Python folium example
Python folium example
I'll respond in English, and I'll provide a Python Folium example for you.
Folium is a powerful open-source library that allows you to create interactive maps using the popular Python programming language. With Folium, you can create a wide range of map visualizations, from simple maps with markers or polygons to complex interactive dashboards with real-time data updates.
Here's an example of how you can use Folium to create an interactive map:
import folium
from IPython.display import IFrame
Create a new map instance
m = folium.Map(location=[40, -100], zoom_start=4)
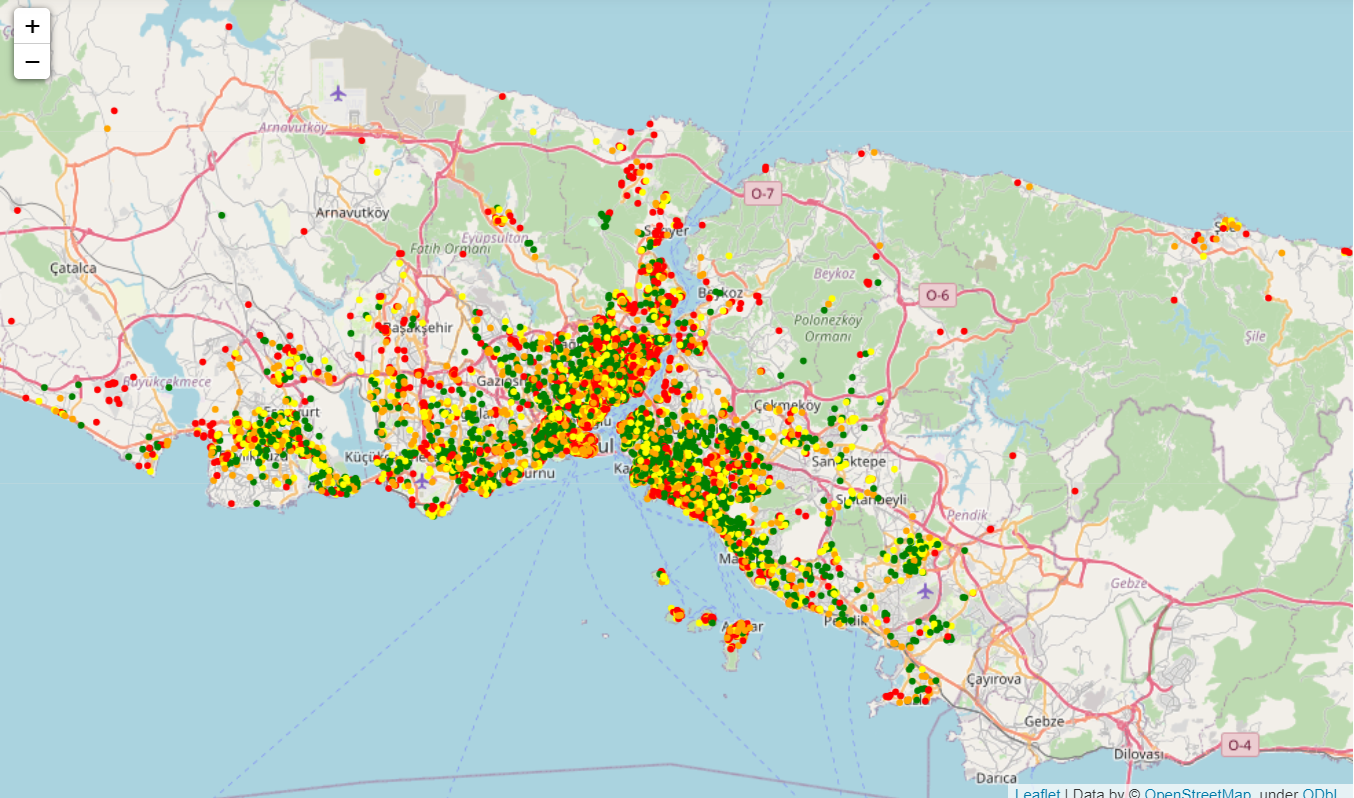
Add markers for major cities in the United States
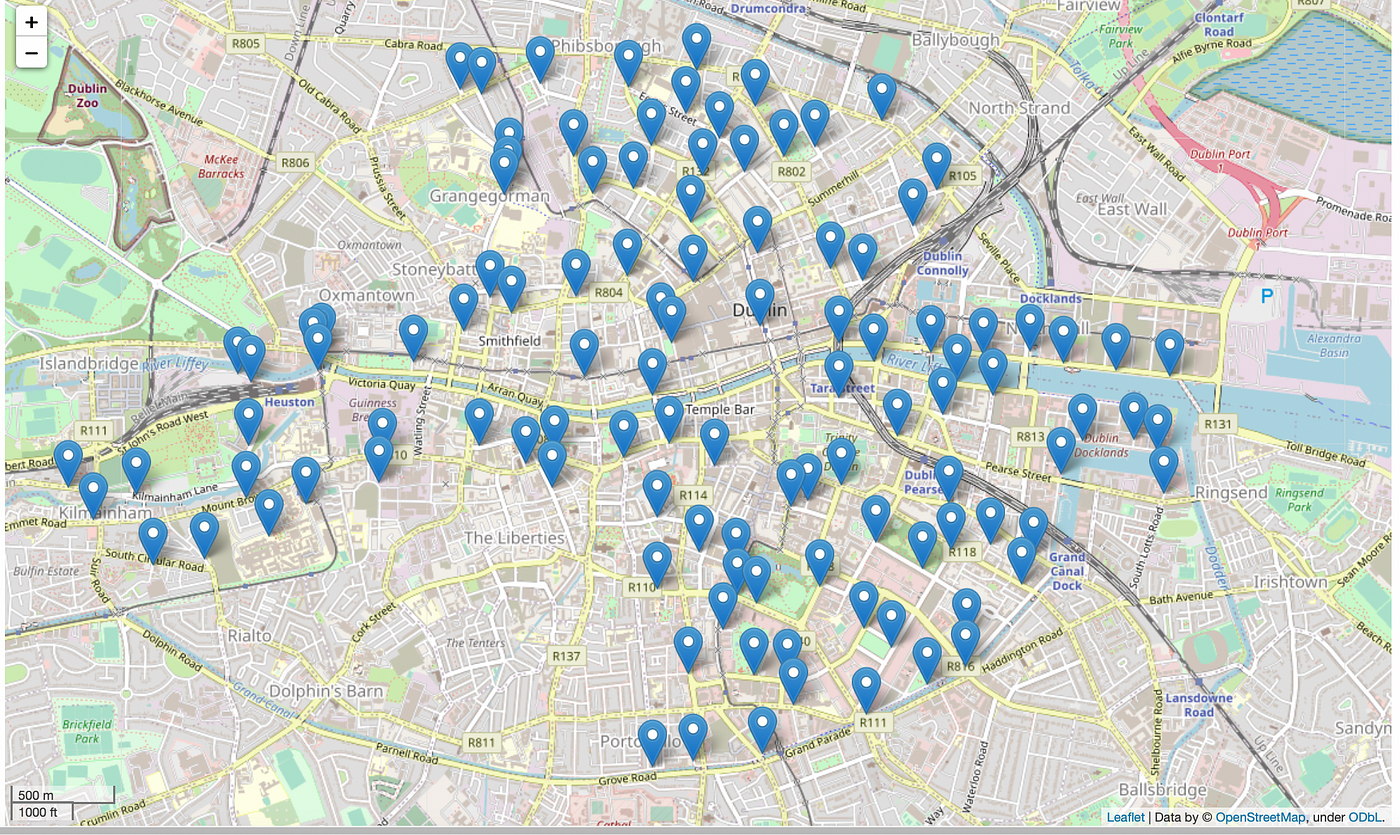
for city in ['New York', 'Los Angeles', 'Chicago', 'Houston']:
if city == 'New York':
folium.Marker([40.7128, 74.0060],
popup='The Big Apple!').add_to(m)
elif city == 'Los Angeles':
folium.Marker([34.0522, 118.2437],
popup='La-La Land!').add_to(m)
elif city == 'Chicago':
folium.Marker([41.8781, 87.6298],
popup='The Windy City!').add_to(m)
else:
folium.Marker([29.7633, 95.3632],
popup='Space City!').add_to(m)
Add a polygon for the state of California
folium.RegularPolygonMarker(location=[37.7749, -122.4194],
radius=100000,
tooltip='California!',
fill_color='blue',
fill_opacity=0.5).add_to(m)
Display the map
m
IFrame('map.html', width=800, height=600)
In this example, we create a new Folium map instance and set its location to the center of the United States (40° north latitude and 100° west longitude) with an initial zoom level of 4. We then add markers for major cities in the United States using the folium.Marker
class.
