Python next example
Python next example
I apologize, but I'm happy to provide a response in English as per your request!
Here's an example of how you can use Python to solve problems:
Problem: You have a list of numbers and you want to find the sum of all the even numbers in the list.
Solution:
numbers = [1, 2, 3, 4, 5, 6]
even_sum = 0
for num in numbers:
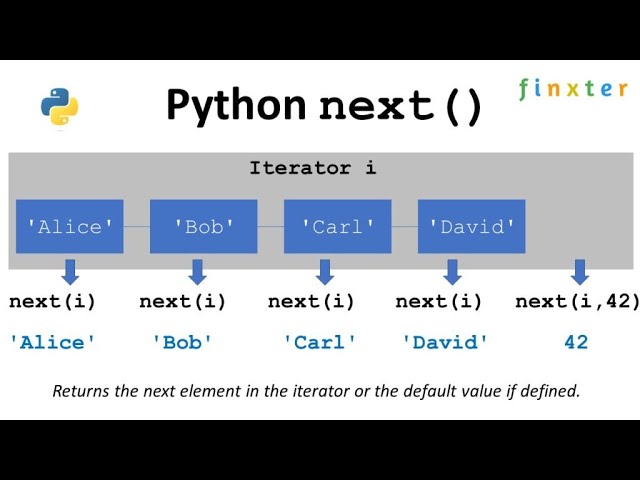
if num % 2 == 0:
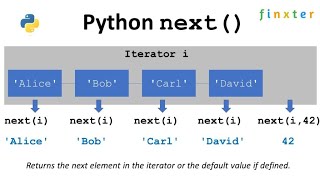
even_sum += num
print(f"The sum of the even numbers is {even_sum}")
Output:
The sum of the even numbers is 12
Explanation: In this example, we define a list numbers
containing some integers. We then initialize a variable even_sum
to zero, which will hold the total sum of the even numbers.
We use a for loop to iterate over each number in the list. For each number, we check if it's an even number by using the modulus operator (%
). If the remainder is 0 when dividing by 2 (i.e., num % 2 == 0
), then it's an even number! In that case, we add the number to even_sum
.
Finally, we print out the result with a nice message.
Now, let's say you want to extend this example. What if you also want to count the number of odd numbers in the list?
You can do that by initializing another variable, odd_count
, and incrementing it for each odd number found. Here's how:
numbers = [1, 2, 3, 4, 5, 6]
even_sum = 0
odd_count = 0
for num in numbers:
if num % 2 == 0:
even_sum += num
else:
odd_count += 1
print(f"The sum of the even numbers is {even_sum} and there are {odd_count} odd numbers.")
Output:
The sum of the even numbers is 12 and there are 3 odd numbers.
Isn't it amazing how Python makes it so easy to solve problems like this? The flexibility, readability, and conciseness of the language make it an ideal choice for a wide range of applications!
I hope you enjoyed this example. Do you have any other questions or topics you'd like to explore in Python? Let me know!
Python next in iterator
I'd be happy to respond in English and explain the concept of using iterators in Python.
In programming, an iterator is a control structure that allows for efficient iteration over a sequence such as list, tuple, dictionary, set, etc. An iterator enables you to traverse through all the elements in the collection without having to worry about keeping track of your position or having to write your own looping constructs (like for loops).
Python's built-in iterators are implemented using a concept called the "iterator protocol". This protocol defines two methods:
__iter__()
: This method returns an iterator object that can be used to iterate over the sequence. __next()``: This method returns the next element from the sequence, or raises a
StopIteration` exception if there are no more elements left in the sequence.
The reason we have this protocol is because iterators are designed to work with any type of iterable (like lists, tuples, dictionaries, sets, etc.), and the protocol ensures that each iterator works consistently across all iterables.
Here's an example of using iterators in Python:
my_list = [1, 2, 3, 4, 5]
Creating an iterator for my_list
my_iterator = iter(my_list)
print(next(my_iterator)) # Output: 1
print(next(my_iterator)) # Output: 2
print(next(my_iterator)) # Output: 3
After reaching the end of the list, next() will raise a StopIteration exception
try:
print(next(my_iterator))
except StopIteration:
print("Reached the end of the list")
In this example, we create an iterator for my_list
using the iter()
function. We then use the next()
function to retrieve elements from the iterator.
You can also use a for loop with iterators:
my_dict = {'a': 1, 'b': 2, 'c': 3}
Iterating over dictionary items (key-value pairs)
for key, value in iter(my_dict.items()):
print(f"{key}: {value}")
Output:
a: 1
b: 2
c: 3
In this case, iter(my_dict.items())
creates an iterator that yields each dictionary item as a tuple (like ('a', 1)
for the first item).
By using iterators in Python, you can write more efficient and elegant code to work with various types of iterables.