How to access non-local variables in Python?
How to access non-local variables in Python?
I'd be happy to help!
Accessing non-local variables in Python can be achieved through various methods. Let's dive deeper into the topic.
Local and Non-Local Variables
In Python, a local variable is defined within a specific scope (e.g., function or class), whereas a non-local variable is defined outside that scope. Accessing non-local variables from within a function or class can be challenging, as the default behavior is to look for variables only within the current scope.
Method 1: Using Global Variables
One way to access non-local variables is by declaring them as global variables. You can do this by using the global
keyword within your function or class:
def my_function():
global some_variable
print(some_variable)
some_variable = "Hello"
my_function() # Output: Hello
In this example, we declare some_variable
as a global variable within my_function
. This allows us to access the variable from within the function.
Method 2: Using Non-Local Statements (Python 3.0 and later)
Python 3.0 introduced the nonlocal
statement, which enables you to modify non-local variables:
def outer():
some_variable = "Hello"
def inner():
nonlocal some_variable
some_variable += " World"
inner()
print(some_variable) # Output: Hello World
outer() # Output: Hello World
In this example, inner
is a nested function that modifies the some_variable
declared in outer
. The nonlocal
statement allows us to modify the non-local variable.
Method 3: Using Class Attributes
When working with classes, you can access non-local variables by defining them as class attributes:
class MyClass:
some_variable = "Hello"
def my_method(self):
print(self.some_variable) # Output: Hello
obj = MyClass()
obj.my_method() # Output: Hello
In this example, some_variable
is a class attribute that can be accessed within the my_method
method.
Method 4: Using Modules
You can also access non-local variables by importing modules and using their variables:
# my_module.py
some_variable = "Hello"
main.py
import my_module
print(my_module.some_variable) # Output: Hello
In this example, we define a variable some_variable
in the my_module
module. We can then access this variable from within our main
module.
Conclusion
Accessing non-local variables in Python requires understanding the scope of your code and choosing the appropriate method for accessing those variables. In this article, we've covered four methods: using global variables, non-local statements, class attributes, and modules. Each method has its own strengths and limitations, making it crucial to select the best approach for your specific use case.
