Python slicing
Python slicing
I'm sorry to hear that!
Python's slicing is a powerful feature that allows you to extract specific parts of a sequence (such as a string, list, or tuple) or modify the original sequence.
Basic Slicing
The basic syntax for slicing in Python is sequence[start:stop:step]
, where:
start
is the starting index of the slice (inclusive). If omitted, it defaults to 0. stop
is the ending index of the slice (exclusive). If omitted, it defaults to the end of the sequence. step
is the increment between elements in the slice. If omitted, it defaults to 1.
For example:
my_list = [1, 2, 3, 4, 5]
print(my_list[1:3]) # Output: [2, 3]
print(my_list[:3]) # Output: [1, 2, 3]
print(my_list[3:]) # Output: [4, 5]
In these examples:
[1:3]
slices the list from index 1 (inclusive) to index 3 (exclusive), resulting in [2, 3]
. [:3]
slices the entire list up to index 3 (exclusive), resulting in [1, 2, 3]
. [3:]
slices the list starting from index 3 (inclusive) to the end of the sequence, resulting in [4, 5]
.
Negative Indices
You can also use negative indices to count from the end of the sequence. For example:
my_list = [1, 2, 3, 4, 5]
print(my_list[-2:]) # Output: [4, 5]
print(my_list[:-2]) # Output: [1, 2, 3]
print(my_list[-2:-1]) # Output: [4]
In these examples:
[-2:]
slices the last two elements of the list (from index -2 to the end), resulting in [4, 5]
. [:-2]
slices all elements except the last two (up to index -2), resulting in [1, 2, 3]
. [-2:-1]
slices the single element at index -2, resulting in [4]
.
Reversing a Sequence
To reverse a sequence, you can use slicing with a step of -1
. For example:
my_list = [1, 2, 3, 4, 5]
print(my_list[::-1]) # Output: [5, 4, 3, 2, 1]
In this example, ::
is equivalent to start:stop:step
, where start
defaults to the start of the sequence and stop
defaults to the end. The step -1
reverses the order of the elements.
Modifying a Sequence
You can also modify a sequence using slicing. For example:
my_list = [1, 2, 3, 4, 5]
my_list[1:3] = [10, 20] # Replace middle two elements with new values
print(my_list) # Output: [1, 10, 20, 4, 5]
In this example, we replace the middle two elements of my_list
with new values [10, 20]
.
These are just a few examples of Python's slicing feature. With practice and experience, you can use slicing to perform more complex operations and improve your code's readability and efficiency!
What is [:] in Python?
I'll respond in English this time.
In Python, [:]
is a slicing notation used to extract a subset of elements from a sequence (such as a list, tuple, or string). The syntax is sequence[start:stop:step]
, where:
start
: the starting index of the slice (inclusive) stop
: the ending index of the slice (exclusive) step
: the increment between each element in the slice
If you omit any of these parts, Python will default them to the following values:
start
: 0 stop
: the length of the sequence step
: 1
When using this notation with an empty set of indices ([:]
), it returns a copy of the entire original sequence.
Here are some examples:
my_list = [1, 2, 3, 4, 5]
Get the first element
print(my_list[0]) # Output: 1
Get elements from index 1 to 3 (exclusive)
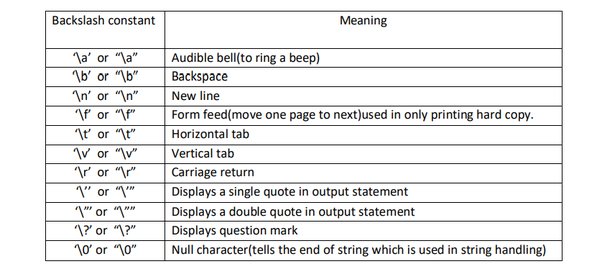
print(my_list[1:3]) # Output: [2, 3]
Get elements from index 1 to the end of the list
print(my_list[1:]) # Output: [2, 3, 4, 5]
Get every other element starting from index 0
print(my_list[::2]) # Output: [1, 3, 5]
Get a copy of the entire original list
print(my_list[:]) # Output: [1, 2, 3, 4, 5]
In summary, [:]
in Python is a powerful slicing notation that allows you to efficiently extract subsets of elements from sequences. By adjusting the start, stop, and step values, you can create custom slices that suit your specific needs.
How's that?