What are the different string pattern matching functions in Python?
What are the different string pattern matching functions in Python?
In Python, there are several string pattern matching functions that can help you search and manipulate strings based on specific patterns. Here are some of the most commonly used ones:
str.find(): This function searches for a specified substring within a given string and returns the index where the first occurrence of the substring is found. If the substring is not found, it returns -1.Example: s = "Hello World"; print(s.find("World"))
find()
, this function also searches for a specified substring within a given string and returns the index where the first occurrence of the substring is found. However, if the substring is not found, it raises a ValueError instead of returning -1.
Example: s = "Hello World"; print(s.index("World"))
Example: s = "Hello World World"; print(s.count("World"))
re
that provides support for regular expressions. Regular expressions are patterns used to match character combinations in strings. The re
module offers several functions, including: re.search(pattern, string)
: Returns the first occurrence of the pattern in the string. re.match(pattern, string)
: Returns the first occurrence of the pattern at the beginning of the string. re.findall(pattern, string)
: Finds all occurrences of the pattern in the string and returns them as a list.
Example: import re; s = "Hello World"; print(re.search("World", s).group())
Example: name = "John"; print("Hello, {}!".format(name))
Example: name = "John"; print(f"Hello, {name}!")
Example: s = "Hello World"; print(s.replace("World", "Earth"))
These are some of the most commonly used string pattern matching functions in Python. They provide a range of ways to search, manipulate, and create strings based on specific patterns.
Python re module w3schools example
Here is the rewritten text in English:
The re
module in Python provides support for regular expressions (regex). Regular expressions are a way to describe patterns in strings, and can be used for tasks such as searching, validating, and extracting data from strings.
Here's an example from W3Schools that demonstrates how to use the re
module:
import re
Pattern
