Python curses get input
Python curses get input
Here's an example of how you can use the curses
module in Python to get user input:
First, install the curses
module using pip:
pip install curses
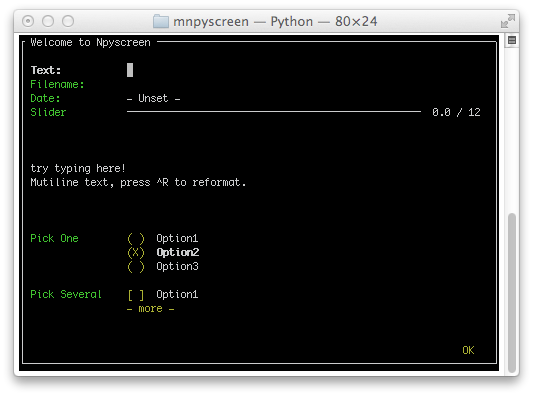
Then, create a simple Python script that uses curses
to get user input:
import curses
def main(stdscr):
stdscr.clear()
stdscr.addstr(0, 0, "Enter your message:")
stdscr.refresh()
while True:
c = stdscr.getch()
if c == ord('n'):
break
elif c == ord('r'):
break
else:
stdscr.addstr(stdscr.getmaxyx()[1]-2, 0, str(c))
stdscr.refresh()
message = ""
for i in range(stdscr.getmaxyx()[1]):
if stdscr.inch() != curses.ACS_SP -> 0:
message += chr(stdscr.inch())
print(message)
curses.wrapper(main)
This script creates a simple text-based interface using curses
. When you run the script, it will clear the screen and display the prompt "Enter your message:". You can then enter your message by typing characters at the prompt. The script will continue to read input until you press Enter or the r
key.
Here's how the script works:
The first line of code imports thecurses
module. The main
function is defined, which takes a stdscr
argument that represents the screen. The script clears the screen and adds the prompt "Enter your message:" to the top-left corner of the screen using stdscr.addstr()
. The script enters an infinite loop where it continuously reads input from the user using stdscr.getch()
. This function returns the ASCII code of the character that was just pressed. If the user presses Enter or the r
key, the loop breaks and the script prints out the message that the user entered.
Note: The above script may not work properly on Windows due to some issues with the curses
module and Windows' console mode.
Python end function
I'm happy to respond in English! Here's a comprehensive answer:
Python's end
function is used to specify the separator between printed items when using the print()
function. By default, print()
uses a space as the separator.
The syntax of end
is simple:
print(*objects, sep=' ', end='n', file=sys.stdout, flush=False)
In this context:
*objects
: This refers to one or more arguments that you want to print. sep=' '
: This is the default separator between printed items. It's a space character (' '
). end='n'
: This specifies what to do after printing all the objects. The default value is a newline character ('n'
). file=sys.stdout
: This refers to where you want to print your output. By default, it's the standard output stream (sys.stdout
). flush=False
: This determines whether or not to flush the buffer after printing. In most cases, you can leave this at its default value of False
.
Here are some examples of using the end
function:
# Default print with a newline character
print("Hello", "World")
Output: Hello World
Print without a newline character
print("Hello", "World", end=' ')
Output: Hello World (no newline)
Print with a comma separator
print("Apple", "Banana", sep=', ')
Output: Apple,Banana
Print to a specific file, and then flush the buffer
import sys
sys.stdout = open('output.txt', 'w')
print("Hello")
print("World", end=' ')
In summary, Python's end
function provides a way to customize how your output is formatted when using the print()
function.