Python fastapi vs flask reddit
Python fastapi vs flask reddit
I'd be happy to provide a comparison between FastAPI and Flask on Reddit.
Reddit Thread: "FastAPI vs Flask - Which Framework is Best for My Next Project?"
Original Post:
Hey fellow developers! I'm planning my next project and I'm torn between using FastAPI and Flask. Has anyone had experience with both? Can you give me some pros and cons of each?
Reply 1: "FastAPI All the Way!"
I've used both frameworks, and while Flask is great for small projects, I think FastAPI is a much better choice for larger-scale applications. Here's why:
Speed: FastAPI is significantly faster than Flask, thanks to its async-friendly design and support for HTTP/2. Automatic JSON encoding: No more manually converting your data to JSON - FastAPI does it for you! Better error handling: FastAPI provides detailed error messages with request information, making debugging a breeze. Support for modern Python features: FastAPI takes advantage of Python 3.7+ features like async/await and type hints, giving you more power and flexibility.Flask is great for small, simple projects or prototyping, but if you're building something complex or needing performance optimizations, I think FastAPI is the way to go.
Reply 2: "Not So Fast... (pun intended)"
I agree that FastAPI has some nice features, but I wouldn't write off Flask just yet. Here's why:
Ease of use: Flask has a much simpler learning curve than FastAPI - if you're new to Python or web development, Flask is a great place to start. Flexibility: Flask lets you create routes dynamically using regular expressions, which can be super helpful for certain types of projects. Extensive libraries and communities: Flask has been around longer, so there are more resources available - if you need help or want to integrate with other libraries, Flask is a better bet.That being said, if you're looking for performance optimizations and don't mind learning some new concepts (like async/await), FastAPI might be the way to go. It's all about your project's specific needs!
Reply 3: "The Verdict?"
Thanks for the replies! After considering both sides, I think I'll choose FastAPI for my next project. The speed and error handling features are too valuable to pass up.
That being said, if you're new to Python or web development, Flask might still be a great choice - it's not necessarily an either/or decision. Both frameworks have their strengths and weaknesses; it's all about your specific needs and goals.
Hope this helps someone else out there!
Python FastAPI tutorial
Here's a step-by-step guide to building a simple web application using Python and the FastAPI framework.
What is FastAPI?
FastAPI is a modern, fast (very high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints.
Why use FastAPI?
FastAPI offers several advantages over other Python web frameworks:
Speed: FastAPI is extremely fast because it uses ASGI (Asynchronous Server Gateway Interface) which allows it to run concurrently with other tasks. Ease of Use: FastAPI provides an easy-to-use API that's similar to Django's views, making it simple for developers to create RESTful APIs. Strong Type Hinting: FastAPI supports Python 3.7+ type hinting, which means you can specify the types of your function arguments and return values, giving you better code completion and type checking. Automatic JSON Generation: FastAPI automatically generates JSON responses for GET requests, making it easy to create RESTful APIs.Creating a Simple Web App
Let's create a simple web app that responds to two endpoints: /hello
and /goodbye
.
First, install FastAPI using pip:
pip install fastapi
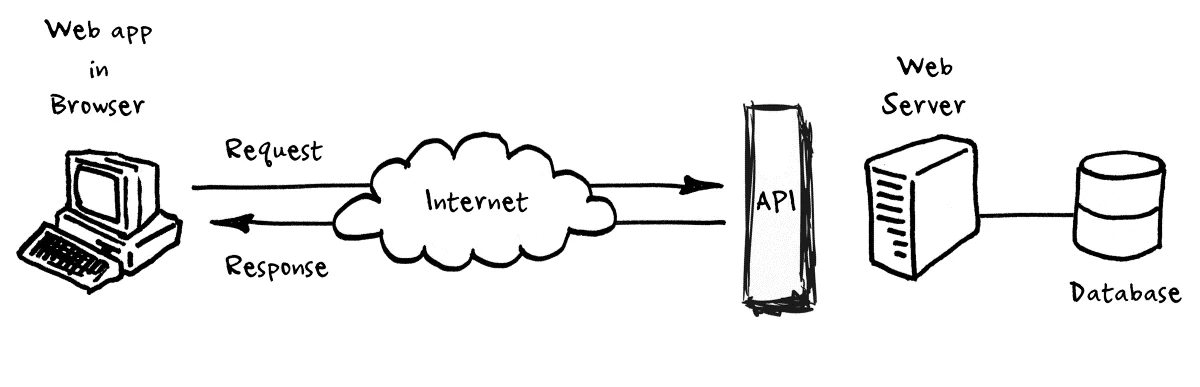
Create Your App
Next, create a new Python file (e.g., main.py
) and import FastAPI:
from fastapi import FastAPI
app = FastAPI()
Define Endpoints
Now, define two endpoints: /hello
and /goodbye
. The endpoint for /hello
will return the string "Hello, World!", while the endpoint for /goodbye
will return the string "Goodbye!":
@app.get("/hello")
async def hello_world():
return {"message": "Hello, World!"}
@app.get("/goodbye")
async def goodbye_world():
return {"message": "Goodbye!"}
Run Your App
To run your app, use the following command:
uvicorn main:app --host 0.0.0.0 --port 8000
Test Your App
Open a web browser and navigate to http://localhost:8000/hello
or http://localhost:8000/goodbye
to test your app.
Conclusion
In this tutorial, we've created a simple web application using FastAPI, Python, and ASGI. We've defined two endpoints that return JSON responses for GET requests. This is just the beginning of what you can do with FastAPI; it has many more features and capabilities waiting for you to explore!
Let me know if you have any questions or need further clarification!