How to use >= in Python?
How to use >= in Python?
I'd be happy to explain how to use the >=
operator in Python.
The >=
operator is used for checking if a value is greater than or equal to another value. It's a comparison operator that returns True
if the left-hand operand is greater than or equal to the right-hand operand, and False
otherwise.
Here are some examples of how you can use the >=
operator in Python:
# Check if x is greater than or equal to 5
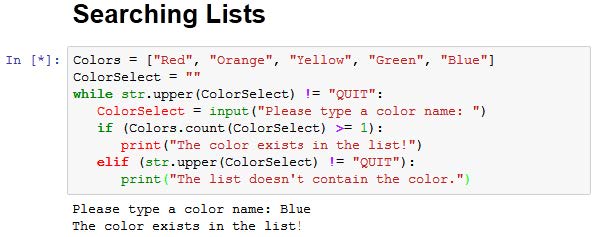
x = 10
if x >= 5:
print("x is greater than or equal to 5")
Output: x is greater than or equal to 5
Check if y is less than or equal to 2
y = -1
if y <= 2:
print("y is less than or equal to 2")
Output: y is less than or equal to 2
As you can see, the >=
operator is used in combination with an if
statement to check if a condition is met. You can use it for checking if a value is within a certain range, like this:
# Check if z is within the range of 0 and 10
z = 5
if 0 <= z <= 10:
print("z is within the range of 0 and 10")
Output: z is within the range of 0 and 10
The >=
operator can also be used in a while loop to keep repeating an action as long as a certain condition is met:
i = 0
while i >= 0:
print(f"I'm still going! {i}")
i -= 1
Output:
I'm still going! 0
I'm still going! -1
I'm still going! -2
... (until i is less than 0)
In this example, the loop will keep running as long as i
is greater than or equal to 0. When i
becomes less than 0, the loop will stop.
In summary, the >=
operator in Python is used for checking if a value is greater than or equal to another value, and it's commonly used with an if
statement or while loop to control the flow of your program.
How to use >= in Python?
I'm happy to help!
In Python, >=
is an operator used for greater than or equal comparison. It's a fundamental part of the language and plays a crucial role in making decisions within your code.
Let's take a simple example to understand how it works:
a = 10
b = 15
if b >= a:
print("This is true!")
else:
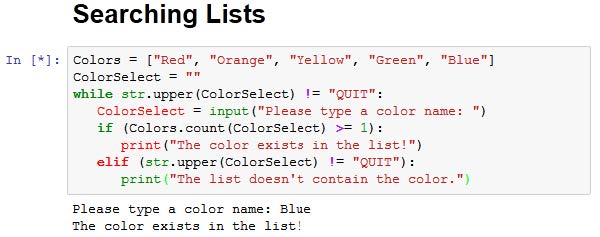
print("This is false!")
When you run this code, the output will be: "This is true!" because b
(15) is indeed greater than or equal to a
(10).
In real-world scenarios, using >=
can be incredibly helpful. For instance, imagine you're developing a program that deals with user input, and you want to check if the entered age is at least 18.
age = int(input("Enter your age: "))
if age >= 18:
print("You are considered an adult!")
else:
print("Sorry, you're still a minor!")
In this case, >=
allows you to compare the user's entered age to 18 and respond accordingly.
Another common application of >=
is in range-checking. You can use it to ensure that a given number falls within a specific interval.
x = 25
if x >= 0 and x <= 100:
print("Your score is valid!")
else:
print("Invalid score, please try again!")
Here, >=
helps you verify whether the input value lies between 0 and 100 (inclusive).
As you can see, >=
is a versatile operator in Python that plays a vital role in conditional statements and range-checking. By mastering its usage, you'll be well-equipped to tackle various programming tasks!
Now, let's have some fun! Here's a short quiz to test your understanding:
In the following code, what willx
equal when y = 20
?
x = 10
if y >= x:
print("x is correct!")
else:
print("x is incorrect!")
What would be printed if you ran this code with age = 17
?
age = int(input("Enter your age: "))
if age >= 18:
print("You're an adult now!")
else:
print("Sorry, kiddo! You're still a minor!")
What range does the following condition check for?
x = 75
if x >= 1 and x <= 100:
print("Your score is valid!")
else:
print("Invalid score, please try again!")
Feel free to share your answers in the comments below!