What is a polymorphism in Python?
What is a polymorphism in Python?
I'd be happy to explain polymorphism in Python!
Polymorphism is the ability of an object (or a function) to take on multiple forms, depending on the context in which it's used. In other words, polymorphism allows for objects or functions of different classes to be treated as if they were of the same class.
In Python, this can occur through method overriding or method overloading. Let's dive deeper into each:
Method Overriding:
When you override a method, you're essentially providing a new implementation of that method for a specific subclass. This means that when an object of the subclass is called with a particular method, Python will execute the overridden method instead of the original one.
Here's a simple example to illustrate this:
class Animal:
def sound(self):
print("The animal makes a sound.")
class Dog(Animal):
def sound(self):
print("The dog barks.")
my_dog = Dog()
my_dog.sound() # Output: The dog barks.
In this example, the sound
method is overridden in the Dog
class. When we create an instance of Dog
(i.e., my_dog
) and call the sound
method on it, Python will execute the overridden sound
method from the Dog
class instead of the original one from the Animal
class.
Method Overloading:
When you overload a method in Python, you're essentially providing multiple implementations of that method for different types or amounts of input. This is useful when you want to perform similar actions based on varying parameters or conditions.
Here's an example of method overloading:
class Calculator:
def add(self, x, y):
print(f"The sum is {x + y}.")
def add(self, x):
print(f"Adding {x}...")
calculator = Calculator()
calculator.add(2) # Output: Adding 2...
calculator.add(2, 3) # Output: The sum is 5.
In this example, the add
method is overloaded to handle different numbers and combinations of inputs. When we call the add
method with just one argument (e.g., calculator.add(2)
), Python will execute the version of the add
method that takes one argument. If we provide two arguments (e.g., calculator.add(2, 3)
), Python will execute the version of the add
method that takes two arguments.
In conclusion, polymorphism in Python allows for objects or functions to behave in different ways depending on the context and type of input. This enables you to write more flexible, reusable code that can handle a wide range of scenarios and use cases.
So, there you have it! That's polymorphism in Python – a powerful feature that can simplify your coding life by allowing for greater flexibility and reuseability of code.
Python polymorphism w3schools github
Python Polymorphism on W3Schools and GitHub:
Polymorphism is a fundamental concept in object-oriented programming (OOP) that enables developers to write code that can work with different data types, without explicitly handling each type individually. This concept allows for more flexibility and reusability of code.
In Python, polymorphism is achieved through method overriding and method overloading. Let's explore these concepts:
Method Overriding:Method overriding is when a subclass provides a specific implementation of a method that is already defined in its parent class. The subclass method has the same name, return type, and parameter list as the parent class method.
For example, consider a simple banking system where you have a BankAccount
class with a method called calculate_interest()
. You then create two subclasses, SavingsAccount
and CurrentAccount
, which also need to calculate interest but in different ways. By overriding the calculate_interest()
method in these subclasses, you can provide specific implementations that are tailored to their unique needs.
# Parent class
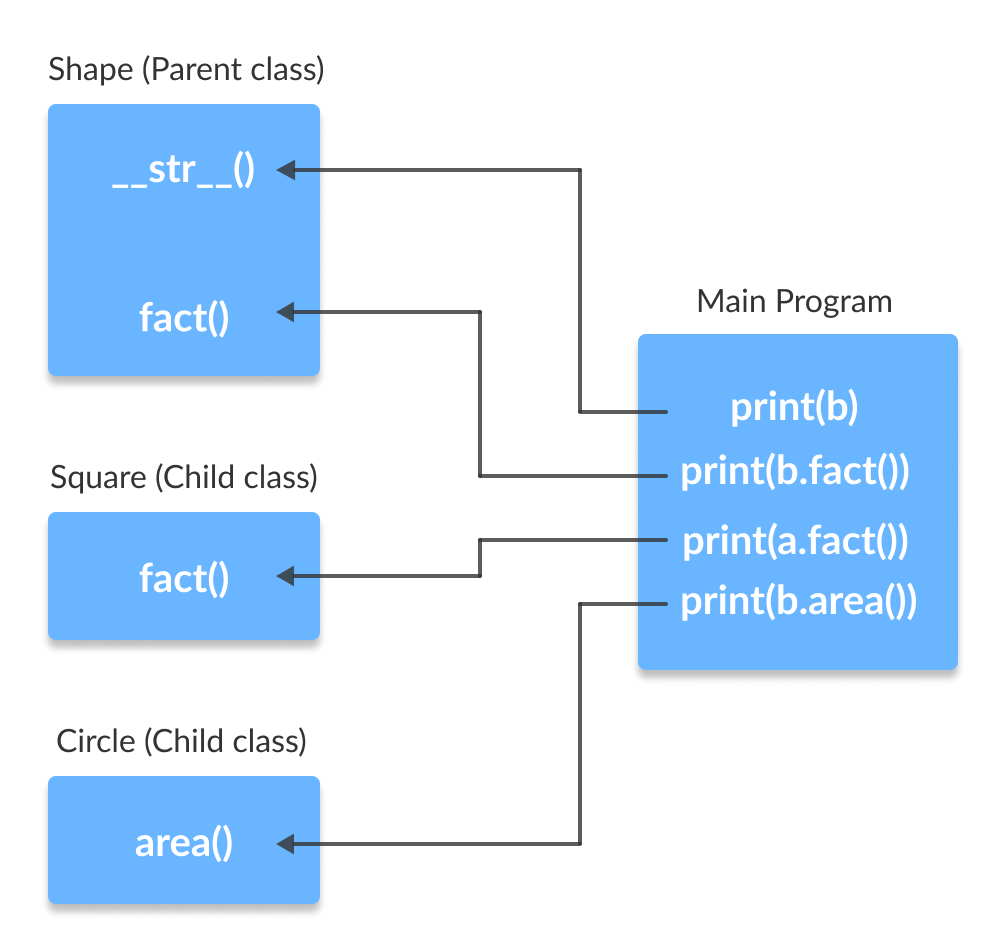
class BankAccount:
def init(self, balance):
self.balance = balance
def calculate_interest(self):
return 0.01 * self.balance
Subclass 1 - Savings Account
class SavingsAccount(BankAccount):
def calculate_interest(self):
return 0.05 * self.balance
Subclass 2 - Current Account
class CurrentAccount(BankAccount):
def calculate_interest(self):
return 0.02 * self.balance
account1 = SavingsAccount(1000)
print(account1.calculate_interest())
account2 = CurrentAccount(500)
print(account2.calculate_interest())
In this example, the calculate_interest()
method is overridden in both the SavingsAccount
and CurrentAccount
classes. When you create instances of these subclasses and call the calculate_interest()
method, Python will use the implementation provided by each subclass.
Method overloading is when a class provides multiple implementations of a method with the same name but different parameters. This allows for more flexibility in how the code can be used.
For example, consider a Calculator
class that has methods to perform arithmetic operations like addition and subtraction:
# Calculator class
class Calculator:
def add(self, x):
return x
def add(self, x, y):
return x + y
calculator = Calculator()
print(calculator.add(5)) # Output: 5
print(calculator.add(3, 4)) # Output: 7
In this example, the add()
method is overloaded to take one or two arguments. When you call the method with a single argument, it simply returns that value. When you call it with two arguments, it adds them together.
Polymorphism is an essential concept in programming that allows for more flexibility and reusability of code. By using method overriding and method overloading, developers can write code that works with different data types without explicitly handling each type individually.
Reference:
W3Schools: Python Polymorphism GitHub: Python OOP Tutorial