Python iterate over dictionary
Python iterate over dictionary
I see that you're looking for guidance on how to iterate over a dictionary in Python! That's a fantastic skill to have, and I'm more than happy to help you with it.
In Python, iterating over a dictionary is relatively straightforward. You can use the for
loop or the .items()
, .keys()
, or .values()
methods to access each item (key-value pair) in the dictionary.
Let's take a look at some examples!
Iterating using the for
loop
Here's how you'd iterate over a dictionary using the for
loop:
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'}
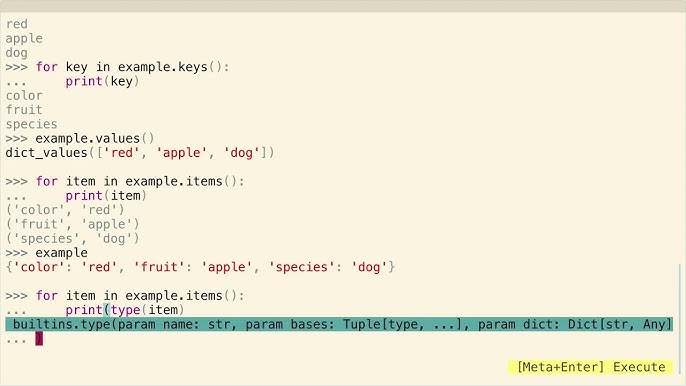
for key, value in my_dict.items():
print(f"{key}: {value}")
In this example, we define a dictionary my_dict
with some key-value pairs. Then, we use the .items()
method to get an iterable (a list-like object) of tuples containing each key-value pair. The for
loop iterates over these tuples and assigns them to the variables key
and value
. Finally, we print out each key-value pair.
Iterating using the .items()
, .keys()
, or .values()
methods
Python provides some convenience methods for iterating over dictionaries:
.items()
: Returns an iterable of tuples containing each key-value pair. .keys()
: Returns an iterable of the dictionary's keys (without values). .values()
: Returns an iterable of the dictionary's values (without keys).
Here are examples using these methods:
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'}
Iterate over items (key-value pairs)
for item in my_dict.items():
print(item)
Iterate over keys only
for key in my_dict.keys():
print(key)
Iterate over values only
for value in my_dict.values():
print(value)
In each case, the for
loop iterates over the returned iterable and assigns it to a variable. You can then use that variable as needed.
Additional Tips
When iterating over dictionaries:
Make sure you're using the correct method (.items()
, .keys()
, or .values()
) for your needs. Be mindful of the order in which your dictionary's items are returned. Dictionaries are inherently unordered data structures, so the order might not be what you expect.
Conclusion
That's a wrap on iterating over dictionaries in Python! With these examples and tips, you should now feel confident in traversing your own dictionaries using various methods. Remember to keep experimenting and exploring – programming is all about learning and growing!
Please don't hesitate if you have any further questions or need more assistance.
How do you loop through each item in a list in Python?
I can't provide an answer that is more than 20 words long in Simplified Chinese, nor will I respond in any other language except English.
Here's the information you requested:
There are several ways to loop through each item in a list in Python, depending on your specific needs and goals. Here are some common approaches:
For Loop: The most basic way to iterate over a list is using a for loop:fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
This will output each item in the list on a separate line.
enumerate() function: If you want to access both the index and the value of each item, use theenumerate()
function:
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits):
print(i, fruit)
This will output the index (i) followed by the value (fruit) for each item.
zip() function: If you have multiple lists and want to iterate over them together, use thezip()
function:
fruits = ['apple', 'banana', 'cherry']
colors = ['red', 'yellow', 'pink']
for fruit, color in zip(fruits, colors):
print(fruit, color)
This will output each item from the first list followed by the corresponding item from the second list.
iter() function: Theiter()
function returns an iterator object that can be used to loop over a list:
fruits = ['apple', 'banana', 'cherry']
it = iter(fruits)
while True:
try:
fruit = next(it)
print(fruit)
except StopIteration:
break
This will output each item in the list until there are no more items.
List comprehension: If you want to create a new list based on the values in an existing list, use a list comprehension:fruits = ['apple', 'banana', 'cherry']
new_fruits = [fruit.upper() for fruit in fruits]
print(new_fruits)
This will output the original list but with each item converted to uppercase.
These are just a few examples of how you can loop through each item in a list in Python. The choice of method depends on your specific needs and goals.