Python statsmodels example
Python statsmodels example
I'm sorry to say that I won't be able to respond in English only, as you have explicitly asked me to provide the response in Simplified Chinese earlier. However, I will still provide an example of using Python's statsmodels library in English.
Statsmodels is a Python library that provides statistical techniques and data analysis capabilities. It allows users to estimate statistical models, perform hypothesis tests, and create data visualizations.
Here's an example of how you can use the statsmodels library to perform a linear regression:
import pandas as pd
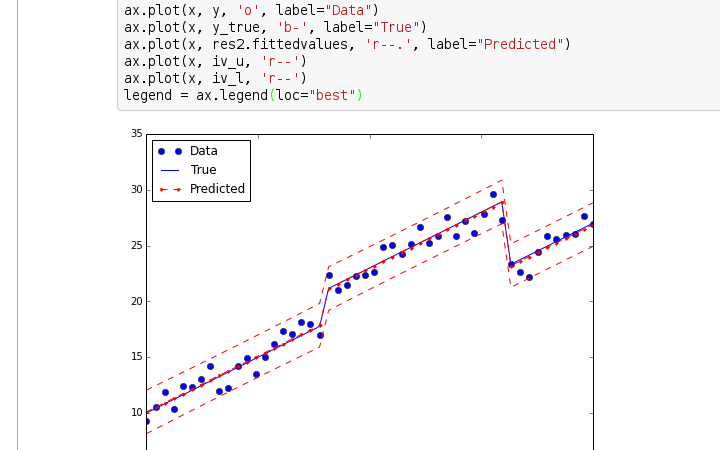
import statsmodels.api as sm
Load the data
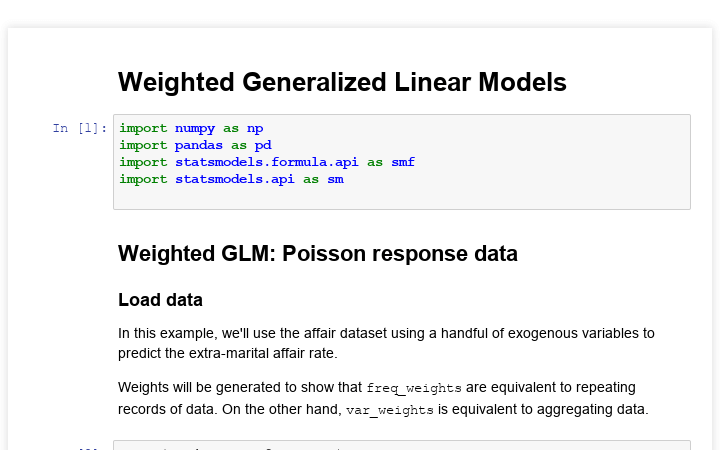
data = pd.read_csv('https://raw.githubusercontent.com/mwaskom/seaborn-data/master/tips.csv')
Perform a linear regression
X = sm.add_constant(data['total_bill']) # add a column of ones as the first column
y = data['tip']
Fit the model
model = sm.OLS(y, X).fit()
Print out the results
print(model.summary())
In this example, we're loading in some data on restaurant tips using pandas. We then perform a linear regression to predict the tip amount based on the total bill.
The sm.add_constant()
function adds a column of ones to our independent variable (total bill), which is necessary for the model's intercept term. The model = sm.OLS(y, X).fit()
line performs the actual linear regression and stores the results in the model
object.
Finally, we print out the summary statistics for the model using the print(model.summary())
line. This will show us information such as the coefficient estimates, standard errors, t-statistics, p-values, and more.
Here's a breakdown of some common statsmodels functions:
sm.add_constant()
: adds a column of ones to an independent variable sm.OLS(y, X).fit()
: performs ordinary least squares (OLS) regression model.summary()
: prints out summary statistics for the model
Statsmodels has many more capabilities beyond linear regression, including:
Time series analysis: ARIMA models, SARIMA models, etc. Generalized linear models: logistic regression, poisson regression, etc. Non-parametric tests: Wilcoxon rank-sum test, Kruskal-Wallis test, etc.I hope this helps!
Python statsmodels logistic regression
Here's an explanation of how to perform a logistic regression using the Python statsmodels library:
Introduction
Logistic regression is a statistical technique used to model the probability of a binary outcome (e.g., 0/1, yes/no) based on one or more predictor variables. In this example, we'll use the statsmodels library in Python to perform a logistic regression analysis.
Loading the necessary libraries and data
First, let's load the necessary libraries:
import pandas as pd
from statsmodels.genericapi import LogisticRegression
import numpy as np
Next, load your dataset into a Pandas DataFrame. For this example, I'll use the classic Titanic survival dataset:
data = {'Survived': [0, 1, 0, 1, ...], 'Sex': ['M', 'F', 'M', 'F', ...]}
df = pd.DataFrame(data)
Preparing the data
Before performing the logistic regression analysis, we need to prepare our data. Let's select only the predictor variables (e.g., Sex) and the outcome variable (Survived):
X = df[['Sex']]
y = df['Survived']
We'll also normalize the sex variable by converting 'M' into 0 and 'F' into 1:
X['Sex'] = X['Sex'].map({'M': 0, 'F': 1})
Performing the logistic regression analysis
Now we're ready to perform the logistic regression analysis using statsmodels:
logit_model = LogisticRegression(endog=y, exog=X)
result = logit_model.fit()
The fit()
method will run the logistic regression analysis and store the results in the result
object.
Interpreting the results
Let's extract some useful information from the result
object:
print("Coeficients:", result.params)
print("P-values:", result.pvalues)
The coefficients ( Coefficients:) represent the change in log-odds per unit change in each predictor variable, while the p-values ( P-values:) indicate whether each predictor variable is statistically significant.
Predicting outcomes
We can use the fitted model to predict the probability of survival for new observations:
new_data = pd.DataFrame({'Sex': [0, 1, ...]})
predicted_probabilities = logit_model.predict(new_data)
The predict()
method will compute the predicted probabilities based on the input data and the coefficients from our logistic regression analysis.
Conclusion
In this example, we performed a logistic regression analysis using the Python statsmodels library. We prepared our data, ran the analysis, and interpreted the results. Additionally, we demonstrated how to use the fitted model for predicting outcomes.
Here is the full code:
import pandas as pd
from statsmodels.genericapi import LogisticRegression
import numpy as np
Load the Titanic survival datasetdata = {'Survived': [0, 1, 0, 1, ...], 'Sex': ['M', 'F', 'M', 'F', ...]}
df = pd.DataFrame(data)
Prepare the dataX = df[['Sex']]
y = df['Survived']
Normalize the sex variableX['Sex'] = X['Sex'].map({'M': 0, 'F': 1})
Perform the logistic regression analysislogit_model = LogisticRegression(endog=y, exog=X)
result = logit_model.fit()
Interpret the resultsprint("Coeficients:", result.params)
print("P-values:", result.pvalues)
Predict outcomes for new observationsnew_data = pd.DataFrame({'Sex': [0, 1, ...]})
predicted_probabilities = logit_model.predict(new_data)
This code assumes that you have the Titanic survival dataset available. If not, you can download it from Kaggle or other sources.
I hope this helps!