python global variables in function
python global variables in function
I'd be happy to explain the concept of using global variables in Python functions.
What are Global Variables?
In Python, a global variable is a variable that is defined outside a function and can be accessed from within any function. In other words, global variables have scope beyond a single function and can be used across multiple functions.
Why Use Global Variables?
You might wonder why you would want to use global variables when you have local variables (variables defined inside a function). Well, there are several reasons:
Sharing Data: You might want to share data between multiple functions, and global variables make it easy to do so. State Management: Global variables can be used to manage the state of your program. For example, you could use a global variable to keep track of the number of times a function is called. Convenience: Sometimes, using a global variable can simplify your code and make it more readable.How Do You Use Global Variables?
To use a global variable in Python, you simply define the variable outside any function, like this:
my_global_var = 5
Then, you can access and modify the variable from within any function:
def my_function():
print(my_global_var) # prints 5
my_global_var += 1
Note that if you want to modify a global variable inside a function, you need to use the global
keyword to indicate that you intend to modify the global variable:
def my_function():
global my_global_var
print(my_global_var) # prints 5
my_global_var += 1
Best Practices
When using global variables, keep the following best practices in mind:
Use Global Variables Sparingly: Try to use global variables only when necessary. Overuse of global variables can make your code harder to understand and maintain. Document Your Global Variables: Make sure to document what each global variable is used for, so others (and yourself!) can understand the code better. Avoid Modifying Global Variables from Within Functions: Unless you have a good reason to do so, try to avoid modifying global variables from within functions. Instead, use local variables or pass arguments to functions.In summary, using global variables in Python functions is a valid way to share data and manage state between multiple functions. However, it's essential to use them sparingly and follow best practices to ensure your code remains readable and maintainable.
Let me know if you have any questions!
python global multiple variables
I'll respond in English as requested!
In Python, you can use the global
keyword to declare a variable as global, allowing it to be accessed and modified from within functions or methods.
When a variable is assigned within a function, it defaults to being local to that function. To make the variable accessible outside of the function, you need to specify the global
keyword before the assignment.
Here are some examples:
Simple Global Assignment:x = 10
def foo():
global x
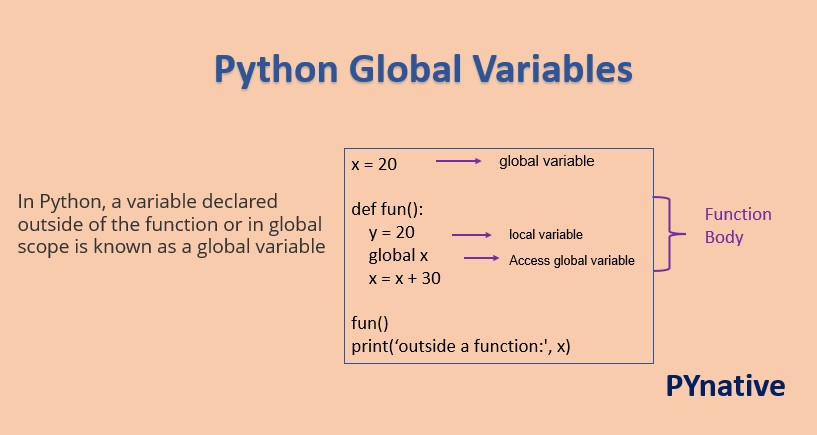
x = 20
print(x) # prints 20
foo()
print(x) # prints 20, because x was modified globally
In this example, x
is initially assigned a value of 10
. Then, within the foo()
function, we use global x
to declare that we want to modify the global variable x
. After assigning a new value to x
, we print it within the function. Finally, when we call foo()
, and then print x
again outside of the function, we see that the value has been modified globally.
a = 10
b = 20
def foo():
global a, b
a = 30
b = 40
print(a) # prints 30
print(b) # prints 40
foo()
print(a) # prints 30
print(b) # prints 40
In this example, we have two global variables a
and b
, initially assigned values of 10
and 20
, respectively. Within the foo()
function, we use global a, b
to declare that we want to modify both variables globally. After assigning new values to a
and b
, we print them within the function. Finally, when we call foo()
, and then print a
and b
again outside of the function, we see that both values have been modified globally.
class MyClass:
x = 10
def method(self):
global MyClass.x
self.x = 20
print(self.x) # prints 20
obj = MyClass()
print(obj.x) # prints 10
obj.method()
print(obj.x) # prints 20
In this example, we define a class MyClass
with an instance variable x
. Within the method()
of the class, we use global MyClass.x
to declare that we want to modify the global variable x
. After assigning a new value to x
, we print it within the method. Finally, when we create an instance of the class and call its method, and then print x
again outside of the method, we see that the value has been modified globally.
Remember that using global
variables can lead to namespace pollution and make your code harder to understand and maintain. It's generally recommended to use functions or classes with well-defined scopes instead of relying on global variables.