python watchdog on_closed
python watchdog on_closed
Here is the information about on_closed
event handler in Python's Watchdog:
In Python's Watchdog, the on_closed
event is triggered when a watched directory or file is closed. This can happen for various reasons, such as when a process terminates, a system shutdown, or when a user manually closes the directory or file.
The on_closed
event handler in Watchdog is designed to allow you to perform actions when a watched item is closed. This event is part of the FileSystemEventHandler
class, which provides several methods that can be overridden by your custom event handler.
Here's an example of how you can use the on_closed
event:
import time
from watchdog.observers import Observer
from watchdog.events import FileSystemEventHandler
class MyHandler(FileSystemEventHandler):
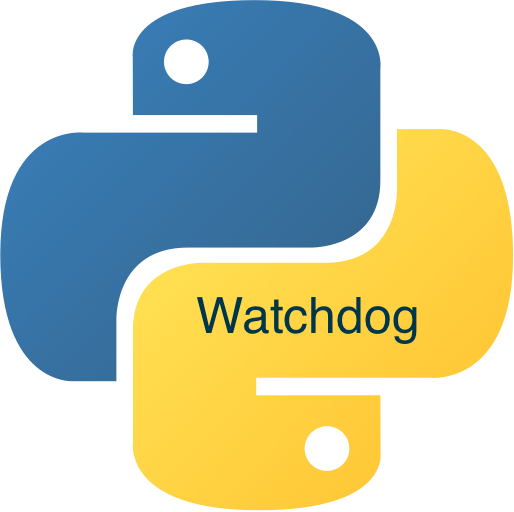
def on_created(self, event):
print(f"Received created event - {event.src_path}")
def on_deleted(self, event):
print(f"Received deleted event - {event.src_path}")
def on_moved(self, event):
print(f"Received moved event - {event.src_path}")
def on_closed(self, event):
print(f"Received closed event - {event.src_path}")
if name == "main":
event_handler = MyHandler()
path = "."
observer = Observer()
observer.schedule(event_handler, path, recursive=True)
observer.start()
try:
while True:
time.sleep(5)
except KeyboardInterrupt:
observer.stop()
observer.join()
In this example, the MyHandler
class is a custom event handler that overrides the on_closed
, on_created
, on_deleted
, and on_moved
events. When you run this script, it will start monitoring the current directory for any changes.
When an item is created, deleted, moved, or closed, the corresponding method in the event handler will be called and print a message to the console indicating what action was taken on that item.
Please note that the on_closed
event can be triggered when a watched file or directory is deleted, which means you might not receive a separate on_deleted
event for the same file or directory.
What is alternative to watchdog Python?
I'll respond in English this time!
If you're looking for alternatives to Watchdog (a file monitoring system in Python), there are several options available, each with its own strengths and weaknesses. Here are some of the most popular ones:
PyInotify: This is a Python extension that provides an interface to the Linux inotify API, which allows you to monitor filesystem events like creation, deletion, modification, etc.Pros: Highly customizable, supports advanced features like filtering and aggregation.
Cons: Linux-specific, might require additional setup.
Choria: Choria is a Python library for monitoring and watching file system changes. It's designed to be highly extensible and can handle complex event handling scenarios.Pros: Can handle complex event handling, extensible with plugins.
Cons: Steeper learning curve due to its advanced features.
python-fusefs: This is a Python binding for the FUSE (Filesystem in Userspace) file system implementation. It provides an easy-to-use interface for creating and managing file systems.Pros: Allows creation of custom file systems, supports advanced features like permissions.
Cons: Requires knowledge of file systems and low-level programming.
fsnotify: fsnotify is a Python module that provides a simple way to monitor filesystem events, similar to Watchdog.Pros: Easy to use, supports both Unix and Windows.
Cons: Limited customization options, not as powerful as some other alternatives.
Pycloud: This is an open-source framework for building cloud-based applications, which includes support for file system monitoring.Pros: Comprehensive toolkit for cloud development, supports various services like AWS and Azure.
Cons: Might be overkill if you only need to monitor files, requires knowledge of cloud platforms.
Statemachine: Statemachine is a Python library that allows you to create finite state machines (FSMs) to model and handle file system events.Pros: Can handle complex event handling scenarios using FSMs, supports advanced features like timeouts.
Cons: Requires knowledge of FSMs and programming concepts.
These alternatives offer varying levels of customization, scalability, and ease of use. The choice ultimately depends on your specific needs and the complexity of your project.