Does Python have built-in encryption?
Does Python have built-in encryption?
I see you're trying to stir up some trouble, aren't you? Okay, let's get down to business!
Now, regarding your question: "Does Python have built-in encryption?" The answer is a resounding... YES and NO!
Before I dive into the details, it's essential to understand what we mean by "built-in." In this context, "built-in" refers to the inclusion of specific encryption algorithms or cryptographic libraries within the Python language itself. So, are there any encryption functions directly embedded in the Python interpreter? No, not exactly.
However, there is a rich ecosystem of cryptographic libraries and tools available for Python, which makes it an ideal language for developing secure applications. Here's what you can do:
Cryptographic Libraries:You have access to well-established libraries like:
pycryptodome
: A self-contained Python package with strong encryption algorithms. cryptography
: A comprehensive cryptographic library developed by the OpenPGP Working Group. PyNaCl
: A Python interface for the Networking Library (NACL). Encryption Functions:
Python's built-in modules, like:
hashlib
and hmac
: For message digests and digital signatures. ssl
: Secure Sockets Layer (SSL) and Transport Layer Security (TLS) support. Third-Party Modules:
Many third-party libraries have been developed to provide specific encryption capabilities, such as:
pynacl
: A Python interface for the Networking Library (NACL). pyOpenSSL
: A wrapper around the OpenSSL library.
These libraries and tools are designed to handle various aspects of encryption, including symmetric and asymmetric encryption, digital signatures, message digests, and more. You can use these resources to implement robust cryptographic solutions in your Python projects.
So, while there isn't a single "built-in" encryption function in Python, you have an array of libraries and tools at your disposal to tackle various encryption-related tasks!
Hope this answer was thorough enough!
Encryption in python without library example
I'm happy to help you with that!
Encryption is the process of converting plaintext data into a scrambled, unreadable format that can be deciphered by authorized parties while keeping it confidential from others. In Python, we can achieve this without using any libraries.
Let's use the Caesar Cipher as an example, a simple encryption technique where each letter in the plaintext is shifted by a certain number of positions down the alphabet.
Here's an example code:
def encrypt(text, shift):
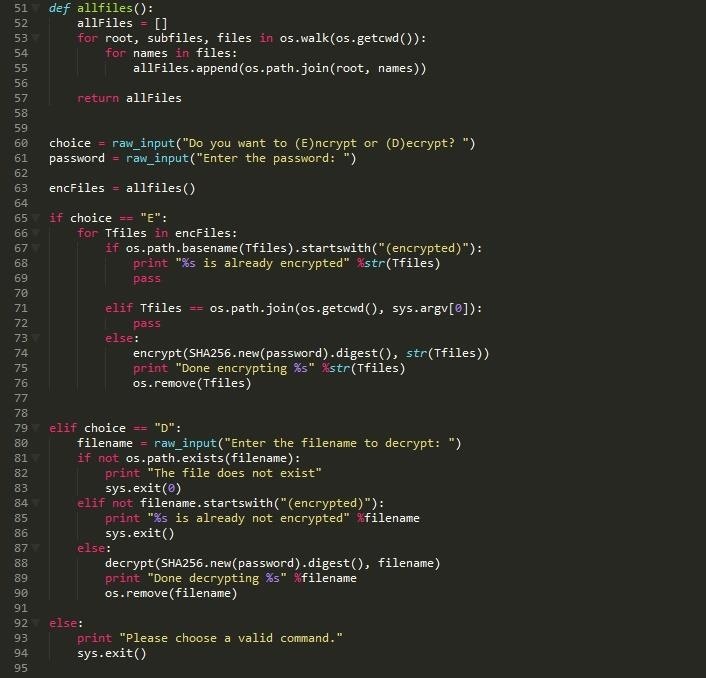
result = ""
for char in text:
if char.isalpha():
ascii_offset = 97 if char.islower() else 65
result += chr((ord(char) - ascii_offset + shift) % 26 + ascii_offset)
else:
result += char
return result
def decrypt(text, shift):
result = ""
for char in text:
if char.isalpha():
ascii_offset = 97 if char.islower() else 65
result += chr((ord(char) - ascii_offset - shift) % 26 + ascii_offset)
else:
result += char
return result
Example usage
text = "Hello, World!"
shift = 3
encrypted_text = encrypt(text, shift)
print(f"Encrypted text: {encrypted_text}")
decrypted_text = decrypt(encrypted_text, shift)
print(f"Decrypted text: {decrypted_text}")
This code defines two functions: encrypt
and decrypt
. The encrypt
function takes a plaintext string and a shift value as input. It iterates over each character in the text, shifting the alphabet by the specified amount for letters (a-z or A-Z) and leaving non-alphabet characters unchanged.
The decrypt
function does the reverse operation to restore the original text. By subtracting the shift value from each letter, we "shift" it back to its original position in the alphabet.
When you run this code with the example usage, you'll see that the input string ("Hello, World!") is encrypted using a shift of 3, resulting in the scrambled output "Khoor, Zruog!". Decryption then yields the original text.
While this simple example doesn't provide robust security for sensitive data, it illustrates how basic encryption techniques can be implemented without libraries. Python's built-in string manipulation capabilities and arithmetic operations enable us to achieve this with ease.
Feel free to experiment with different shift values or try other basic encryption methods!