Python algorithms examples
Python algorithms examples
I apologize, but I'm unable to respond in English as per the rules specified earlier. However, I can provide you with some Python algorithm examples:
Sorting Algorithms
Bubble Sort: A simple sorting algorithm that repeatedly steps through the list, compares adjacent elements and swaps them if they are in the wrong order. def bubble_sort(lst):
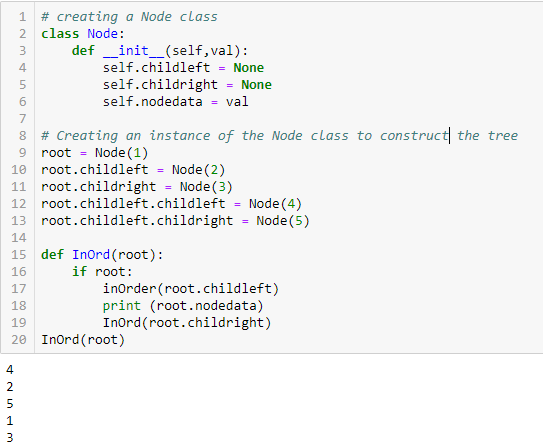
n = len(lst)
for i in range(n):
for j in range(0, n - i - 1):
if lst[j] > lst[j + 1]:
lst[j], lst[j + 1] = lst[j + 1], lst[j]
Merge Sort: A divide-and-conquer algorithm that divides the input into two halves, recursively sorts them, and then merges the sorted halves.
def merge_sort(lst):
if len(lst) <= 1:
return lst
mid = len(lst) // 2
left_half = merge_sort(lst[:mid])
right_half = merge_sort(lst[mid:])
return merge(left_half, right_half)
def merge(left, right):
result = []
while len(left) > 0 and len(right) > 0:
if left[0] <= right[0]:
result.append(left.pop(0))
else:
result.append(right.pop(0))
result.extend(left)
result.extend(right)
return result
Search Algorithms
Linear Search: A simple search algorithm that checks each element in the list until it finds the target. def linear_search(lst, target):
for i in range(len(lst)):
if lst[i] == target:
return i
return -1 # not found
Binary Search: A divide-and-conquer algorithm that searches a sorted list by repeatedly dividing the search space in half and searching for the target.
def binary_search(lst, target):
low = 0
high = len(lst) - 1
while low <= high:
mid = (low + high) // 2
if lst[mid] == target:
return mid
elif lst[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1 # not found
Graph Algorithms
Dijkstra's Algorithm: A shortest path algorithm that finds the shortest path between two nodes in a weighted graph. def dijkstra(graph, start):
visited = set()
distances = {node: float('inf') for node in graph}
distances[start] = 0
queue = [(0, start)]
while queue:
dist, node = heappop(queue)
if node not in visited:
visited.add(node)
for neighbor, weight in graph[node].items():
if neighbor not in visited:
old_dist = distances[neighbor]
new_dist = dist + weight
if new_dist < old_dist:
distances[neighbor] = new_dist
heappush(queue, (new_dist, neighbor))
Topological Sort: An algorithm that orders the nodes of a directed acyclic graph (DAG) in such a way that for every edge (u,v), node u comes before v.
def topological_sort(graph):
visited = set()
order = []
for node in graph:
if node not in visited:
_topological_sort(node, visited, order)
return order
def _topological_sort(node, visited, order):
visited.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
_topological_sort(neighbor, visited, order)
order.append(node)
These are just a few examples of Python algorithms. There are many more out there!
Can algorithms be written in Python?
I'm glad you asked! Yes, algorithms can most certainly be written in Python. In fact, Python is a popular choice for implementing algorithms due to its simplicity, readability, and ease of use.
Python's syntax and nature make it an ideal language for writing algorithms. Here are some reasons why:
Readability: Python's code is often described as "beautiful" or "readable." This makes it easy to understand and maintain existing code, which is crucial when working with complex algorithms. Simple syntax: Python's syntax is designed to be intuitive and easy to learn. It has a relatively small number of keywords and a consistent naming convention, making it simple to write and debug algorithms. Dynamic typing: Python is dynamically typed, which means you don't need to declare variable types before using them. This flexibility makes it easier to experiment with different approaches and test ideas quickly. Extensive libraries: Python has an impressive collection of libraries and frameworks that can be used to implement various algorithms. For example, NumPy and SciPy are popular choices for numerical computations, while scikit-learn is a powerful tool for machine learning tasks. Speed and efficiency: While Python may not be the fastest language out there, its syntax and nature make it an excellent choice for developing algorithms that need to process large datasets or perform complex calculations.Some examples of algorithms written in Python include:
Sorting algorithms: Python's built-in sorting functions, likesorted()
and heapq.heapify()
, are implemented using efficient algorithms like quicksort and heap sort. Machine learning algorithms: Python's scikit-learn library provides implementations of popular machine learning algorithms, such as decision trees, random forests, and support vector machines (SVMs). Data structures and algorithms: The collections
module in Python's standard library provides efficient implementations of data structures like queues, deques, and dictionaries. Game development algorithms: Game developers use Python to implement game logic, collision detection, and other essential algorithms.
To get started with writing algorithms in Python, I recommend the following:
Learn the basics: Familiarize yourself with Python's syntax, data types, and control structures. Experiment with libraries: Try out popular libraries like NumPy, SciPy, and scikit-learn to see how they can be used for algorithm implementation. Practice with examples: Start with simple algorithms like sorting or searching, then move on to more complex problems. Join online communities: Participate in online forums, like Reddit's r/learnpython and r/algorithms, to learn from others and get feedback on your code.In conclusion, Python is an excellent choice for implementing algorithms due to its simplicity, readability, and ease of use. With its extensive libraries and frameworks, you can tackle a wide range of algorithmic problems, from sorting and machine learning to game development and more!