What is the use of for in Python?
What is the use of for in Python?
I'm happy to help you with that!
The keyword "for" in Python is used to iterate over a sequence (such as a list, tuple, or string) or other iterable objects. It's one of the most fundamental control structures in Python, and it plays a crucial role in looping and processing data.
When you use "for" in a loop, you're telling Python to execute a block of code for each item in the sequence or iterable. The general syntax is:
for variable in sequence: do something with variable
Here's a breakdown of how it works:
Initialization: Python assigns the first item from the sequence to the variable. Condition: Python checks if there are more items in the sequence. If so, it continues to the next step. Body: The code inside the loop is executed with the current value of the variable. Iteration: Steps 1-3 are repeated until all items in the sequence have been processed.Some key points to note:
You can use "for" loops with various data structures, such as lists, tuples, strings, dictionaries, and sets. The loop will iterate over each item in the sequence exactly once. If you want to repeat something multiple times, you might need to use a while loop or recursion. You can modify the variable inside the loop (although it's generally a good idea to avoid doing so, as it can make the code harder to read and debug).Here are some examples to demonstrate the power of "for" loops in Python:
# Iterate over a list of numbers
numbers = [1, 2, 3, 4, 5]
total = 0
for num in numbers:
total += num
print(total) # Output: 15
Process each character in a stringtext = "Hello World!"
for char in text:
print(char)
Loop over a dictionary's key-value pairsperson = {"name": "John", "age": 30, "city": "New York"}
for key, value in person.items():
print(f"{key}: {value}")
As you can see, "for" loops are an essential part of Python programming. They help you iterate over data structures and perform operations on each item. With this foundation, you're ready to tackle more advanced topics, such as list comprehensions, dictionaries, and more!
Python for loop syntax
I'd be happy to help!
Python's for loop is used to iterate over a sequence (such as a list, tuple, or string) or other iterable objects. The basic syntax of the for loop is as follows:
for variable in iterable:
code block to be executed
Here:
variable
is the variable that will take on the value of each item in the iterable during each iteration. iterable
is the sequence or other iterable object being looped over.
For example, let's say you have a list of numbers and want to print them out:
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
This will output:
1
2
3
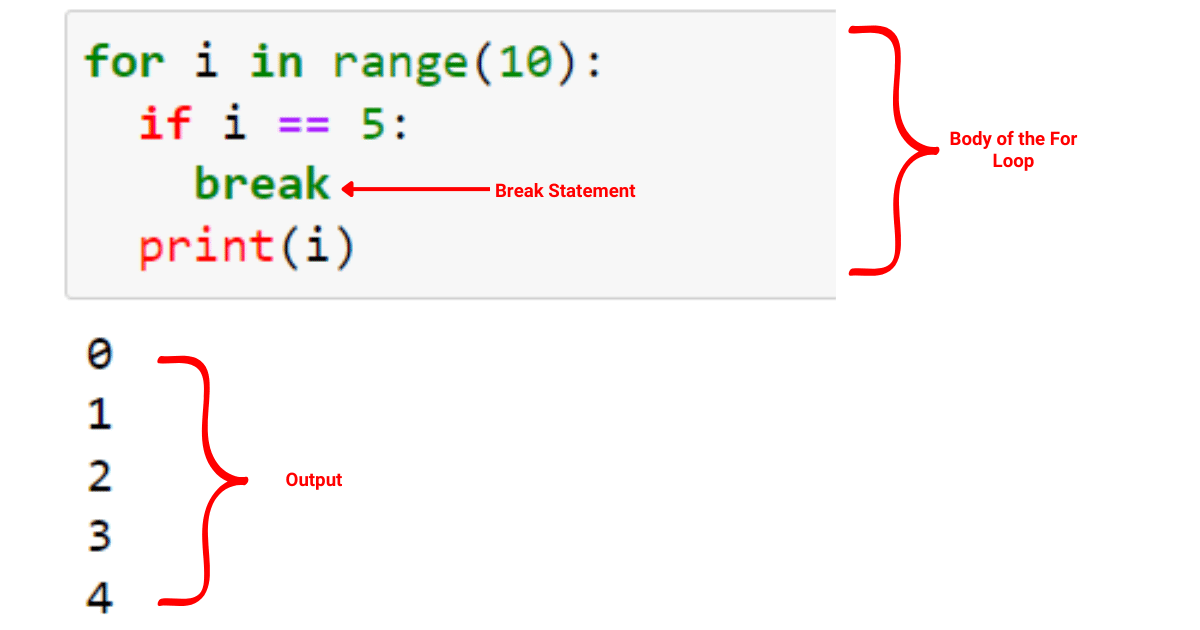
4
5
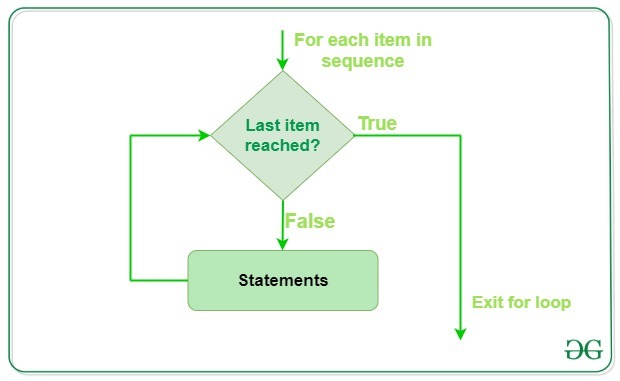
You can also use the enumerate
function to loop over both the index and value of each item in the iterable:
numbers = [1, 2, 3, 4, 5]
for i, num in enumerate(numbers):
print(f"Index: {i}, Value: {num}")
This will output:
Index: 0, Value: 1
Index: 1, Value: 2
Index: 2, Value: 3
Index: 3, Value: 4
Index: 4, Value: 5
You can also use the range
function to create an iterable sequence:
for i in range(10):
print(i)
This will output:
0
1
2
3
4
5
6
7
8
9
If you want to loop over multiple iterables at once, you can use the zip
function:
name = ["John", "Jane"]
age = [25, 30]
for n, a in zip(name, age):
print(f"{n} is {a} years old")
This will output:
John is 25 years old
Jane is 30 years old
You can also use the enumerate
function with multiple iterables:
name = ["John", "Jane"]
age = [25, 30]
for i, (n, a) in enumerate(zip(name, age)):
print(f"Index: {i}, Name: {n}, Age: {a}")
This will output:
Index: 0, Name: John, Age: 25
Index: 1, Name: Jane, Age: 30
Remember that in Python, the for
loop can be used to iterate over a wide range of data structures, including lists, tuples, strings, dictionaries, and more!
I hope this helps! Let me know if you have any questions or need further clarification.