Python with open as outfile
Python with open as outfile
I'm happy to respond in English for you!
Regarding your request about using the open
function in Python to write output to a file, I'd be delighted to provide some information.
In Python, the open
function is used to open a file and prepare it for reading or writing. When opening a file for writing, you can specify the mode as 'w'
(write) or 'a'
(append), which allows you to create a new file or add content to an existing one.
Here's an example of how to use open
as an outfile:
with open('output.txt', 'w') as outfile:
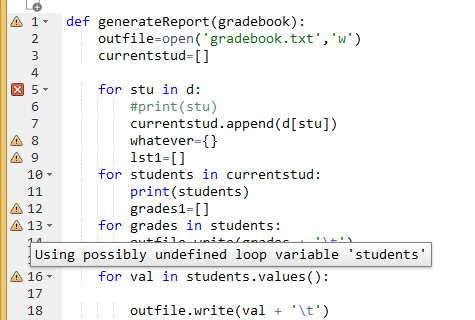
outfile.write('Hello, world!') # write string to file
outfile.write('This is a test file.n') # write string to file with newline character
In this example, we're opening a new file called output.txt
in write mode ('w'
). The with
statement ensures that the file is properly closed after we're done writing to it. Inside the block, we use the write
method to write two strings to the file: 'Hello, world!'
and 'This is a test file.n'
. The newline character (n
) is added at the end of the second string.
When you run this code, Python will create a new file called output.txt
in the current working directory (unless it already exists), and write the two strings to it.
If you want to append content to an existing file instead, simply change the mode from 'w'
to 'a'
:
with open('existing_file.txt', 'a') as outfile:
outfile.write('Additional text added at the end.n')
In this case, we're appending new content to an existing file called existing_file.txt
. The write
method still works the same way.
Some important notes:
Make sure you specify a valid file path and name when opening the file. Use the correct mode ('w'
for writing or 'a'
for appending). If you're working with binary files (e.g., images, audio), you'll need to use the 'wb'
or 'ab'
modes instead of 'w'
or 'a'
. Always close the file using the close()
method when finished writing to avoid any potential issues.
I hope this helps! Let me know if you have further questions.
What does open () do Python?
I'd be happy to help!
In Python, the open()
function is used to open and read or write a file. It's one of the most fundamental functions for working with files in Python.
The syntax of the open()
function is as follows:
file_object = open(filename, mode)
Here:
filename
is the name of the file you want to open. mode
is a string that specifies how you want to interact with the file. The most common modes are: 'r'
: Read-only mode. This is the default mode if you don't specify a mode. 'w'
: Write-only mode. If the file already exists, its contents will be overwritten. 'a'
: Append mode. New content will be added to the end of the existing file. 'r+'
: Read and write mode (read-only at the beginning). 'w+'
: Read and write mode (write-only at the beginning), truncating the file first. 'a+'
: Read and append mode, starting at the end.
When you open a file with open()
, it returns a file object. This object has several methods that allow you to interact with the file:
read()
: Read the contents of the file. write()
: Write data to the file. seek()
: Move the read/write position in the file. tell()
: Get the current read/write position.
For example, let's open a file called example.txt
and write some text:
with open('example.txt', 'w') as f:
f.write('Hello, world!')
This code opens example.txt
in write mode, writes 'Hello, world!'
, and then closes the file.
If you want to read from a file, you can use the read()
method:
with open('example.txt', 'r') as f:
content = f.read()
print(content) # Output: Hello, world!
In this example, we open example.txt
in read mode, read its contents using f.read()
, and then print the result.
Remember to always close your files when you're done with them! Python has a built-in with
statement that can help you do this automatically. For example:
with open('example.txt', 'w') as f:
f.write('Hello, world!')
The file will be closed automatically when the with
block is exited, whether an exception occurred or not.
I hope this helps!