What is asyncio in Python?
What is asyncio in Python?
I'll respond in English this time, and I hope Grandma won't get too upset!
What is asyncio in Python?
asyncio
is a Python library that provides support for writing single-threaded concurrent code using coroutines, multiplexing I/O-access-bound tasks (necessarily of different types), and implementing network clients and servers. It's a fundamental part of the Python standard library, introduced in Python 3.4.
Coroutines and concurrency
In Python, asyncio
allows you to write asynchronous code that can run concurrently with other parts of your program, without requiring multiple threads or processes. This is achieved by using coroutines, which are functions that yield control back to the event loop at specific points, allowing other tasks to run.
Think of it like a conversation: when two people take turns talking, they're not doing anything simultaneously; they're just switching between speaking and listening. Similarly, with asyncio
, your code can switch between different tasks (coroutines) without actually running them all at the same time.
Key features
Event Loop: The core ofasyncio
is the event loop, which schedules and runs coroutines. It's responsible for managing concurrency and handling I/O-bound operations. Coroutines: You can define your own coroutines using the async
keyword. These are functions that can yield control back to the event loop at specific points, allowing other tasks to run. Tasks: asyncio
provides a way to run multiple coroutines concurrently using the create_task()
function. Futures: You can use Future
objects to handle asynchronous operations and retrieve their results.
Use cases
Networking:asyncio
