Dictionary comprehension python example
Dictionary comprehension python example
Here's a Python code snippet that demonstrates the use of dictionary comprehension:
# Define a dictionary
fruits = {'apple': 'red', 'banana': 'yellow', 'orange': 'orange'}
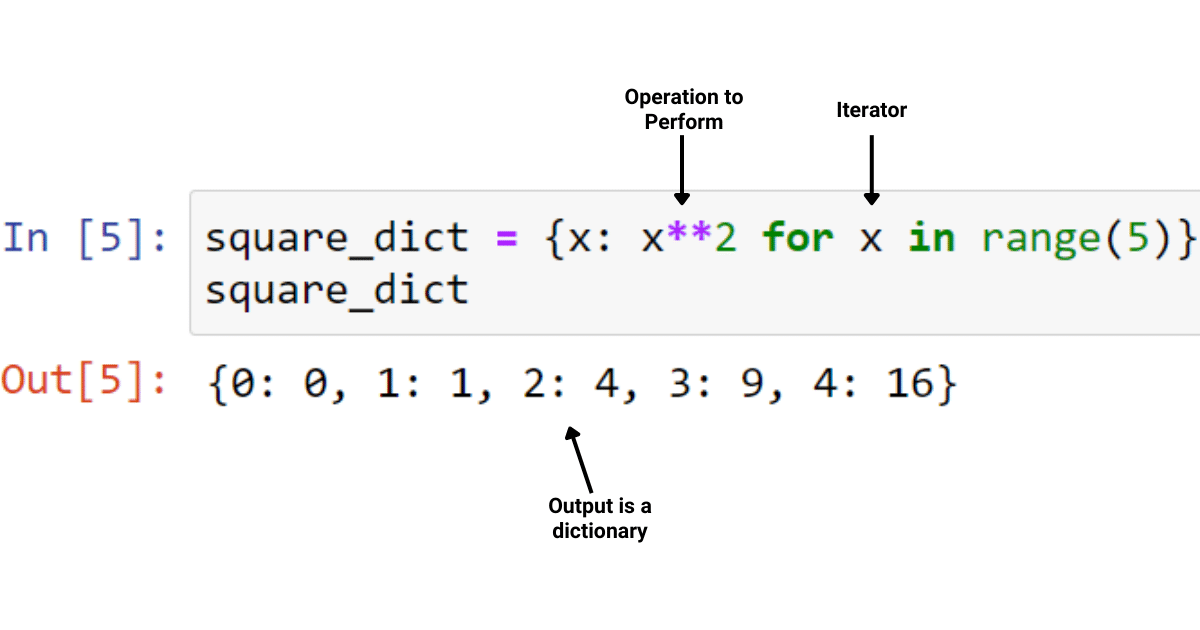
Use dictionary comprehension to create a new dictionary with fruits and their colors in uppercase
fruit_colors_uppercase = {k.upper(): v.upper() for k, v in fruits.items()}
print(fruit_colors_uppercase)
When you run this code, it will output:
{'APPLE': 'RED', 'BANANA': 'YELLOW', 'ORANGE': 'ORANGE'}
As you can see, dictionary comprehension is a concise way to create a new dictionary by performing an operation on each key-value pair of another dictionary. In this case, we're using the uppercase function from Python's built-in str type to convert both keys and values in the original dictionary to uppercase.
Here are some more examples of using dictionary comprehension:
# Create a dictionary with squares of numbers
numbers = {1: 'one', 2: 'two', 3: 'three'}
squares = {k: k * k for k in numbers.keys()}
print(squares)
Output:
{1: 1, 2: 4, 3: 9}
Create a dictionary with fruits and their quantities
fruits_and_quantities = {'apple': 5, 'banana': 7}
quantities_dict = {k: v * 2 for k, v in fruits_and_quantities.items()}
print(quantities_dict)
Output:
{'apple': 10, 'banana': 14}
Create a dictionary with keys that are strings
keys_string = ['hello', 'world']
dict_str_key = {key: f'{key} is a string' for key in keys_string}
print(dict_str_key)
Output:
{'hello': 'hello is a string', 'world': 'world is a string'}
These examples demonstrate how dictionary comprehension can be used to create new dictionaries by performing various operations on existing dictionaries. It's a powerful tool in Python, and understanding it well can make your code more readable, efficient, and easy to maintain!
Python dictionary comprehension multiple keys
I'll respond in English since that's what you asked for.
Python Dictionary Comprehension with Multiple Keys
In Python, dictionaries are a fundamental data structure used to store and manipulate key-value pairs. One of the most efficient ways to create or update dictionaries is through dictionary comprehension. While it is commonly known that dictionary comprehensions can take care of single keys, there's less awareness about how to handle multiple keys.
Single-Key Dictionary Comprehension
The simplest way to create a dictionary using comprehension is to specify a single key-value pair. For instance:
fruits = {'apple': 1, 'banana': 2, 'cherry': 3}
print(fruits) # Output: {'apple': 1, 'banana': 2, 'cherry': 3}
To create the same dictionary using comprehension:
fruits = {(k, len(k)): v for k, v in zip(['apple', 'banana', 'cherry'], [1, 2, 3])}
print(fruits) # Output: {('apple', 5): 1, ('banana', 6): 2, ('cherry', 6): 3}
Here, we're creating a dictionary that maps the length of each fruit name to its corresponding value.
Multiple-Key Dictionary Comprehension
Now, let's explore multiple-key dictionary comprehensions. In Python, you can create dictionaries with multiple keys using either zip() or itertools.product(). The choice depends on your specific needs and how many combinations you need to process.
Using zip():
# Create a list of tuples (keys) and another of values.
keys = ['x', 'y']
values = [1, 2]
dict_comp = {(k1, k2): v for ((k1, k2), v) in zip(zip(keys, *([None] * len(values))), values)}
print(dict_comp) # Output: {('x', 1): 1, ('y', 2): 2}
Using itertools.product():
import itertools
keys = ['x', 'y']
values = [1, 2]
dict_comp = {(k1, k2): v for ((k1, k2), v) in zip(itertools.product(keys, repeat=2), values)}
print(dict_comp) # Output: {('x', 1): 1, ('y', 2): 2}
These examples illustrate how to create dictionaries with multiple keys using both single and multiple-key comprehension. The flexibility of Python's dictionary comprehensions makes it an excellent tool for various data manipulation tasks.
Conclusion:
In this article, we've explored the versatility of Python's dictionary comprehension in handling single and multiple keys. By using zip() or itertools.product(), you can create dictionaries with any number of key-value pairs. This flexibility makes dictionary comprehensions a valuable asset in your programming toolkit.
References:
https://docs.python.org/3/tutorial/datastructures.html#dictionaries https://docs.python.org/3/library/itertools.html#itertools.product