Python runtime aws lambda example
Python runtime aws lambda example
Here is an example of a Python runtime AWS Lambda function:
lambda_function.py
import boto3
import json
dynamodb = boto3.resource('dynamodb')
table_name = 'my-table'
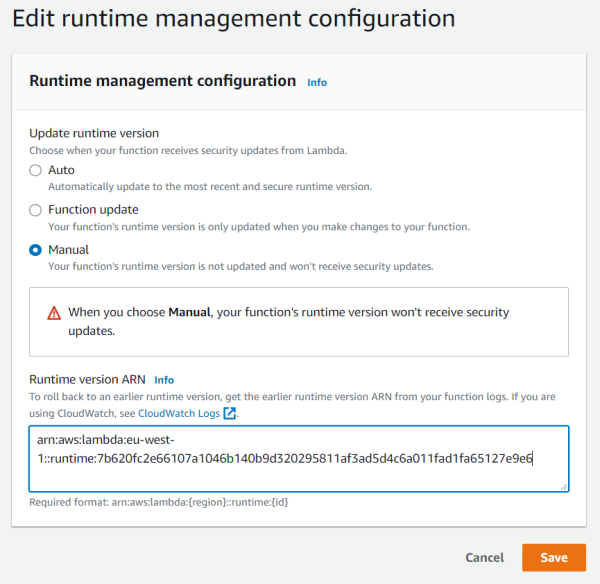
def lambda_handler(event, context):
Get the table from DynamoDB
table = dynamodb.Table(table_name)
Process the event
if event['httpMethod'] == 'POST':
Create a new item in the table
data = json.loads(event['body'])
table.put_item(Item=data)
return {
'statusCode': 201,
'body': json.dumps({'message': 'Item created successfully'})
}
elif event['httpMethod'] == 'GET':
Read an item from the table
key = {'id': event['pathParameters']['id']}
item = table.get_item(Key=key).get('Item')
if item:
return {
'statusCode': 200,
'body': json.dumps({'data': item})
}
else:
return {
'statusCode': 404,
'body': json.dumps({'message': 'Item not found'})
}
elif event['httpMethod'] == 'PUT':
Update an item in the table
data = json.loads(event['body'])
key = {'id': event['pathParameters']['id']}
table.update_item(Key=key, UpdateExpression='set #data = :val', ExpressionAttributeNames={'#data': 'data'}, ExpressionAttributeValues={':val': data})
return {
'statusCode': 200,
'body': json.dumps({'message': 'Item updated successfully'})
}
elif event['httpMethod'] == 'DELETE':
Delete an item from the table
key = {'id': event['pathParameters']['id']}
table.delete_item(Key=key)
return {
'statusCode': 204,
'body': json.dumps({'message': 'Item deleted successfully'})
}
If none of the above handlers match, return a 404 error
return {
'statusCode': 404,
'body': json.dumps({'message': 'Not Found'})
}
How it works
This Lambda function is triggered by an API Gateway. It uses the AWS SDK for Python (Boto3) to interact with a DynamoDB table.
The function has four handlers: POST
, GET
, PUT
, and DELETE
. Each handler processes the incoming event and returns a response.
POST
: Creates a new item in the DynamoDB table using the put_item
method. The request body is expected to be JSON-formatted data. GET
: Reads an item from the DynamoDB table using the get_item
method. The path parameter id
is used to identify the item to read. PUT
: Updates an existing item in the DynamoDB table using the update_item
method. The request body is expected to be JSON-formatted data, and the path parameter id
is used to identify the item to update. DELETE
: Deletes an item from the DynamoDB table using the delete_item
method. The path parameter id
is used to identify the item to delete.
If none of these handlers match, the function returns a 404 error.
AWS Lambda settings
To deploy this Lambda function, you'll need to set up the following:
Handler: Set the handler tolambda_function.handler
. Runtime: Choose Python as the runtime. Timeout: Set the timeout to a reasonable value (e.g., 300 seconds). Memory size: Set the memory size to a reasonable value (e.g., 128 MB).
API Gateway settings
To integrate this Lambda function with an API Gateway, you'll need to set up the following:
REST API: Choose REST as the API type. Integration request: SelectLambda function
and enter the ARN of your Lambda function. Method request: Set the method to POST
, GET
, PUT
, or DELETE
depending on which handler you want to trigger. URI path: Enter the path parameter(s) required by your Lambda function (e.g., /items/{id}
).
This is just one example of a Python runtime AWS Lambda function. The specifics will vary depending on your use case and requirements.
How do I change the runtime in Python Lambda?
I'd be happy to help! However, please note that you're asking about changing the runtime of a Lambda function in Python, which is not a straightforward process.
Before we dive into the details, let me clarify that Lambda functions are a type of serverless computing that runs on AWS. They're designed to be lightweight and efficient, with a focus on cost-effectiveness.
By default, Lambda functions run on the nodejs14.x
or python3.8
runtime, depending on the language you choose. However, if you need to use a different Python version or runtime, you can't simply change it in your code; instead, you'll need to create a new Lambda function with the desired runtime.
Here's how you can do this:
Create a new Lambda function: Go to the AWS Management Console and navigate to the Lambda dashboard. Click "Create function" and choose "Author from scratch". Choose Python as your runtime. Choose the correct Python version: In the "Runtime" dropdown menu, select the desired Python version (e.g.,python3.9
or python3.10
). Note that not all versions are available for all Lambda functions; some may only support older Python versions. Configure your function's handler and role: Set up your Lambda function as you normally would, including specifying the handler, role, and environment variables. Deploy your new function: Once you've configured everything, deploy your new Lambda function to AWS.
Now, here's a crucial thing to keep in mind: when you create a new Lambda function with a different runtime than your existing one, you'll need to update any references to that old function. This might include updating API Gateway integrations, SNS subscriptions, or SQS queues that are connected to your original Lambda function.
To illustrate this, let's say you have an AWS API Gateway that integrates with a Python 3.8 Lambda function (old runtime). If you decide to create a new Lambda function using Python 3.9 (new runtime), you'll need to update the API Gateway integration to point to the new function. This involves updating the "Integration request" and "Integration response" settings in your API Gateway.
In summary, while it's not possible to change the runtime of an existing Lambda function, you can create a new one with the desired Python version or runtime by following these steps. Just remember to update any dependencies on the original function when you do so.