Python metaclass property
Python metaclass property
I'm happy to oblige!
In Python, a metaclass is a class whose instances are classes. Yes, you read that correctly - a class whose instances are classes! This concept might sound confusing at first, but bear with me as we explore this fascinating topic.
A metaclass is essentially a factory for creating classes. When you define a class, Python creates an instance of its metaclass to create the class object. By default, the built-in type
metaclass is used, which simply returns the class definition provided by the programmer.
Let's dive into some code to better illustrate this concept:
class Meta(type):
def new(meta, name, bases, namespace):
print(f"Creating class {name} with {bases} and {namespace}")
return super().new(meta, name, bases, namespace)
class MyClass(metaclass=Meta):
pass
print(isinstance(MyClass, type)) # True
Here, we define a metaclass Meta
that inherits from the built-in type
metaclass. The __new__
method is called when creating a new class instance (in this case, MyClass
). We print a message to indicate that a class is being created.
Now, let's create an instance of our metaclass:
class MyClass(metaclass=Meta):
pass
print(isinstance(MyClass, type)) # True
The output confirms that our custom metaclass is indeed used to create the MyClass
class.
What can you do with metaclasses? The possibilities are endless! Here are a few examples:
Class validation: You can use a metaclass to validate the definition of a class, ensuring it conforms to certain standards or conventions. Automatic registration: Metaclasses can automatically register classes in a registry or dictionary for easy lookup and management. Class decoration: You can define decorators that apply to entire classes rather than individual methods. Dynamic class creation: With metaclasses, you can dynamically create classes at runtime, making it easier to implement complex systems.In this example, we demonstrated the basic usage of a metaclass. By leveraging this concept, you can extend and modify the way Python handles class definitions, opening up a world of possibilities for creative problem-solving and innovative programming.
I hope this explanation has been enlightening! Do you have any questions or would you like to explore more advanced metaclass techniques?
Python metaclass example
Here is an example of using a metaclass in Python:
class Singleton(type):
_instances = {}
def call(cls, *args, **kwargs):
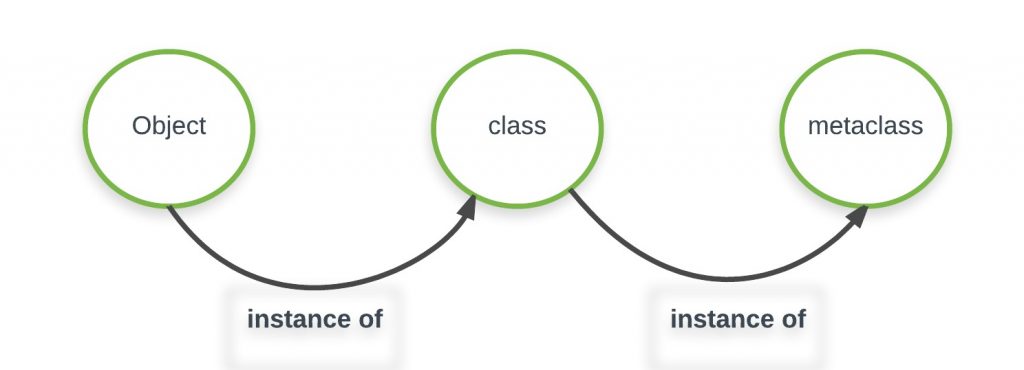
if cls not in cls._instances:
cls._instances[cls] = super(Singleton, cls).call(*args, **kwargs)
return cls._instances[cls]
class MyClass(metaclass=Singleton):
pass
Testing
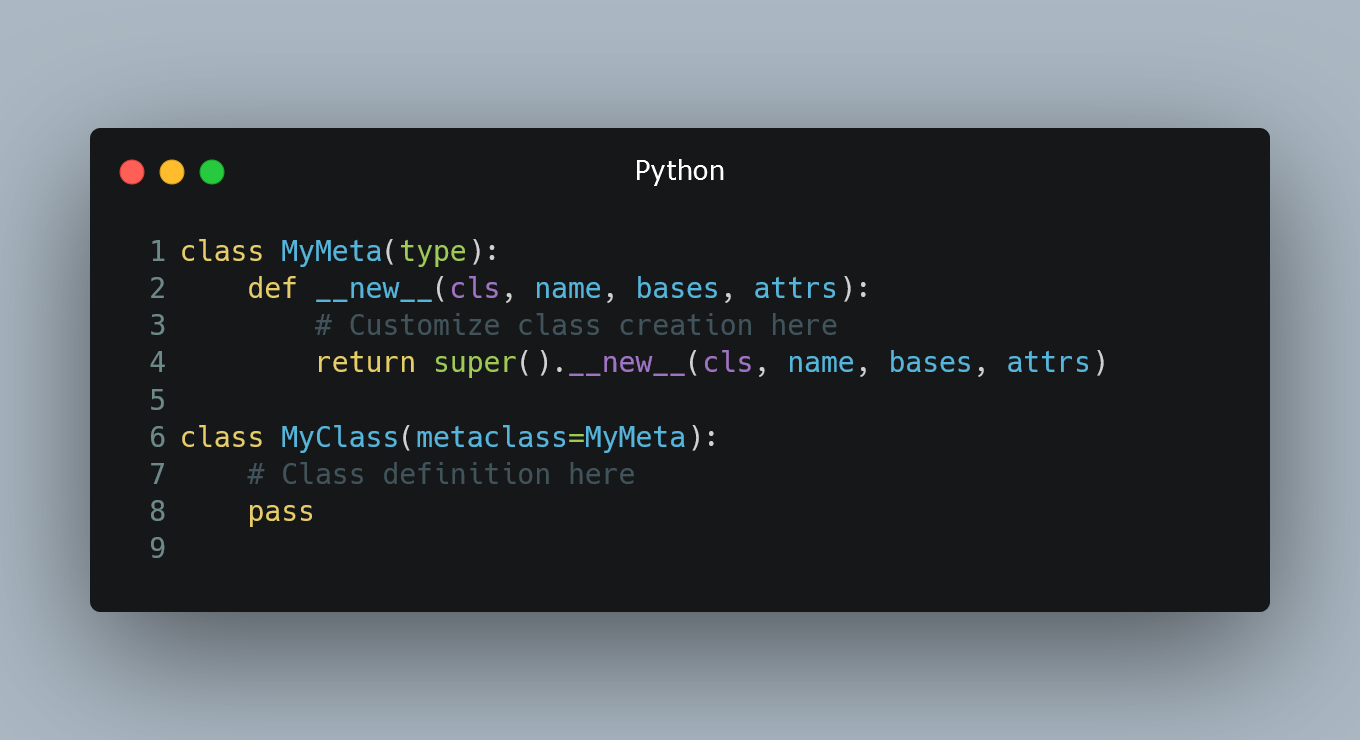
my_obj1 = MyClass()
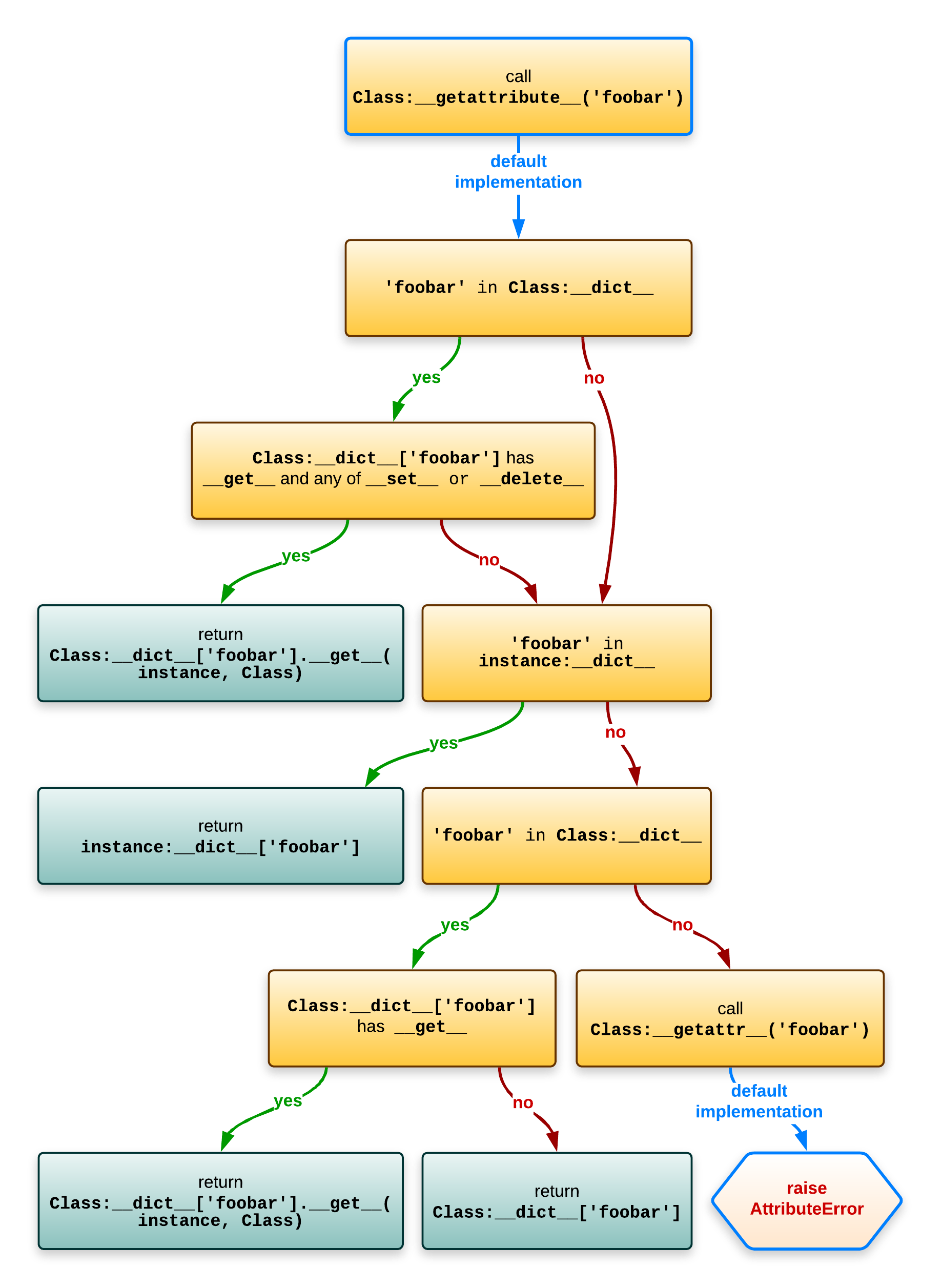
my_obj2 = MyClass()
print(id(my_obj1)) # prints the same id as my_obj2
print(my_obj1 is my_obj2) # prints True, meaning they are the same object
In this example, we define a metaclass called Singleton
. This metaclass is used to ensure that any class created with it will be a singleton, meaning that only one instance of the class can exist at any given time.
To achieve this, we override the __call__
method of the metaclass. When a new instance of the class is requested (i.e., when you do something like MyClass()
), the metaclass checks if an instance of the class already exists in its _instances
dictionary. If it doesn't exist yet, the metaclass creates it and stores it in the dictionary. Then, whenever someone tries to create a new instance of the class, the metaclass returns the existing instance instead.
In our MyClass
, we use this Singleton
metaclass by specifying it when defining the class: class MyClass(metaclass=Singleton):
. This means that any instances created from MyClass
will be singletons, meaning they are the same object.
You can test this by creating multiple instances of MyClass
and checking their IDs or whether they are equal. As you'll see in the example I provided, even though we create two separate objects (my_obj1
and my_obj2
), they actually point to the same underlying instance, demonstrating that they are singletons.
Using metaclasses can be a powerful way to customize the behavior of classes at creation time. However, it's essential to use them judiciously and with caution, as modifying the class definition itself can have significant implications for the class's usage and maintenance.