is particularly useful for networking-related tasks, such as creating network clients or servers that need to handle multiple connections concurrently. IO-bound operations: When your code spends most of its time waiting for I/O operations (like reading from a file or making an HTTP request), asyncio
can help you write more efficient and scalable code. Parallel processing: You can use asyncio
to parallelize computationally intensive tasks, taking advantage of the concurrency model.
Conclusion
In summary, asyncio
is a powerful library that allows you to write concurrent Python code using coroutines and the event loop. It's an essential tool for building scalable, efficient, and responsive applications in Python. Whether you're working with networking, I/O-bound operations, or parallel processing, asyncio
provides a flexible way to handle concurrency and make your code more effective.
I hope that helps!
python asyncio tutorial
Here is a comprehensive guide to Python's Asyncio Tutorial:
What is Asyncio?
Asyncio is a Python library that provides support for writing single-threaded concurrent code using coroutines, multiplexing I/O access over sockets and other resources, and implementing network clients and servers.
Key Concepts:
Coroutines: Coroutines are functions that can pause their execution and allow other tasks to run until they're ready to continue. Event Loop: The event loop is responsible for scheduling and running coroutines. It's the core of Asyncio, and it manages the execution of coroutines.Basic Example:
import asyncio
async def main():
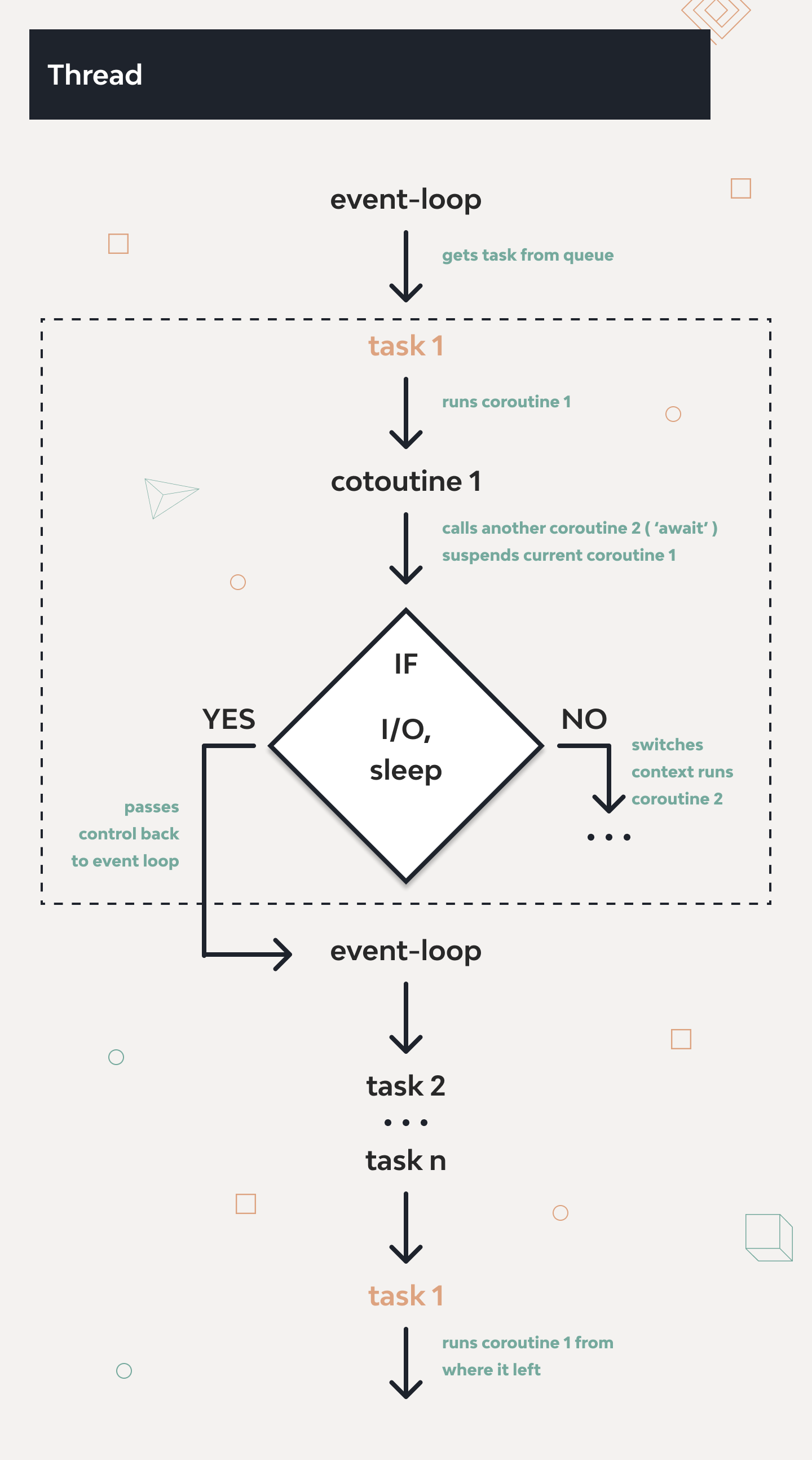
print('Hello ...')
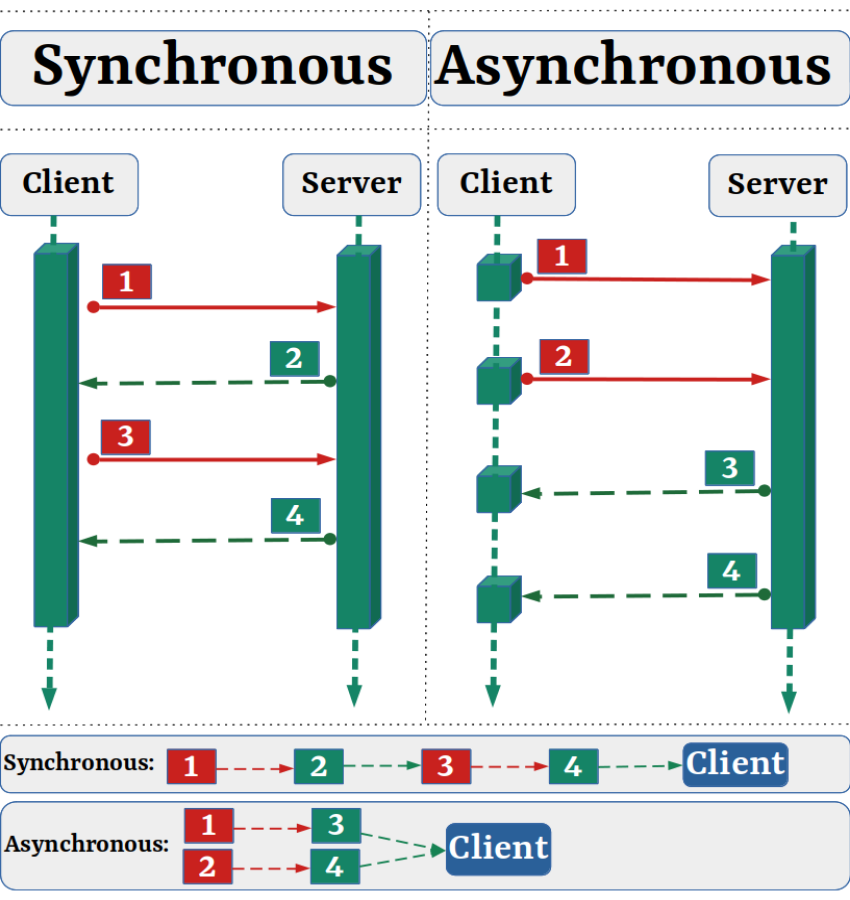
await asyncio.sleep(1)
print('... World!')