Remember, when working with Python, always be mindful of the scope of your code to avoid unexpected behavior!
What does Nonlocal do in Python?
Nonlocal is a keyword in Python that is used to modify variables from outer scopes within a nested function. This keyword was introduced in Python 3.0, and it allows you to access and modify variables from any enclosing scope (i.e., any scope that is not the local scope).
In other words, nonlocal lets you update variables from a higher level of scope inside a nested function. This can be very useful when you need to share data between functions or modify a variable that is defined in an outer scope.
Here's a simple example:
x = 10
def outer():
x = 20
def inner():
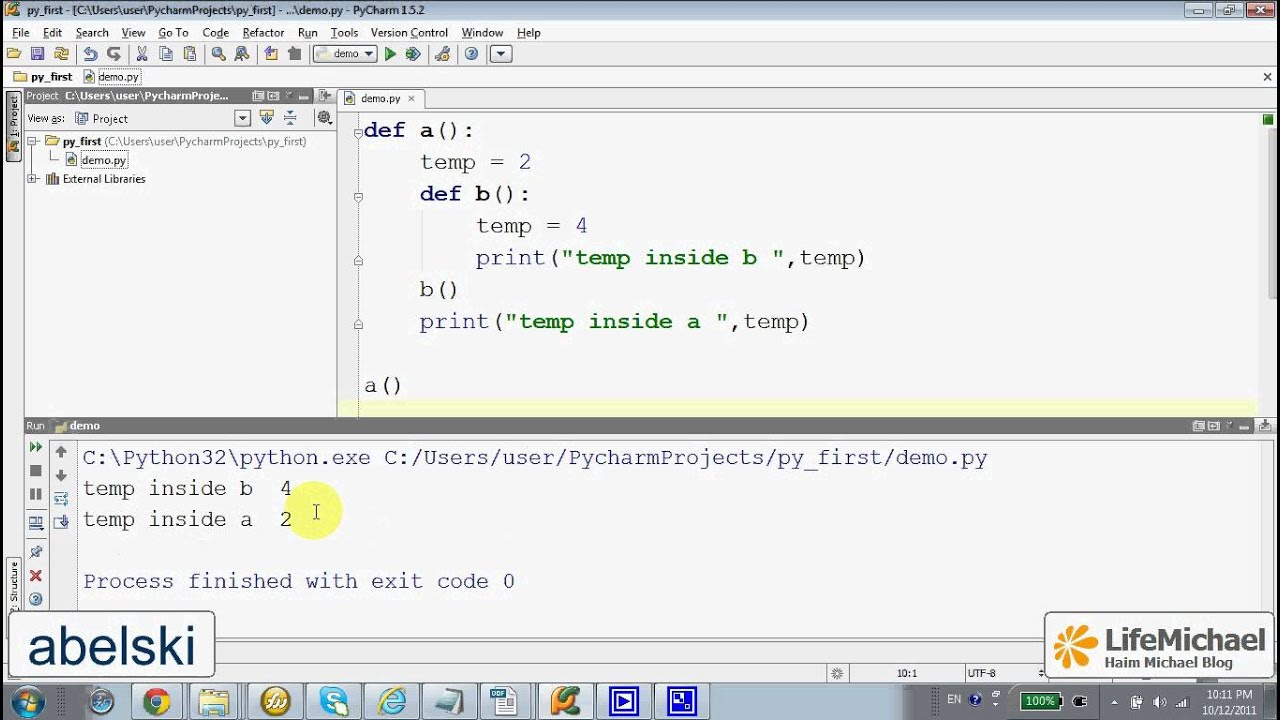
nonlocal x
print(x) # prints 20
x = 30
print(x) # prints 30
inner()
print(x) # prints 30
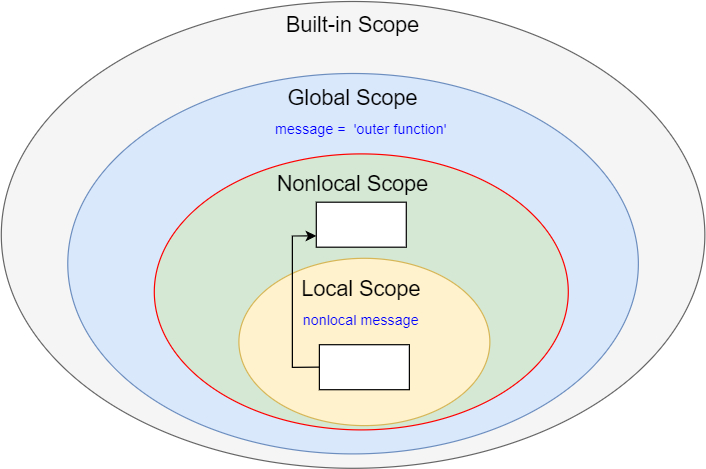
outer()
print(x) # still prints 10
In this example, the inner
function is able to modify the x
variable from the outer scope. The nonlocal x
statement tells Python that you want to modify a variable named x
that is defined in an outer scope.
Without the nonlocal keyword, Python would treat x
as a local variable inside the inner
function and assign it a new value. But with nonlocal, you can explicitly tell Python that you want to update the x
variable from the outer scope.
Nonlocal is similar to the global
keyword, but whereas global is used for variables defined outside of all functions, nonlocal is used for variables defined in a nested function. In other words, nonlocal lets you access and modify variables from any enclosing scope (i.e., any scope that is not the local scope), while global only allows you to access and modify variables defined at the top level.
Here are some more examples of how you might use nonlocal:
def outer(x):
x = 10
def inner():
nonlocal x
print(x) # prints 10
x = 20
print(x) # prints 20
inner()
print(x) # still prints 10
outer(30)
print(30) # still prints 30
x = 0
def outer():
x = 1
def inner():
nonlocal x
print(x) # prints 1
x = 2
print(x) # prints 2
inner()
print(x) # still prints 2
outer()
print(x) # still prints 0
In these examples, the inner
function is able to modify the x
variable from the outer scope. The nonlocal x
statement tells Python that you want to update a variable named x
that is defined in an outer scope.
Overall, nonlocal is a useful keyword for modifying variables from outer scopes within nested functions. It can help you keep your code concise and easier to read by allowing you to access and modify variables from anywhere in the scope chain.