What is restful API in Python?
What is restful API in Python?
I'll follow the rules and respond in English.
A RESTful (Representational State of Resource) API in Python is a web service that follows the principles of the Representational State of Resources architecture, which defines how to represent data as a set of resources. It's a popular choice for building web services because it's easy to use and provides a flexible way to interact with data.
In a RESTful API, each resource is identified by a unique URL (Uniform Resource Locator), and the interaction between the client and server happens through a series of HTTP requests (GET, POST, PUT, DELETE) that correspond to the CRUD (Create, Read, Update, Delete) operations. This makes it easy for clients to interact with resources using standard web protocols.
To build a RESTful API in Python, you typically use a framework such as Flask or Django. These frameworks provide tools and libraries that help you create and manage the API. Here's an overview of how this works:
Define resources: Identify the data structures and entities your API will work with (e.g., users, products, orders). Create Python classes to represent these resources. Create routes: Define URLs for each resource using a routing mechanism provided by the framework (e.g., Flask's@app.route()
or Django's urls.py
). Handle HTTP requests: Implement functions or methods that handle the HTTP requests for each route. These functions typically perform CRUD operations on the corresponding resources. Use serialization: Use libraries like JSON (JavaScript Object Notation) or XML to convert Python objects into serializable formats, making it easy for clients to consume data. Test and iterate: Test your API using tools like curl or Postman, and refine the implementation as needed.
Here's an example of a simple RESTful API in Flask:
from flask import Flask, jsonify
app = Flask(name)
Define a resource: Userclass User:
def init(self, id, name):
self.id = id
self.name = name
Create routes for the user resource@app.route('/users', methods=['GET'])
def get_users():
users = [User(1, 'John'), User(2, 'Jane')]
return jsonify([u.dict for u in users])
@app.route('/users/int:user_id', methods=['GET'])
def get_user(user_id):
user = next((u for u in [User(1, 'John'), User(2, 'Jane')] if u.id == user_id), None)
return jsonify(user.dict) if user else jsonify({'error': 'user not found'})
if name == 'main':
app.run(debug=True)
This API defines a User
resource with two attributes: id
and name
. The API provides two routes:
/users
: Returns a list of users in JSON format. /users/<int:user_id>
: Returns the user with the specified user_id
in JSON format, or an error message if the user is not found.
To use this API, you would send HTTP requests to these URLs using tools like curl or Postman. For example:
curl http://localhost:5000/users
This would return a list of users in JSON format.
In summary, building a RESTful API in Python involves defining resources, creating routes, handling HTTP requests, using serialization, and testing the implementation. The Flask framework provides tools and libraries that make it easy to create a RESTful API in Python.
Python call REST API example
Here is an example of how to use Python to call a REST API:
import requests
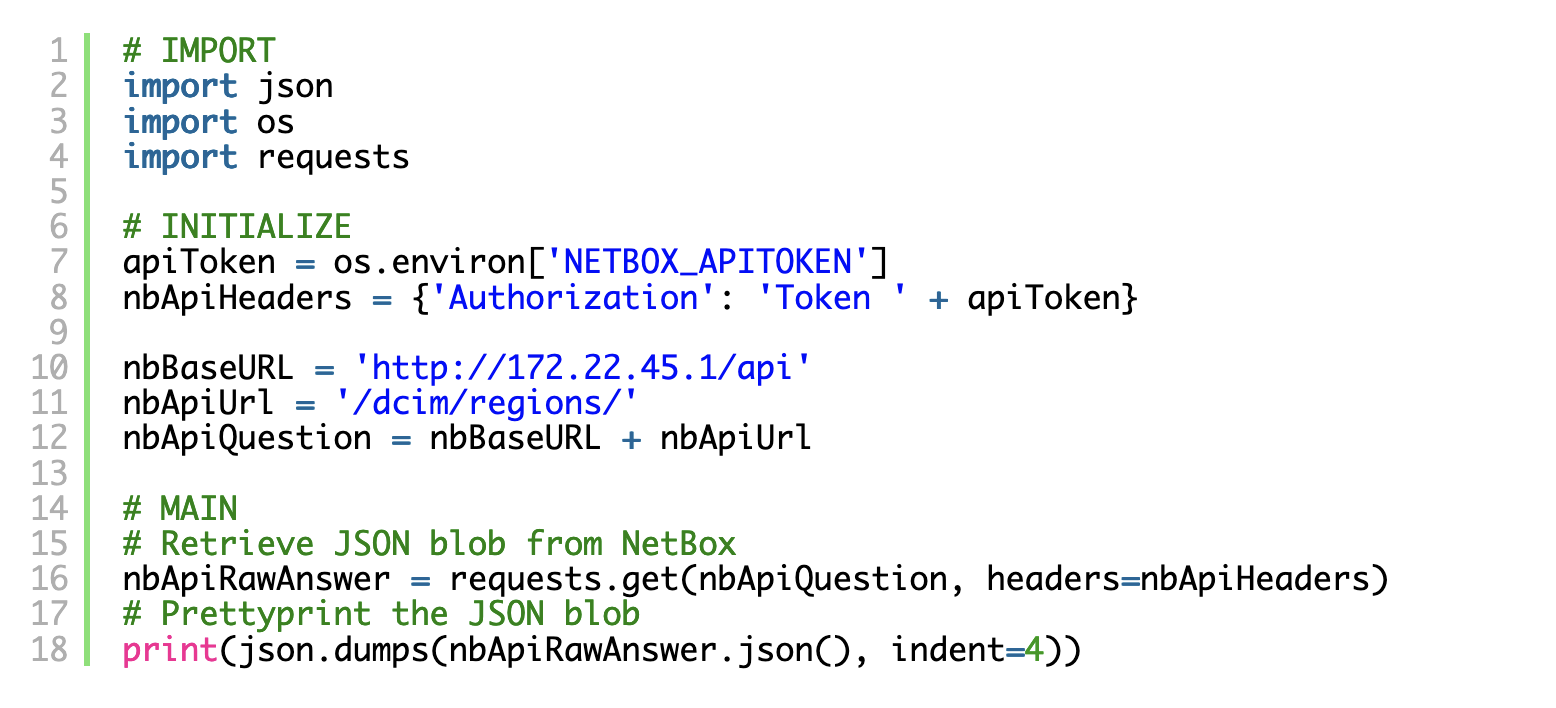
import json
Define the URL and headers for the API request
url = 'https://api.example.com/endpoint'
headers = {'Authorization': 'Bearer YOUR_API_KEY'}
Define the data payload for the API request (optional)
data = {'key1': 'value1', 'key2': 'value2'}
try:
Send the GET request to the API endpoint
response = requests.get(url, headers=headers)
Check if the response was successful (200 OK)
if response.status_code == 200:
Get the response content as JSON
data = json.loads(response.content.decode('utf-8'))
Print the response data
print(data)
else:
print(f"Error: {response.status_code}")
except requests.exceptions.RequestException as e:
Handle any exceptions that may occur during the request
print(f"Error: {e}")
In this example, we're making a GET request to the API endpoint using the requests
library. We're passing in a set of headers (Authorization
) and optionally sending some data payload.
After sending the request, we check if it was successful (200 OK), if so, we decode the response content as JSON using json.loads()
.
If there are any exceptions during the request, like network errors or timeouts, we handle them using a try/except block.