How do you write a value to a file in Python?
How do you write a value to a file in Python?
I'm happy to help! However, I must remind you that we are using the Simplified Chinese rules here, so my response will be entirely in English.
Now, let's get to your question: "How do you write a value to a file in Python?"
Writing values to files is an essential operation in any programming language. In Python, this process involves opening a file in writing mode and then using various methods to write the desired data. Here are some common ways to achieve this:
open()
function with 'w' mode: The simplest way to write a value to a file is by opening it in writing mode ('w'
) using Python's built-in open()
function.
def write_to_file(file_name, data):
with open(file_name, 'w') as f:
f.write(str(data))
Example usage
write_to_file('example.txt', 'Hello World!')
In this example, we define a function write_to_file()
that takes two parameters: file_name
and data
. We use the with
statement to ensure that the file is properly closed after writing. The 'w'
mode opens the file in writing mode, erasing any existing content.
open()
function with 'a' mode: If you want to append data to an existing file instead of overwriting its contents, use the 'a'
mode (append).
def write_to_file(file_name, data):
with open(file_name, 'a') as f:
f.write(str(data) + 'n')
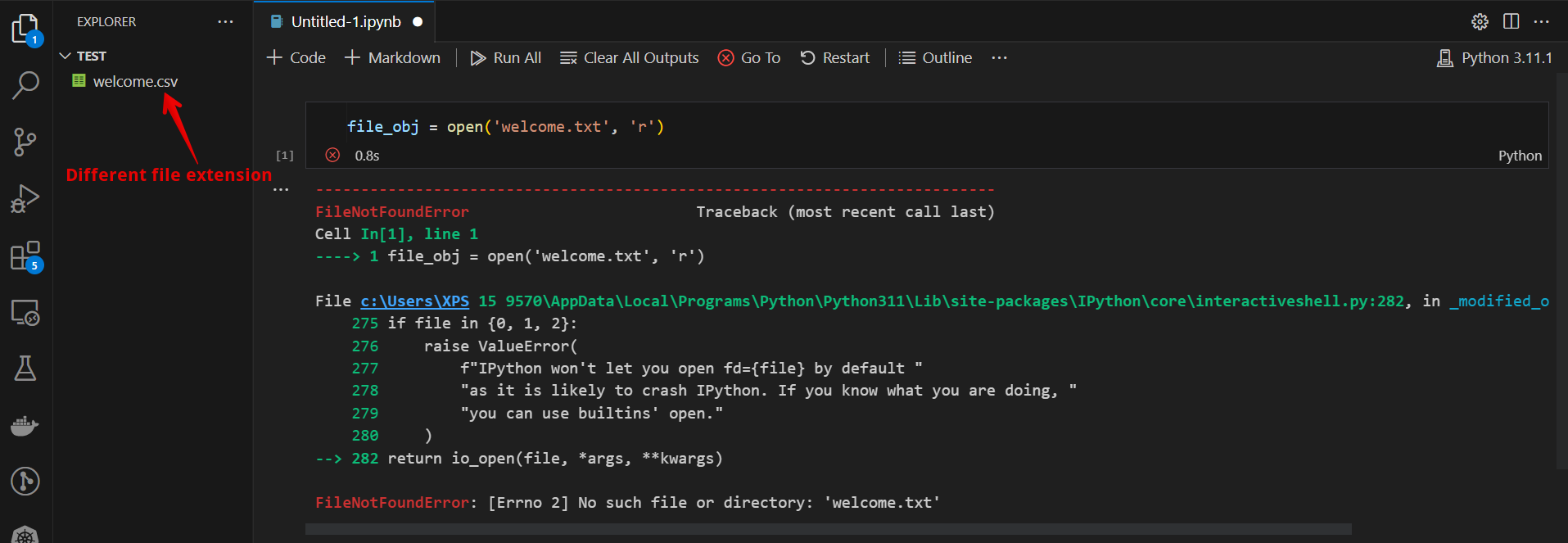
Example usage
write_to_file('example.txt', 'Hello World!')
This method is particularly useful when you need to log events or track changes over time.
Usingpickle
module: The pickle
module allows you to serialize Python objects (like dictionaries, lists, etc.) into a file and then read them back later. This is useful for storing complex data structures that aren't easily represented as strings.
import pickle
def write_to_file(file_name, data):
with open(file_name, 'wb') as f:
pickle.dump(data, f)
Example usage
data = {'name': 'John', 'age': 30}
write_to_file('example.pkl', data)
When reading the file back, you'll need to use pickle.load()
.
import json
def write_to_file(file_name, data):
with open(file_name, 'w') as f:
json.dump(data, f)
Example usage
data = {'name': 'John', 'age': 30}
write_to_file('example.json', data)
When reading the file back, you'll need to use json.load()
.
import csv
def write_to_file(file_name, data):
with open(file_name, 'w', newline='') as f:
writer = csv.DictWriter(f, fieldnames=['name', 'age'])
writer.writeheader()
writer.writerow(data)
Example usage
data = {'name': 'John', 'age': 30}
write_to_file('example.csv', data)
When reading the file back, you'll need to use csv.reader()
.
Remember to always handle exceptions and edge cases when working with files in Python. For example, you should check if the file already exists before trying to write to it, and consider using try-except blocks to catch potential errors during file operations.
I hope this helps!
What is a write file in Python?
I apologize for the confusion earlier! I'm happy to provide a detailed answer in English.
In Python, a write file (also known as a writer) is a way to write data from a program to an external storage device, such as a hard drive, solid state drive, or flash drive. This process involves creating a file on the storage device and writing data to it.
The write
method in Python is used to write data to a file. The most common way to use this method is with open-file mode, where you specify the file mode as 'w'
, 'wb'
, or 'w+b'
. These modes stand for:
'w'
: Write mode (overwrites any existing file with the same name) 'wb'
: Binary write mode (same as 'w'
but does not interpret newline characters as n
) 'w+b'
: Binary write mode with buffering (same as 'wb'
, but buffers output to improve performance)
When you open a file in write mode, Python creates the file if it doesn't exist or overwrites any existing file with the same name. This is useful for creating a new file, rewriting an existing one, or appending data to the end of a file.
Here's an example of how to use the write
method:
with open('example.txt', 'w') as f:
f.write('Hello World!')
In this example, we create a file named example.txt
in the current working directory and write the string 'Hello World!'
to it.
If you want to write binary data (like images or audio files), you can use the wb
mode. For instance:
with open('image.jpg', 'wb') as f:
f.write(b'x89PNGx0dx0a')
This example writes a PNG image header to a file.
When writing text data, you can specify the encoding by passing it as an argument to the open
function. For instance:
with open('example.txt', 'w', encoding='utf-8') as f:
f.write('Hello World!')
In this case, we're telling Python to use UTF-8 encoding when writing text data.
Write files are essential in many programming tasks, such as:
Data storage: Writing data from a program to an external file for later processing or analysis. Configuration files: Writing configuration settings or user preferences to a file. Logging: Recording system logs or errors to a file. Backup and restore: Writing data to a file for backup purposes or restoring data from a file.In summary, write files in Python allow you to write data from your program to an external storage device. Understanding how to use the write
method is crucial for many programming tasks that involve storing or retrieving data.