How to specify input type in Python function
How to specify input type in Python function
I'll respond in English this time!
In Python, specifying the input type for a function is crucial for ensuring that the function receives the correct data and behaves as expected. There are several ways to do this:
Function Signatures: In Python, you can specify the input types using thedef
statement when defining a function. For example:
def add(x: int, y: int) -> int:
return x + y
In this example, the function add
takes two integer arguments (x
and y
) and returns an integer value.
->
syntax. You can use this syntax to specify the return type of a function:
def greet(name: str) -> None:
print(f"Hello, {name}!")
In this example, the function greet
takes a string argument (name
) and returns None
.
mypy
: A static type checker that checks your code against type hints. pytype
: A Python type system that allows you to specify type annotations for variables and functions. typing
: The built-in typing module in Python, which provides support for generic types, unions, and other advanced type concepts.
For example, with the mypy
library, you can add type hints to your function definitions:
Docstrings: While not strictly related to type hints, docstrings can help you document the expected input and output types for your functions. For example:from mypy import annotate
def add(x: int, y: int) -> int:
return x + y
@annotate(type=complex)
def complex_add(x: complex, y: complex) -> complex:
return x + y
def greet(name: str) -> None:
"""
Greet a person by name.
Args:
name (str): The name of the person to greet.
Returns:
None: No return value.
"""
print(f"Hello, {name}!")
By specifying input types and docstrings, you can make your code more readable, maintainable, and easy to understand. This is especially important when working with large or complex projects where type hints can help catch errors earlier in the development process.
Remember that while type hints are useful for documentation and static analysis, Python is a dynamically-typed language at its core. It's still possible for a function to receive unexpected input types and behave correctly (although this might not be what you intended!).
Python input type example
I'd be happy to provide an example of Python input type! Here's a brief explanation:
Python is a high-level programming language that is known for its simplicity, flexibility, and ease of use. One of the key features of Python is its ability to handle various types of input data.
Here are some common types of input in Python:
Integers (int): These are whole numbers, such as 1, 2, 3, etc. In Python, you can define an integer using theint()
function. For example:
num = int(input("Enter a number: "))
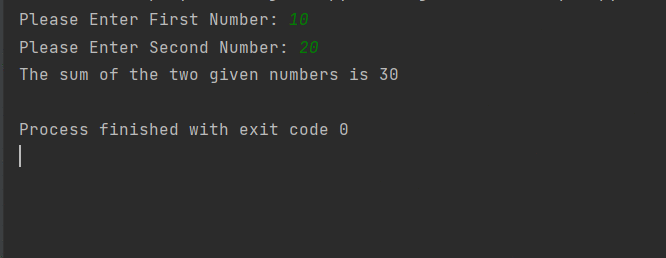
print(num)
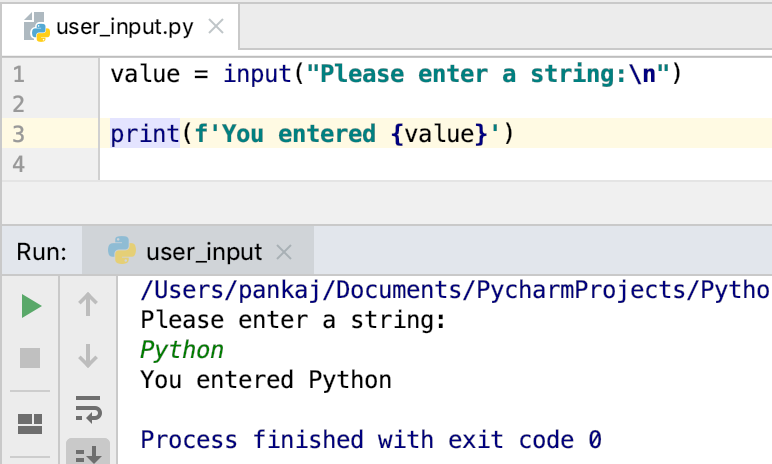
In this example, the user is prompted to enter a number, and the input is stored in the num
variable.
float()
function. For example:
x = float(input("Enter a number with decimals: "))
print(x)
In this example, the user is prompted to enter a number with decimal places, and the input is stored in the x
variable.
""
). For example:
name = str(input("Enter your name: "))
print(name)
In this example, the user is prompted to enter their name, and the input is stored in the name
variable.
bool()
function. For example:
agree = bool(input("Do you agree? (yes/no): "))
print(agree)
In this example, the user is prompted to enter either "yes" or "no", and the input is stored in the agree
variable.
None
keyword. For example:
answer = None
print(answer)
In this example, the answer
variable is set to None, indicating that there is no value assigned.
Here's an example of how these input types can be used in a program:
def main():
num1 = int(input("Enter first number: "))
operator = str(input("Choose operator (+, -, x, /): "))
num2 = float(input("Enter second number: "))
if operator == "+":
result = num1 + num2
elif operator == "-":
result = num1 - num2
elif operator == "*":
result = num1 * num2
elif operator == "/":
if num2 != 0:
result = num1 / num2
else:
print("Error! Division by zero!")
return
print("Result:", result)
main()
In this program, the user is prompted to enter two numbers and an operator. The program then performs a calculation based on the operator and prints the result.
These are just a few examples of input types in Python. By using various input types, you can create programs that interact with users in a wide range of ways!