How to access a 2D list in Python
How to access a 2D list in Python
I'm happy to help you with that!
In Python, accessing elements in a 2D list (also known as a matrix or a list of lists) is a crucial task. A 2D list is a collection of lists, where each sublist contains elements of the same data type. For example:
my_2d_list = [
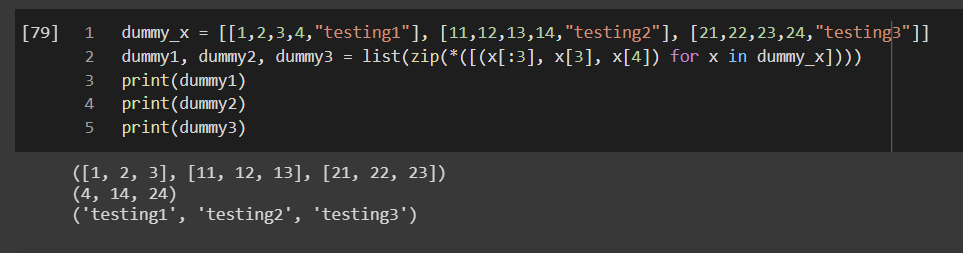
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
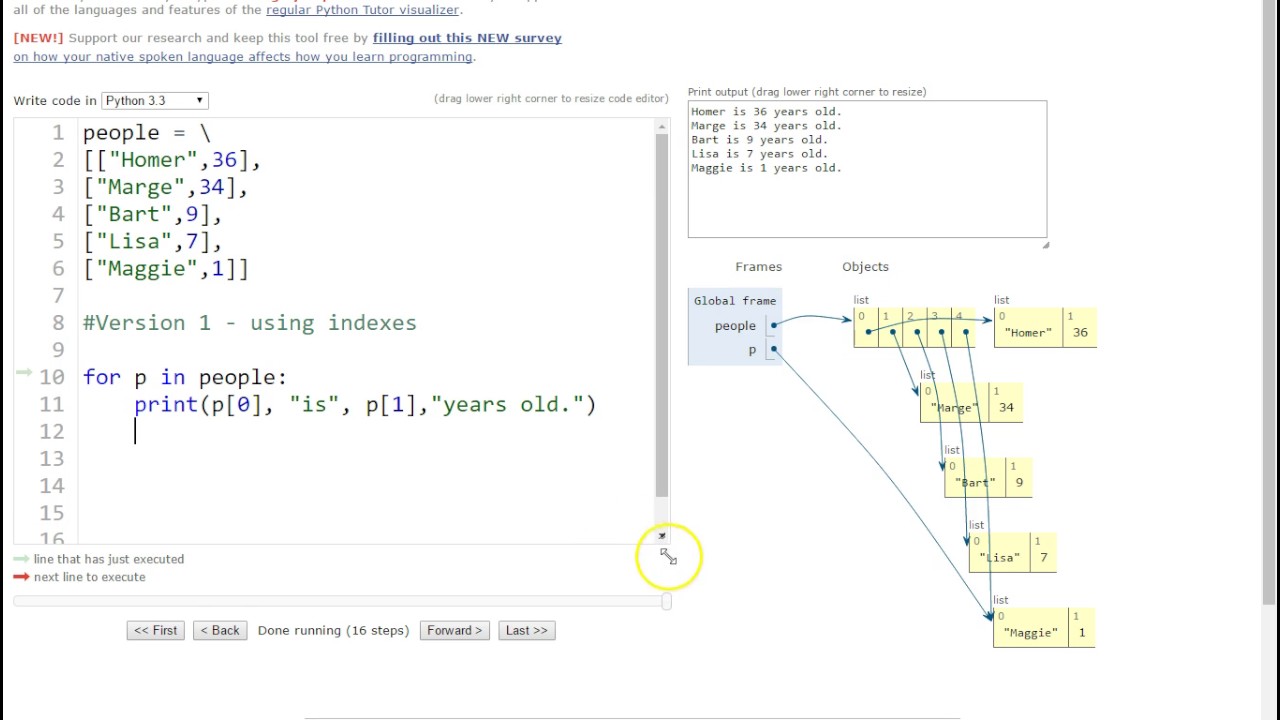
]
To access an element in a 2D list, you need to specify the row and column indices. The syntax is my_2d_list[row_index][column_index]
.
Here's how it works:
Row index: Specify the index of the sublist that contains the desired element. In Python, list indices start from 0, so if your 2D list has three sublists (rows), the row indices would be 0, 1, and 2.For example, to access the first row:
my_2d_list[0] # returns [1, 2, 3]
Column index: Specify the index of the element within a sublist (row). Again, list indices start from 0. So, if your sublists have three elements (columns), the column indices would be 0, 1, and 2.
For example, to access the second element in the first row:
my_2d_list[0][1] # returns 2
Here's a step-by-step guide:
Accessing a specific element:
Identify the row and column indices of the desired element. Use square brackets[]
to access the sublist (row) at that index. Inside those brackets, add another set of square brackets to specify the column index.
Example:
my_2d_list[1][2] # returns 6
Iterating over a 2D list:
You can use nested loops or list comprehensions to iterate over a 2D list. Here are some examples:
Nested Loops:
for row in my_2d_list:
for element in row:
print(element)
List Comprehension:
[element for sublist in my_2d_list for element in sublist]
These examples demonstrate how to access and iterate over elements in a 2D list. I hope this helps!
Python 2D list slice column
I'm happy to help with your query! Here's a detailed explanation of slicing columns in a 2D list using Python:
Python lists are dynamic arrays, which means they can grow or shrink as elements are added or removed. When you create a 2D list (also known as a matrix), you have the flexibility to slice it into sub-lists based on certain criteria.
A slice is created by specifying start and stop indices for the row and column. The syntax is similar to that of strings:
list_name[start_row:stop_row, start_col:stop_col]
Here's an example using a 2D list matrix
with values from 0 to 9:
import numpy as np Create a 3x4 matrix filled with numbers from 0 to 9
matrix = np.array([[i for i in range(12)]]).reshape((3, 4))
print("Original Matrix:")
print(matrix)
To slice the matrix
along a particular column, you can specify the start and stop indices for the row and column. For example, let's extract all elements from columns 0 to 2 (inclusive):
# Slice the matrix along columns 0 to 2
sliced_matrix = matrix[:, :3]
print("nMatrix Sliced Along Columns 0-2:")
print(sliced_matrix)
The output will be:
Original Matrix:
[[0 1 2 3]
[4 5 6 7]
[8 9 10 11]]
Matrix Sliced Along Columns 0-2:
[[0 1 2]
[4 5 6]
[8 9 10]]
In the same way, you can slice along rows. The syntax is similar:
# Slice the matrix along rows 1 to 2
sliced_matrix = matrix[1:3, :]
print("nMatrix Sliced Along Rows 1-2:")
print(sliced_matrix)
The output will be:
Original Matrix:
[[0 1 2 3]
[4 5 6 7]
[8 9 10 11]]
Matrix Sliced Along Rows 1-2:
[[4 5 6 7]
[8 9 10 11]]
Slicing is an effective way to extract specific data subsets from your 2D list, which can be useful for various purposes such as data analysis or visualization.