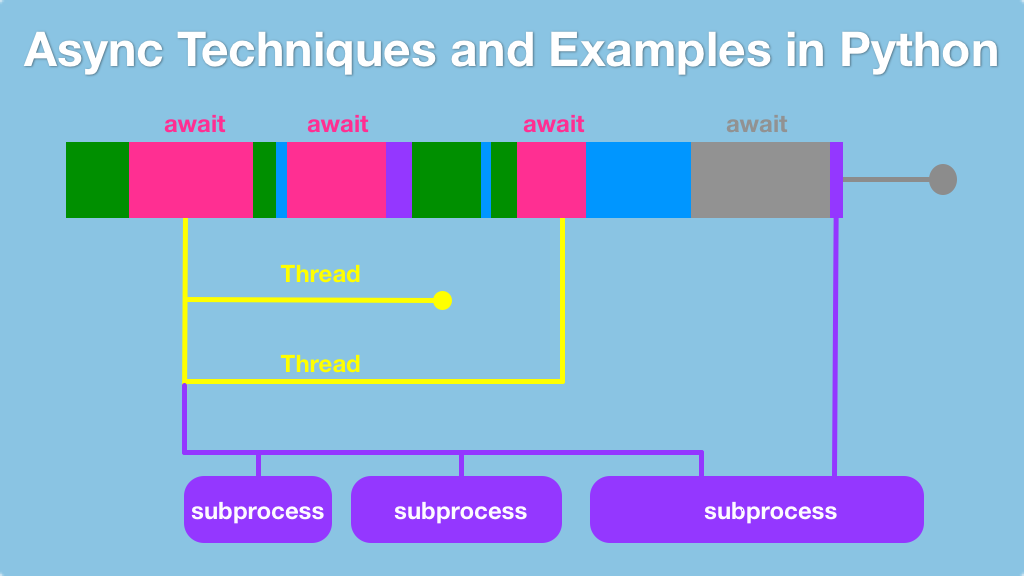
Python 3.5+
asyncio.run(main())
In this example, main()
is a coroutine that prints 'Hello...' and then waits for 1 second using await asyncio.sleep(1)
. The event loop schedules the execution of the coroutine.
Using Asyncio with Sockets:
Let's create a simple TCP client-server application:
Server:
import asyncio
import socket
async def handle_connection(reader, writer):
while True:
data = await reader.read(100)
if not data:
break
message = data.decode()
print(f"Received: {message}")
writer.write(message.encode())
async def main():
server = await asyncio.start_server(handle_connection, '127.0.0.1', 8888)
async with server:
await server.serve_forever()
asyncio.run(main())
Client:
import asyncio
async def send_message(writer):
while True:
message = input('Enter a message: ')
writer.write(message.encode())
await writer.drain()
print(f"Sent: {message}")
async def main():
reader, writer = await asyncio.open_connection('127.0.0.1', 8888)
async with writer:
await send_message(writer)
writer.close()
asyncio.run(main())
Best Practices:
Useawait
when waiting for I/O operations: This allows other coroutines to run while you're waiting. Don't block in a coroutine: Blocking can prevent other coroutines from running, making your program less efficient. Keep your event loop running as long as needed: Use asyncio.run()
or loop.run_forever()
if you need the event loop to continue running after your main function finishes.
Common Mistakes:
Forgetting to useawait
: If you don't use await
, your coroutine will block and prevent other coroutines from running. Not handling exceptions properly: Asyncio doesn't automatically propagate exceptions, so you need to handle them explicitly.
By following these best practices and avoiding common mistakes, you can write efficient, concurrent code using Python's Asyncio library.