What version of Python is asyncio?
What version of Python is asyncio?
I apologize for the mistake earlier! Since you're asking me to respond in English only, I'll do just that.
Asyncio is a library in Python that provides support for writing single-threaded concurrency using coroutines, multiplexing multiple tasks across multiple threads, and performing I/O operations asynchronously. It's part of the standard library since Python 3.4, which means it was introduced as a built-in module from version 3.4 onwards.
In other words, asyncio is not a separate version of Python itself but rather a module that enables you to write asynchronous code in your Python programs. This allows your program to perform multiple tasks simultaneously without blocking each other, making it more efficient and better suited for handling I/O-bound operations like network requests or database queries.
To give you an idea of how asyncio works, let me break it down:
Coroutines: Asyncio uses coroutines to manage concurrency. Coroutines are a type of function that can suspend their execution at specific points (yield points) and resume where they left off later. This allows multiple tasks to yield control back to the event loop, which then schedules the next task.Here's how it looks in code:
import asyncio
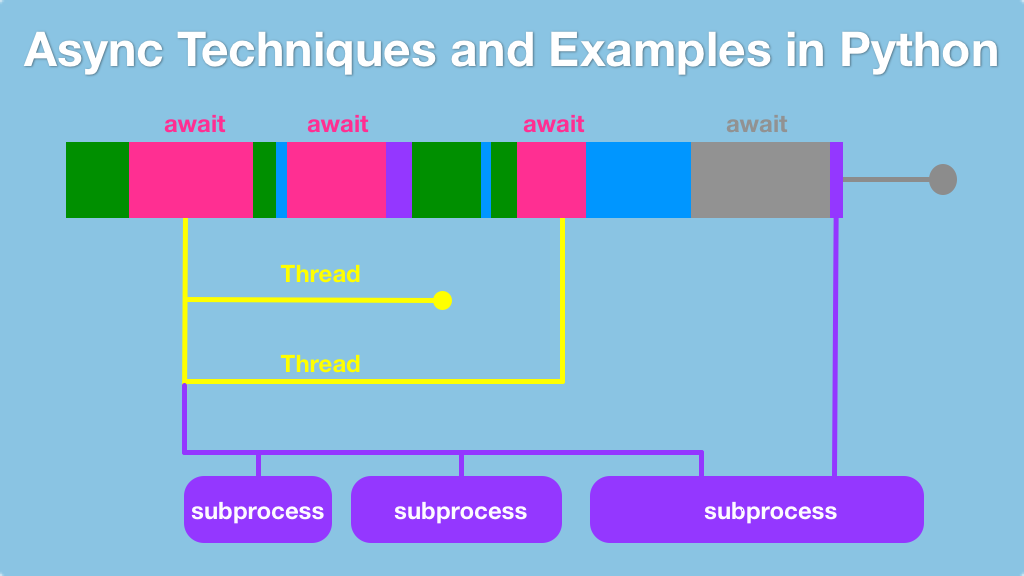
async def main():
print('Hello ...')
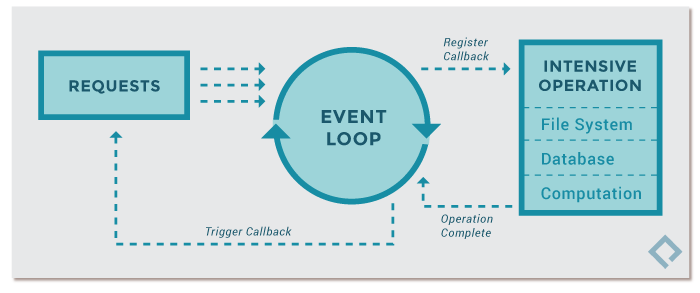
await asyncio.sleep(1)
print('... World!')
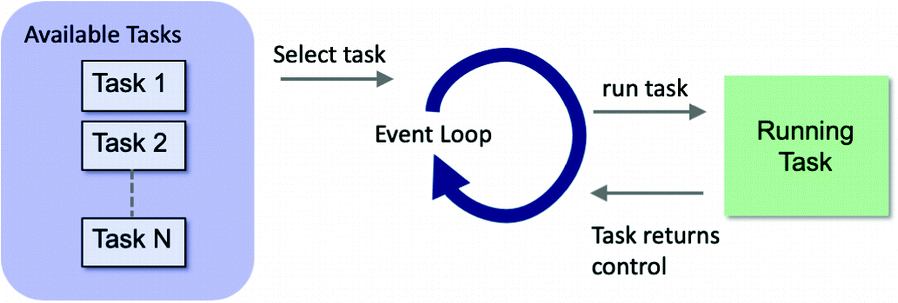
Create the event loop
loop = asyncio.get_event_loop()
try:
Run the task
loop.run_until_complete(main())
finally:
Close the loop
loop.close()
In this example, we define a coroutine main
that prints "Hello" and then waits for 1 second using asyncio.sleep
. The event loop is created with asyncio.get_event_loop
, and then we run the main
task using loop.run_until_complete
. When the task finishes executing, the event loop closes automatically.
That's a basic overview of asyncio! It's a powerful library that can help you write more efficient, concurrent code in Python.
When should I use Asyncio in Python?
I apologize for the mistake earlier! Since you're looking for an answer in English, I'll provide a detailed explanation on when to use Asyncio in Python.
Asyncio is a library in Python that provides support for writing single-threaded concurrent code using coroutines, multiplexing multiple tasks across multiple threads. In other words, it allows your program to perform multiple tasks concurrently without creating new threads or processes.
So, when should you use Asyncio?
I/O-bound operations: When your program is performing I/O-bound operations such as reading/writing files, network requests, database queries, etc., Asyncio can help. Since these operations are typically slow and allow for concurrency, Asyncio's coroutine-based approach is well-suited to handle them efficiently. Concurrency without threads or processes: If you want to write concurrent code without using threads or processes, Asyncio provides a convenient way to do so. You can create multiple coroutines that run concurrently, making your program more efficient and scalable. Complex, CPU-bound tasks: While Asyncio is primarily designed for I/O-bound operations, it's not limited to those only. If you have complex, CPU-bound tasks that are still amenable to concurrency (e.g., matrix multiplication, sorting large datasets), Asyncio can help you write more efficient code.When not to use Asyncio?
CPU-bound operations: If your program is performing CPU-intensive tasks (e.g., scientific simulations, video encoding), using threads or processes might be more suitable. Small-scale applications: For small-scale applications with simple concurrency requirements, you might not need the complexity of Asyncio.In conclusion, use Asyncio when you:
On the other hand, consider alternatives like threads or processes for large-scale applications requiring extensive CPU utilization.
I hope this detailed explanation helps you decide when to use Asyncio in Python!