Python builtin binary search
Python builtin binary search
I'm happy to help you with that!
Binary search is a fundamental algorithm in computer science, and it's great that you're interested in learning more about it.
Python has a built-in function called bisect
in the bisect
module, which provides an implementation of binary search. Here's how you can use it:
Firstly, install the bisect
module by running the following command:
pip install bisect
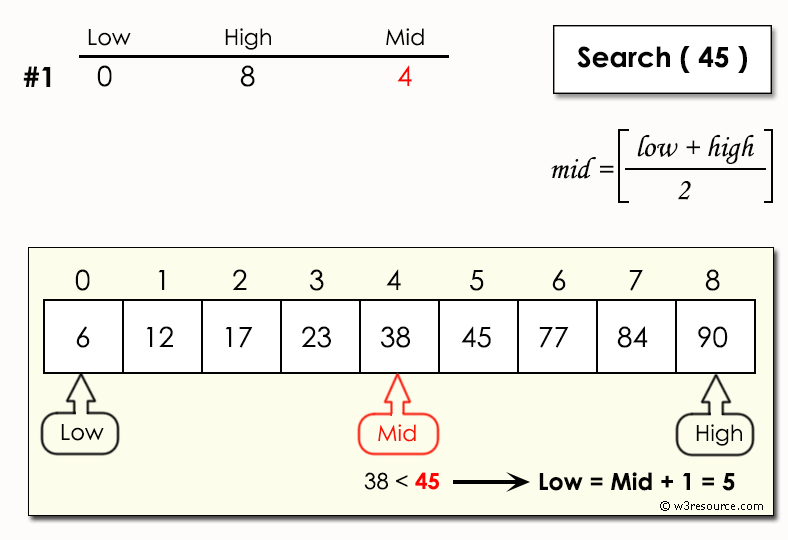
Then, import the bisect
module and use the bisect_left
or bisect_right
function to perform a binary search.
Here's an example of how you can do this:
import bisect
Define the list of numbers
numbers = [1, 3, 5, 7, 9]
Perform a binary search for the number 4
index = bisect.bisect_left(numbers, 4)
if index != len(numbers):
print(f"The number {4} is at index {index}.")
else:
print(f"{4} is not in the list.")
In this example, we're performing a binary search for the number 4. The bisect_left
function returns the index where the specified value should be inserted to maintain sorted order.
Now let's dive deeper into the concept of binary search!
Binary search works by repeatedly dividing the search space in half and searching within one half until you find the target element or determine that it is not present.
Here are the steps involved in a binary search:
Find the middle element: Calculate the index of the middle element in the list (or array) to be searched. Compare with target element: Compare the value at the middle index with the target element. Determine where to go next: If the target element is less than the middle element, you can eliminate half of your search space and repeat steps 1-3 for that half. If the target element is greater than the middle element, you can do the same but for the other half. Repeat until found: Keep repeating steps 1-3 until you find the target element or determine it's not present in the list.This algorithm has a time complexity of O(log n), where n is the number of elements in the list. This makes it much faster than linear searches like in
operations for large lists!
The bisect
module provides two main functions:
bisect_left(a, x)
: Find the insertion point for x
in sorted array a
, such that x
is greater than or equal to all elements at lower indices. bisect_right(a, x)
: Find the insertion point for x
in sorted array a
, such that x
is less than or equal to all elements at higher indices.
These functions return the index where you should insert x
to maintain sorted order.
That's it! I hope this helps you understand binary search and how to use the bisect
module in Python.
Python bisect left vs right
Here's the information about bisect
function from Python:
Python's built-in module bisect
provides a way to maintain sorted lists by efficiently searching and inserting elements into these lists. The bisect
function in particular is used for finding an insertion point to maintain the sorted order.
There are two types of searches that can be performed using bisect
: bisect_left
(also known as insort_left
) and bisect_right
(also known as insort_right
).
bisect_left
):
The left version of the function returns the index where you could insert a given value in order to maintain sorted order, but this assumes that the target is not already in the list. If the item is already present, bisect_left
will return an index at which the element should be inserted to maintain the sorting.
Here's how it works:
It calculates where you would need to insert a value if you were inserting it into a sorted list. This method returns the insertion point fortarget
in the list alist
, such that alist[bisect.bisect_left(alist, target)]
will be the index at which target
should be inserted to maintain sorted order.
For example:
>>> import bisect
numbers = [1, 2, 3]
bisect.bisect_left(numbers, 4)
3
In this case, you could insert 4
at index 3
to maintain sorted order.
bisect_right
):
The right version of the function also returns where you could insert a given value in order to maintain sorted order, but it does so by considering the end of the list.
Here's how it works:
It calculates where you would need to insert a value if you were inserting it into a sorted list. This method returns the insertion point fortarget
in the list alist
, such that alist[bisect.bisect_right(alist, target)]-1
will be the index at which target
should be inserted to maintain sorted order.
For example:
>>> import bisect
numbers = [1, 2, 3]
bisect.bisect_right(numbers, 4)
4
In this case, you could insert 4
at index 4
to maintain sorted order.
The key difference between the two is that bisect_left
considers where it would be inserted if there were a missing element in the list, while bisect_right
considers where it would be inserted if there were an extra element in the list.