List of membership operators in python
List of membership operators in python
Here is the list of membership operators in Python:
Membership Operators
In Python, membership operators are used to check if a value exists within another value or container. The membership operators are in
and not in
.
Example:
fruits = ['apple', 'banana', 'cherry']
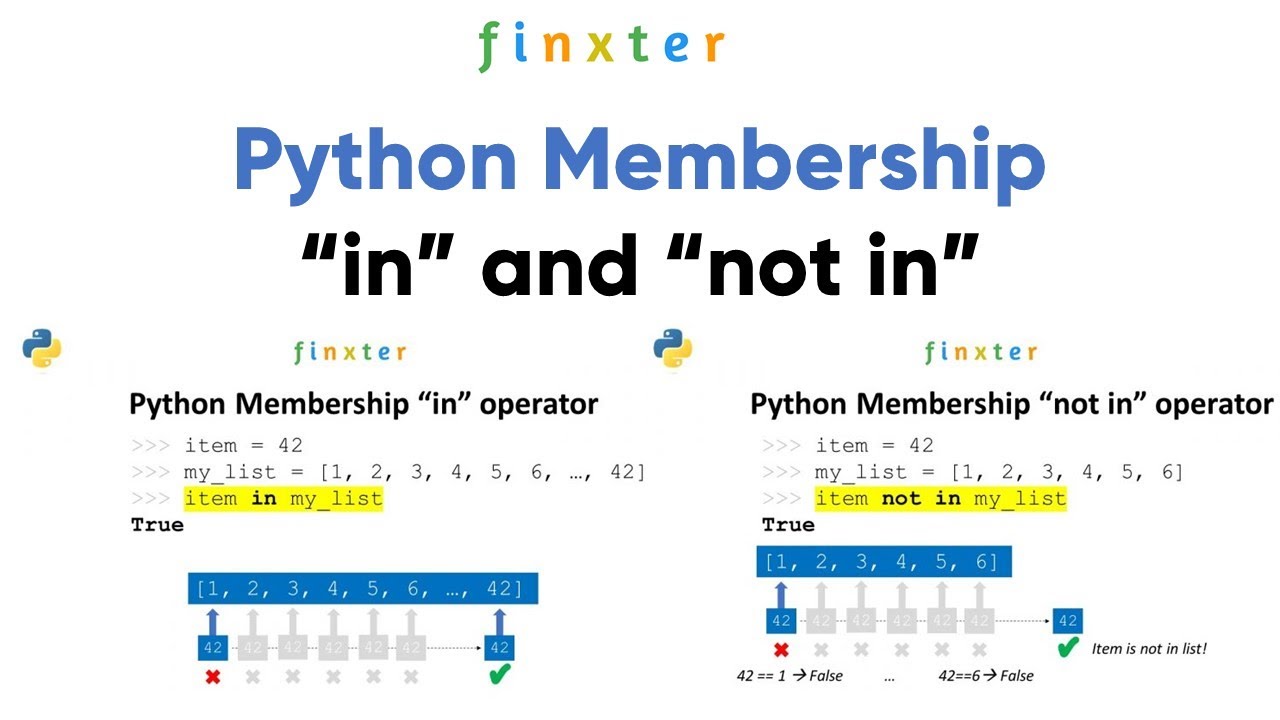
if 'banana' in fruits:
print("Banana is in the list.")
else:
print("Banana is not in the list.")
print('apple' in fruits) # Outputs: True
print('pear' in fruits) # Outputs: False
not in: This operator checks if a value is NOT present within a sequence (such as a list, tuple, or string), dictionary, set, or other iterable.
Example:
fruits = ['apple', 'banana', 'cherry']
if 'grapes' not in fruits:
print("Grapes are not in the list.")
else:
print("Grapes are in the list.")
print('apple' not in fruits) # Outputs: False
print('pear' not in fruits) # Outputs: True
Examples with Dictionaries
In addition to checking membership within sequences and sets, in
and not in
can also be used with dictionaries.
Example:
person = {'name': 'John', 'age': 30}
if 'age' in person:
print("Age is a key in the dictionary.")
else:
print("Age is not a key in the dictionary.")
print('name' in person) # Outputs: True
print('city' in person) # Outputs: False
Examples with Sets
in
and not in
can also be used with sets.
Example:
numbers = {1, 2, 3}
if 2 in numbers:
print("2 is a member of the set.")
else:
print("2 is not a member of the set.")
print(4 in numbers) # Outputs: False
print(3 in numbers) # Outputs: True
These are all the membership operators available in Python.
Why are in and not in operators called membership operators in Python?
The in
and not in
operators in Python are indeed referred to as "membership operators". This is because they are used to check if a value or element exists within a sequence, such as a list, tuple, set, or dictionary.
In more detail, the in
operator checks if a specified value is present in a given iterable (like a list, tuple, or set), while the not in
operator checks for the absence of that value. For example:
fruits = ['apple', 'banana', 'orange']
print('banana' in fruits) # Output: True
print('grape' not in fruits) # Output: True
phonebook = {'John': '12345', 'Jane': '67890'}
print('John' in phonebook) # Output: True
print('Mike' not in phonebook) # Output: True
In each of these cases, the membership operators are used to determine whether a specific value (or key-value pair) is contained within the specified iterable or dictionary.
The in
and not in
operators can be used with various types of sequences and data structures in Python. For instance, you might use them to check if an element exists in a list, set, tuple, or even a string. Similarly, you could utilize these operators to verify the presence or absence of a key-value pair within a dictionary.
It's worth noting that membership testing can have significant implications for your code's behavior and efficiency. When working with large data sets, it's essential to consider the time complexity and memory usage associated with membership testing. This is especially important when dealing with complex algorithms or massive datasets.
In conclusion, the in
and not in
operators are fundamental membership operators in Python that allow developers to check if specific values exist within sequences or dictionaries. By mastering these operators, you'll be better equipped to craft efficient and effective code for a wide range of applications and scenarios.