pattern = r"hellos+world"
String to search
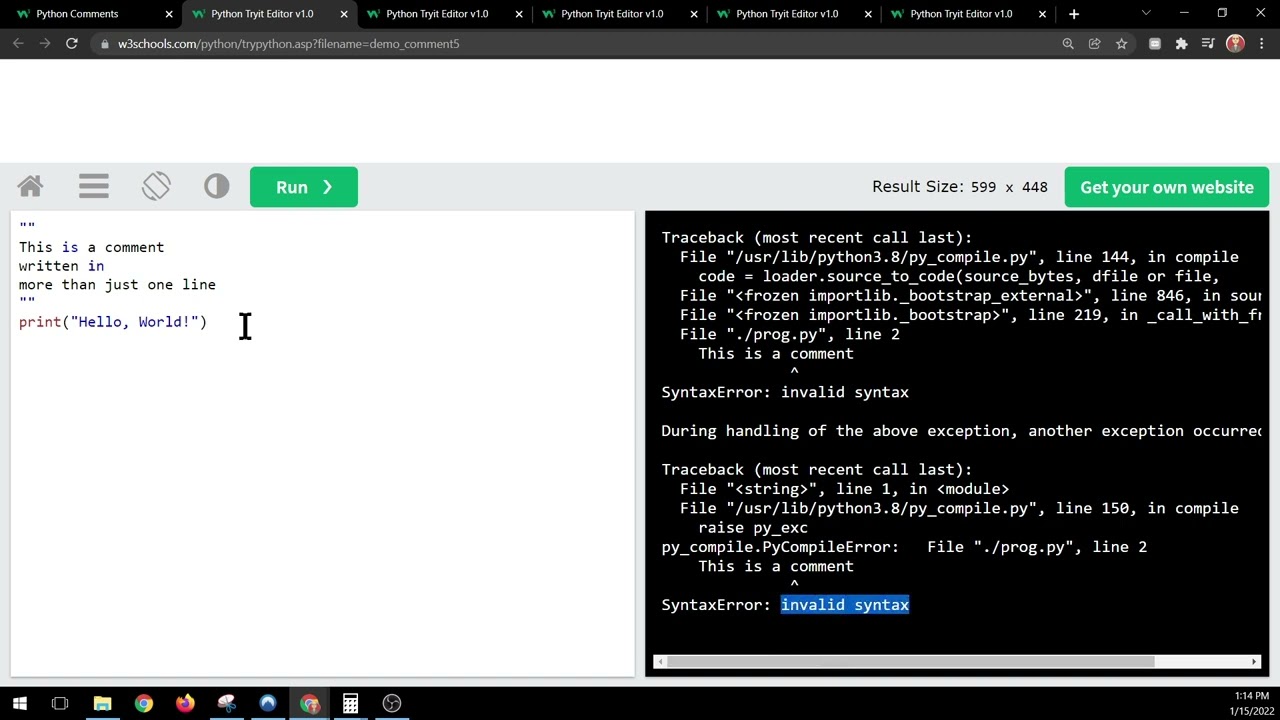
text = "hello world"
Search for the pattern in the text
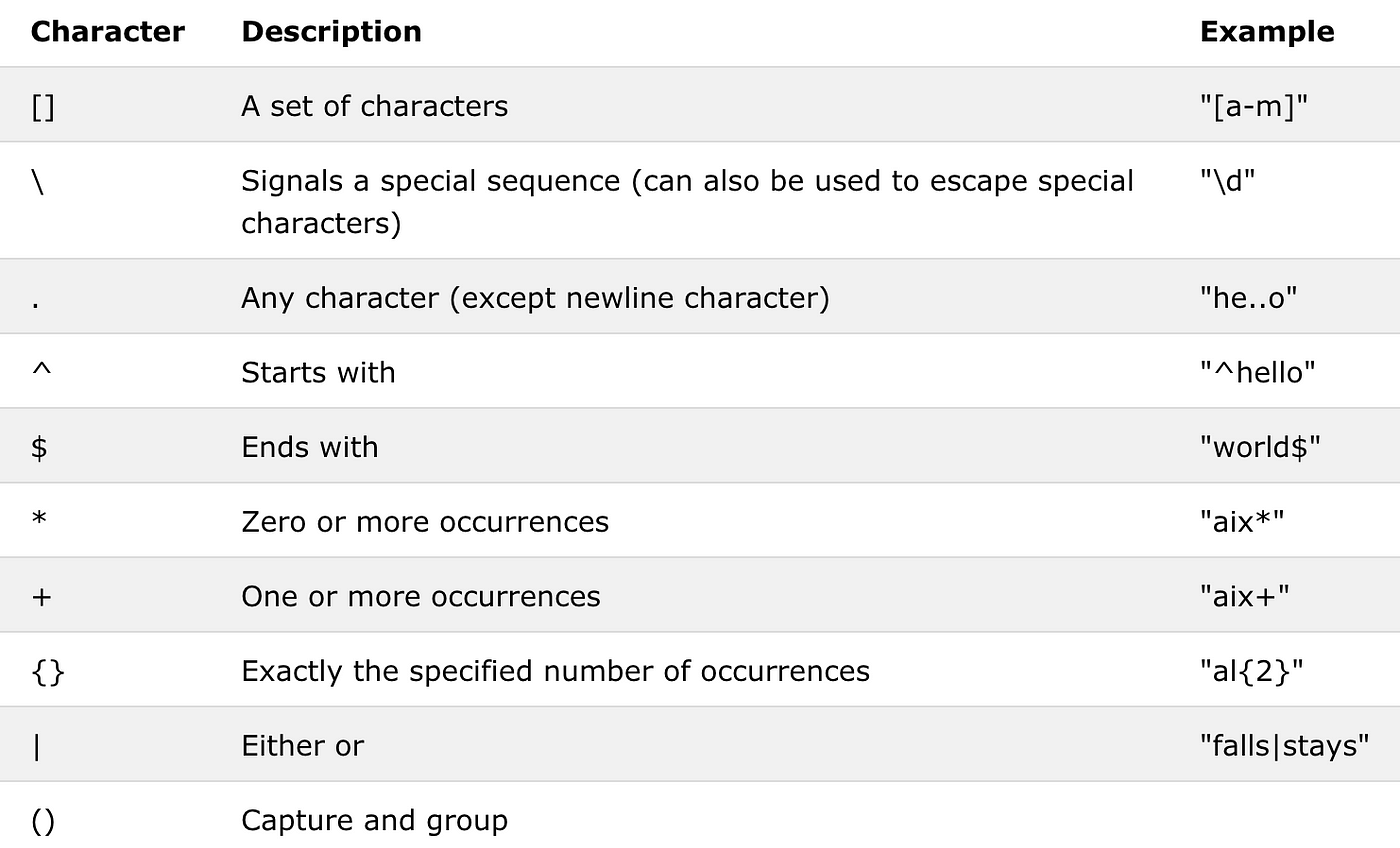
match = re.search(pattern, text)
if match:
print("Match found: ", match.group())
else:
print("No match")
In this example:
We import there
module. We define a regular expression pattern (r"hellos+world"
). This pattern matches the string "hello followed by one or more whitespace characters and then world". We create a text variable with the value "hello world", which contains the text we want to search for the pattern. We use the re.search()
function to find all non-overlapping matches of the pattern in the text. The search()
method scans through the text looking for a match, and returns a match object if it finds one.
If you run this code, the output will be:
Match found: hello world
This demonstrates how to use regular expressions with the re
module in Python.
The regular expression pattern (r"hellos+world"
):
"hello"
matches the literal string "hello". s+
matches one or more whitespace characters (spaces, tabs, etc.). "world"
matches the literal string "world".
In this example, the regular expression is used to search for a specific pattern in a text string. The re
module provides several methods for working with regular expressions, including search()
, match()
, and findall()
.
Here's a breakdown of the different parts of the code:
import re
: This line imports the re
(regular expression) module. pattern = r"hellos+world"
: This line defines the regular expression pattern that we want to search for in our text. The r
prefix means this is a raw string, and won't be interpreted as a Python string. text = "hello world"
: This line creates a text variable with the value "hello world". This is the text that we'll use to test our pattern. match = re.search(pattern, text)
: This line searches for our regular expression pattern in our text. If it finds a match, it returns a match object; otherwise, it returns None
. if match: ... else: ...
: These lines check whether the re.search()
method found a match or not.
If you're interested in learning more about regular expressions and how to use them with Python's re
module, I'd be happy to help!