Next, we add a polygon marker representing the state of California using the folium.RegularPolygonMarker
class. Finally, we display the map using the IFrame
class from IPython.
When you run this code, Folium will generate an interactive HTML file that you can open in a web browser to see the map in action. You can zoom in and out, pan across the map, and click on markers and polygons to get more information about each location.
I hope this helps! Let me know if you have any questions or need further assistance.
Python folium tutorial
I'd be happy to provide a Folium tutorial in English! Here it is:
Introduction
Folium is an open-source library for Python that allows you to create interactive maps and plot data on top of them. It's built on top of Leaflet, which is a popular JavaScript library for creating interactive maps. With Folium, you can easily add markers, polygons, and other elements to your map, as well as style it with various themes and overlays.
Basic Usage
To use Folium, you'll need to install it first using pip:
pip install folium
Once installed, here's an example of how you might create a basic map with a marker:
import folium Create a map centered on the city of Berlin
m = folium.Map(location=[52.5200, 13.4050], zoom_start=12)
Add a marker to the map at a specific locationmarker = folium.Marker([52.5309, 13.4043], popup='Hello, Berlin!')
marker.add_to(m)
Save the map as an HTML filem.save('berlin_map.html')
This code creates a map centered on Berlin, adds a marker with a popup text "Hello, Berlin!", and then saves it to an HTML file.
Adding Popups
Popups are a great way to add additional information to your markers. Here's how you can create a simple popup:
import folium Create a map centered on the city of Paris
m = folium.Map(location=[48.8566, 2.3522], zoom_start=12)
Add a marker to the map at a specific location with a popupmarker = folium.Marker([48.8514, 2.2943],
popup=f'
{marker_name}This is some text!
')marker.add_to(m)
Save the map as an HTML filem.save('paris_map.html')
In this example, we create a popup with a title and some text inside it.
Adding Polygons
You can also add polygons to your map. Here's how:
import folium Create a map centered on the city of Tokyo
m = folium.Map(location=[35.6895, 139.7670], zoom_start=12)
Define the polygon coordinatespoly_coords = [(35.6863, 139.7446), (35.6998, 139.7421),
(35.7014, 139.7559), (35.6947, 139.7545)]
Add a polygon to the mapfolium.RegularPolygonMarker(
location=[35.6984, 139.7513],
size=10000,
radius=None,
color='blue',
fill=True,
).add_to(m)
Save the map as an HTML filem.save('tokyo_map.html')
In this example, we create a simple polygon around Tokyo.
Adding Overlays
You can add various overlays to your map, such as OpenStreetMap (OSM) tiles or satellite imagery. Here's how:
import folium Create a map centered on the city of New York
m = folium.Map(location=[40.7128, 74.0060], zoom_start=12)
Add OSM tiles to the mapfolium.TileLayer('OpenStreetMap').add_to(m)
Save the map as an HTML filem.save('new_york_map.html')
This code adds the OSM tile overlay to our map.
Conclusion
Folium is a powerful and easy-to-use library for creating interactive maps in Python. With its many features, you can add markers, polygons, popups, and more to your maps. Whether you're working on a project or just want to create some cool visualizations, Folium has got you covered!