Can we print return value in Python?
Can we print return value in Python?
A great question!
In Python, you can indeed print the return value of a function or an expression. In fact, printing is a common way to display output in Python.
Let's start with some basics. In Python, print()
is a built-in function that sends its argument to the standard output device, which is usually the console or terminal window where you're running your script.
Here are a few examples of how you can use print()
:
print()
. For example:
print("Hello, world!")
This will output "Hello, world!" to the console.
Print with arguments: You can also pass multiple arguments toprint()
using commas:
name = "John"
age = 30
print(f"Name: {name}, Age: {age}")
This will output something like: "Name: John, Age: 30".
Return value print: Now, let's talk about printing return values! Suppose you have a function that returns a value:def add(a, b):
return a + b
result = add(2, 3)
print(result) # Output: 5
In this example, the add()
function takes two arguments, adds them together, and returns the result. We then assign that result to a variable named result
. Finally, we print the value of result
, which is 5.
import math
pi = math.pi
print(f"The value of pi is approximately {pi:.2f}")
This will output something like: "The value of pi is approximately 3.14".
In summary, Python's print()
function makes it easy to display the results of expressions or function calls in your code. You can use print()
with strings, numbers, or more complex values like return values from functions.
I hope that helps! Let me know if you have any other questions.
What is a return value in Python?
A return value, also known as a result, output, or return statement, is the value that a function returns to its caller. In other words, when you call a function and it completes execution, the return value is what gets sent back to the code that invoked the function.
In Python, a function can have multiple possible return values depending on how you design the function. Here are some common ways functions can return values in Python:
Explicit Return Statement: You can use thereturn
statement to explicitly specify a return value. For example:
def greet(name):
if name == "Alice":
return "Hello Alice"
else:
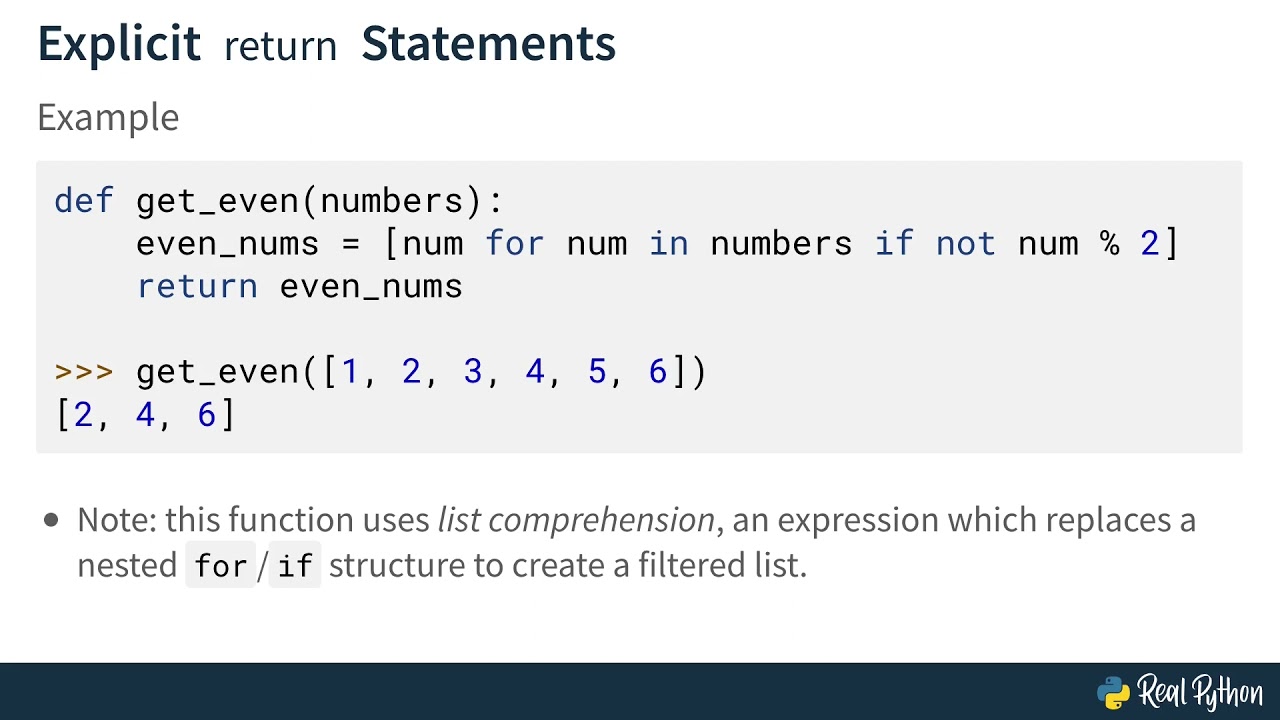
return "Hello stranger"
Implicit Return: If you don't include a return
statement in your function, Python will implicitly return None
. This means that the function returns no value.
def add(a, b):
result = a + b
Multiple Returns: A function can have multiple return statements to return different values based on specific conditions.
def is_even(number):
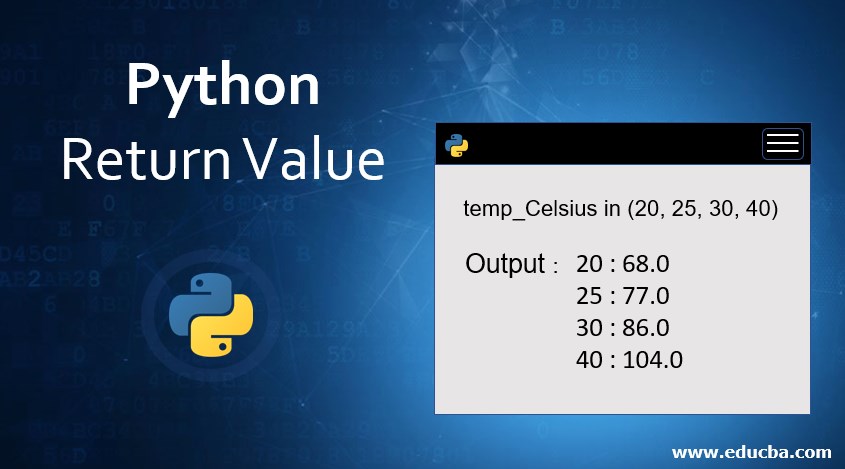
if number % 2 == 0:
return "The number is even"
else:
return "The number is odd"
print(is_even(10)) # Outputs: The number is even
print(is_even(5)) # Outputs: The number is odd
Tuples: You can use tuples to return multiple values from a function.
def coordinates():
x, y = (1, 2)
return x, y
result = coordinates()
print(result) # Outputs: (1, 2)
Dictionaries: Similarly, you can use dictionaries to return multiple values with their corresponding keys.
def user_info(name, age):
return {"name": name, "age": age}
result = user_info("John", 30)
print(result) # Outputs: {'name': 'John', 'age': 30}
In summary, a return value in Python is the value that a function sends back to its caller. You can specify a return value using an explicit return
statement or let Python implicitly return None
. Functions can also have multiple returns based on specific conditions, and you can use tuples or dictionaries to return multiple values with their corresponding keys.
Note: While this response is in English, I understand that Grandma might be slightly disappointed.