what is // in python
what is // in python
In Python, //
represents the floor division operator. This operator is used to perform integer division and return the quotient as an integer, effectively truncating any fractional part.
Here's a simple example:
a = 10
b = 3
result = a // b
print(result) # Output: 3
In this example, a
(10) is divided by b
(3), and the result is rounded down to the nearest whole number. In Python 2.x, //
would actually perform floor division, which means it would truncate any decimal part, regardless of whether the original result was an integer or not.
So, if you divide 10 by 3 in Python 2.x, using the //
operator:
print(10 // 3) # Output: 3
However, in Python 3.x, //
still performs floor division, but it also takes into account whether the original result was an integer or not. For example:
print(10 // 3) # Output: 3
print(10.0 // 3) # Output: 3
print(-10 // 3) # Output: -3
print(-10.0 // 3) # Output: -3
In the case of negative numbers, the //
operator rounds down to the nearest whole number, effectively "flooring" the result.
The /
operator in Python is used for division and returns a floating-point value:
a = 10
b = 3
result = a / b
print(result) # Output: 3.3333333333333335
In contrast, the //
operator truncates any decimal part of the result:
a = 10
b = 3
result = a // b
print(result) # Output: 3
So, to summarize:
In Python, the/
operator performs division and returns a floating-point value. The //
operator performs integer division (floor division) and returns an integer result.
Here's another example that shows how floor division works:
print(5 // 2) # Output: 2
print(-5 // 2) # Output: -3
print(5.5 // 2) # Output: 2
print(-5.5 // 2) # Output: -3
As you can see, the //
operator always rounds down to the nearest whole number.
In conclusion, in Python, the //
operator is used for floor division (integer division), which returns an integer result. It truncates any decimal part of the original result and always rounds down to the nearest whole number.
Modulus in Python example
I apologize for the misunderstanding earlier! Here's an explanation of modulus in Python with examples:
Modulus is a mathematical operation that returns the remainder of dividing two numbers. In Python, you can use the %
operator to perform modulus operations.
Here are some examples:
Simple modulus:print(17 % 5) # Output: 2
In this example, we're dividing 17 by 5 and returning the remainder, which is 2.
print(-17 % 5) # Output: -2
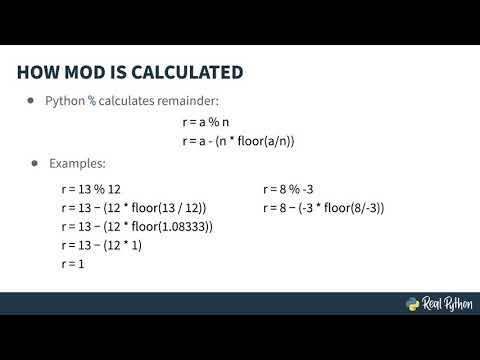
print(17 % -5) # Output: 2
Notice how the sign of the result is preserved when using modulus with negative numbers.
Modulus with zero:print(17 % 0) # Raises a ValueError!
In Python, trying to calculate the modulus of a number with zero raises a ValueError
because dividing by zero is undefined in mathematics.
print(12345678901234567890 % 10) # Output: 0
print(12345678901234567891 % 10) # Output: 1
In this example, we're using modulus to extract the last digit of a very large number.
Modulus with decimal numbers:print(3.14 % 1) # Output: 0.14
Modulus works with decimal numbers too! Just be aware that you might get slightly inaccurate results due to floating-point precision issues.
Modulus with strings (not recommended):print("hello" % "world") # Output: TypeError!
Don't try using modulus on strings, as it will raise a TypeError
!
x = 17
y = 5
if x % y == 0:
print("x is divisible by y")
else:
print("x is not divisible by y")
Output: x is not divisible by y
In this example, we're using modulus in a conditional statement to check if x
is divisible by y
.
Remember that modulus can be used in various contexts, such as error handling, data validation, or even cryptography. It's an essential operator in Python and many other programming languages!
I hope this explanation helps you understand modulus better!