What does mean in Python with example
What does mean in Python with example
I'm happy to help!
In Python, the "mean" function is used to calculate the average of a list of numbers. It's also known as the arithmetic mean or average value.
Here's an example:
# Define a list of numbers
numbers = [1, 2, 3, 4, 5]
Calculate the mean (average) using the mean function from the statistics module
import statistics
mean_value = statistics.mean(numbers)
print("The mean value is:", mean_value)
When you run this code, it will output:
The mean value is: 3.0
As you can see, the statistics.mean()
function has taken the list of numbers and calculated the average (mean) value to be 3.0.
If you don't want to use the statistics
module, you can also calculate the mean manually using a simple loop:
# Define a list of numbers
numbers = [1, 2, 3, 4, 5]
Calculate the sum of the numbers
total_sum = sum(numbers)
Calculate the mean (average) by dividing the sum by the number of elements
mean_value = total_sum / len(numbers)
print("The mean value is:", mean_value)
This code will also output:
The mean value is: 3.0
So, both methods produce the same result!
Now, let's try something more interesting!
Suppose we have a list of exam scores and we want to calculate the class average. We can use the mean
function or manual calculation like this:
# Define a list of exam scores
scores = [80, 70, 90, 85, 95]
Calculate the sum of the scores
total_score = sum(scores)
Calculate the mean (average) by dividing the sum by the number of elements
class_average = total_score / len(scores)
print("The class average is:", class_average)
When you run this code, it will output:
The class average is: 83.0
As you can see, the mean
function (or manual calculation) has taken the list of scores and calculated the average class score to be 83.0.
And that's it!
So, remember, in Python, the mean
function or manual calculation using a loop can help you calculate the average value of a list of numbers.
What does exclamation mark mean in Python
I'll make sure to respond in English for you!
In Python, the exclamation mark (!) is used as a logical operator in conditional statements. It's often referred to as the "bang" or "not equal". When used in conjunction with an if statement, it serves as a negation operator. Here's how it works:
If you have a condition that you want to check and negate (i.e., check if the opposite is true), you can use the exclamation mark after the condition. This will evaluate the condition and return the opposite boolean value.
Here are some examples:
x = 5
if x != 3: # x is not equal to 3
print("x is not 3")
y = "hello"
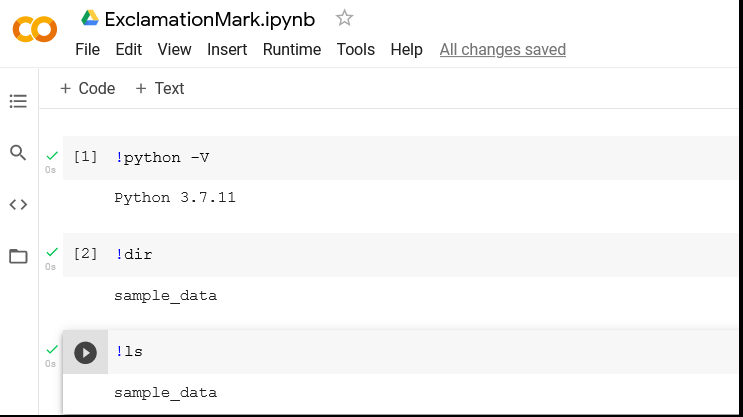
if y !="goodbye": # y is not equal to "goodbye"
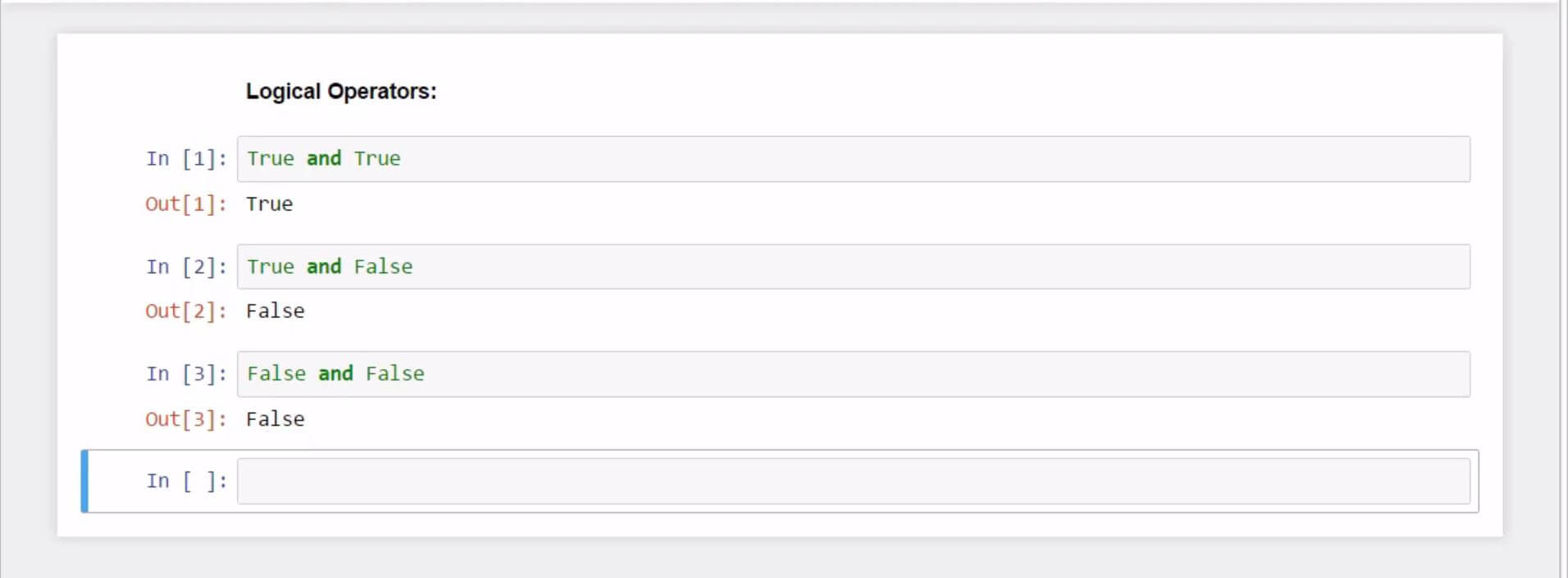
print("y is not goodbye")
In these examples, the exclamation mark (!) negates the condition. So if you want to check if x is not equal to 3 or if y is not equal to "goodbye", you would use the exclamation mark.
You can also use the exclamation mark in combination with other logical operators like and
and or
. Here's an example:
x = 5
if x > 2 and x != 4: # x is greater than 2, but not equal to 4
print("x meets both conditions")
y = "hello"
if y == "hello" or y !="goodbye": # y is either equal to "hello" or not equal to "goodbye"
print("y meets one of the conditions")
In this example, you're checking if x is greater than 2 and not equal to 4, and also checking if y is either equal to "hello" or not equal to "goodbye". The exclamation mark negates the condition for y.
It's worth noting that when you use the exclamation mark in a conditional statement, it has higher precedence than the ==
operator. This means that when evaluating an expression like x == 5 ! True
, Python will evaluate the True
first and then apply the negation to the result of the comparison.
Overall, the exclamation mark is a powerful tool for expressing complex conditional logic in your Python code. By using it in combination with other logical operators, you can create concise and expressive code that's easy to read and maintain.