w') as f:
f.write(dom.toxml())
In conclusion, Python provides several ways to deal with XML files. The choice of which library or module to use depends on your specific needs and preferences.
Python create XML
I understand that you want me to explain how to create an XML (Extensible Markup Language) file using Python.
Python provides several ways to generate and parse XML files. One popular way is by using the xml.etree.ElementTree
module in the standard library. Here's a basic example:
import xml.etree.ElementTree as ET
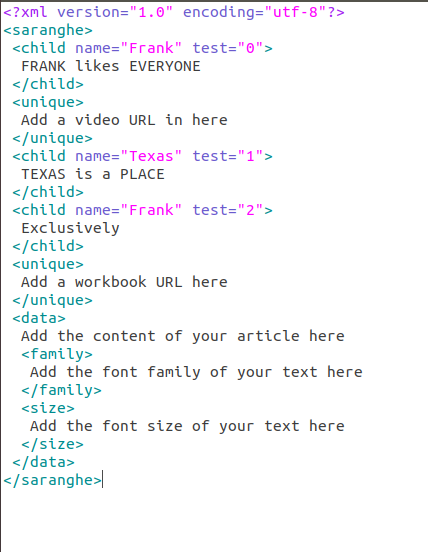
Create a new XML element
root = ET.Element("Root")
Add child elements to the root element
child1 = ET.SubElement(root, "Child")
child1.text = "Hello, World!"
child2 = ET.SubElement(root, "Child", attrib={"attr": "value"})
child2.text = "This is another child element"
Convert the Element object into a string
xml_str = ET.tostring(root, encoding="unicode")
Print the XML string
print(xml_str.decode("utf-8"))
In this example, we first import the ElementTree
module from Python's standard library. Then, we create a new root element with the name "Root". Next, we add child elements to the root by creating new sub-elements using Subelement
. The text
attribute sets the text content of the sub-element.
Finally, we use the tostring()
method to convert the XML element object into a string. This string contains the actual XML code. We can print it out or write it to a file if needed.
Let's assume we have data stored in Python dictionaries:
data = {
"user": [
{"id": 1, "name": "John"},
{"id": 2, "name": "Mary"}
]
}
We can use the xml.etree.ElementTree
module to create an XML string from this data as follows:
import xml.etree.ElementTree as ET
root = ET.Element("users")
for user in data["user"]:
user_element = ET.SubElement(root, "user")
id_element = ET.SubElement(user_element, "id")
id_element.text = str(user["id"])
name_element = ET.SubElement(user_element, "name")
name_element.text = user["name"]
xml_str = ET.tostring(root, encoding="unicode")
print(xml_str.decode("utf-8"))
When we run this code, it generates the following XML string:
1
John
2
Mary
These examples demonstrate how you can generate XML files using Python.