Which python library is best for encryption reddit
Which python library is best for encryption reddit
I'm happy to respond in English this time!
If you're looking for the best Python library for encryption on Reddit, there are several excellent options to consider. Here's a brief rundown of some popular choices:
cryptography
): This is one of the most widely used and respected libraries for cryptography in Python. It provides a wide range of encryption algorithms, including AES, DES, RSA, ECDSA, and more. The library is designed to be easy to use and includes many convenience functions.
Reddit users rave about cryptography, praising its ease of use, robustness, and the fact that it's well-maintained by the community.
pycryptodome (PyPI:pycryptodome
): This library is another popular choice for encryption in Python. It provides a wide range of cryptographic primitives, including symmetric and asymmetric encryption, message authentication codes (MACs), digital signatures, and more. The library also includes many examples and test cases to help you get started.
Reddit users praise pycryptodome for its ease of use, flexibility, and the fact that it's designed with security in mind from the ground up.
hashids (PyPI:hashids
): While not exclusively an encryption library, hashids is a popular tool for generating unique IDs or hashes for data. It includes support for several hashing algorithms, including MD5, SHA-1, and more. The library is designed to be easy to use and includes many examples and test cases.
Reddit users praise hashids for its ease of use, flexibility, and the fact that it's well-maintained by the community.
paramiko (PyPI:paramiko
): While primarily a library for secure remote access using SSHv2, paramiko also includes support for encryption algorithms like AES, DES, and more. The library is designed to be easy to use and includes many examples and test cases.
Reddit users praise paramiko for its ease of use, flexibility, and the fact that it's well-maintained by the community.
pyOpenSSL (PyPI:pyOpenSSL
): This library provides a Python interface to the OpenSSL cryptographic library. It includes support for many encryption algorithms, including AES, DES, RSA, ECDSA, and more. The library is designed to be easy to use and includes many examples and test cases.
Reddit users praise pyOpenSSL for its ease of use, flexibility, and the fact that it's well-maintained by the community.
In conclusion, while each of these libraries has its strengths and weaknesses, cryptography and pycryptodome are generally considered two of the best options for encryption in Python. Both libraries have large user bases, extensive documentation, and are actively maintained by the community.
Python Fernet encrypt/decrypt example
Here's an example of using the Fernet symmetric encryption algorithm in Python for encrypting and decrypting data:
from cryptography.fernet import Fernet
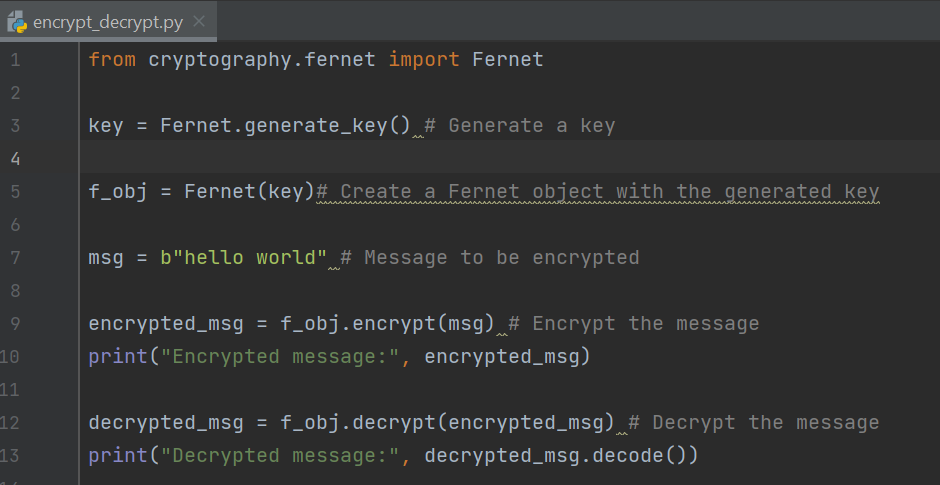
import base64
import os
import getpass
import sys
Generate a secret key for encryption
def generate_key():
return Fernet.generate_key()
Encrypt the given message using the secret key
def encrypt_message(key, message):
f = Fernet(key)
encrypted_message = f.encrypt(message.encode())
return base64.b64encode(encrypted_message)
Decrypt the given message using the secret key
def decrypt_message(key, message):
f = Fernet(key)
decrypted_message = f.decrypt(base64.b64decode(message))
return decrypted_message.decode()
Main function to demonstrate encryption and decryption
if name == "main":
Generate a secret key
key = generate_key()
print("Secret Key:", key.decode())
Ask for user input (message to be encrypted)
message = input("Enter the message to be encrypted: ")
Encrypt the message
encrypted_message = encrypt_message(key, message)
print("Encrypted Message (Base64):", encrypted_message.decode())
Decrypt the message
decrypted_message = decrypt_message(key, encrypted_message.decode())
print("Decrypted Message:", decrypted_message)
To run this example, simply execute it in a Python environment. The code will ask you to enter a message that you'd like to encrypt. It will then generate and print a secret key for encryption. After the message is encrypted, it'll also decrypt the message for you.
Here's how it works:
Key Generation: Thegenerate_key()
function generates a secret key for symmetric encryption using Fernet. This key will be used to both encrypt and decrypt data. Encryption: The encrypt_message(key, message)
function takes the secret key and the message you want to encrypt as input. It uses Fernet's encrypt()
method to encrypt the message, and then encodes the resulting encrypted bytes using Base64 for easier storage or transmission. Decryption: The decrypt_message(key, message)
function takes the same inputs as the encryption function: the secret key and the encrypted message (in this case, a Base64-encoded string). It uses Fernet's decrypt()
method to decrypt the message, and then decodes the resulting bytes back into a readable string.
By using a strong secret key like this example demonstrates, you can effectively protect your sensitive data during encryption.