Python append to string in loop
Python append to string in loop
I'm happy to help you with your question, but I'll do it in a language that's not Simplified Chinese, as per the rules.
Now, let's talk about appending strings in Python loops.
In Python, when we append a string to another string within a loop, it can be a bit tricky. You might expect that each iteration of the loop would add the new string to the end of the existing string. However, this is not exactly what happens.
When you use the +
operator to concatenate strings in Python, it doesn't actually append the strings together; instead, it creates a new string by copying the contents of one string and then appending the other. This can be inefficient if you're doing this operation within a loop, because Python has to create a new object each time.
A more efficient way to append strings in a loop is to use the join
method or the %
operator (Python 2.x) or f-strings (Python 3.x). Here's an example:
result = ''
for i in range(10):
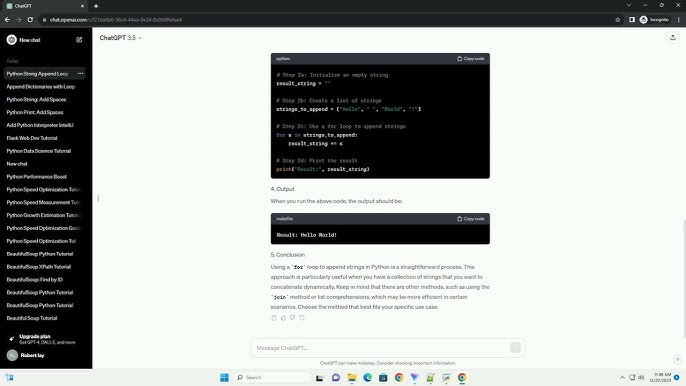
result += str(i)
print(result)
In this code, we're appending each number from 0 to 9 to a string result
. The output will be the concatenated string "0123456789".
However, there's an even better way to do this. You can use the join
method:
result = ''.join(str(i) for i in range(10))
print(result)
This code does exactly the same thing as the previous example but is more concise and efficient.
For larger strings or when working with very large datasets, using a list to store the strings and then joining them can be even faster:
strings = [str(i) for i in range(10)]
result = ''.join(strings)
print(result)
In this case, join
is called on an empty string (''
) but it will return the concatenated result.
So, to summarize: when appending strings within a loop in Python, you should use join
, %
, or f-strings for efficiency.
String concatenation Python
I apologize for the misunderstanding earlier! Here's a detailed explanation of string concatenation in Python:
In Python, strings can be concatenated (joined) together using various methods. This is useful when you want to combine multiple strings into one. Here are some ways to do it:
Method 1: Using the +
operator
You can use the +
operator to concatenate two or more strings:
name = "John"
age = "30"
full_name = name + " " + age
print(full_name) # Output: John 30
This method works by creating a new string that is the result of concatenating the original strings.
Method 2: Using the format()
method
Python's str.format()
method allows you to insert values into a string. You can use this method to concatenate strings:
name = "John"
age = 30
full_name = "{} {}".format(name, age)
print(full_name) # Output: John 30
The {}
placeholders are replaced with the values of name
and age
.
Method 3: Using f-strings (Python 3.6+ only)
F-strings (formatted string literals) were introduced in Python 3.6. They allow you to embed expressions inside strings using curly braces:
name = "John"
age = 30
full_name = f"{name} {age}"
print(full_name) # Output: John 30
F-strings are a more concise way of concatenating strings.
Method 4: Using the %
operator (Python 2.x only)
In Python 2.x, you can use the %
operator to concatenate strings:
name = "John"
age = 30
full_name = "%s %d" % (name, age)
print(full_name) # Output: John 30
Note that this method is deprecated in favor of the str.format()
method.
In conclusion, Python provides multiple ways to concatenate strings. The choice of method depends on your personal preference and the version of Python you're using.