How to test a regex in Python?
How to test a regex in Python?
To test a regular expression (regex) in Python, you can use the re
module's built-in functions and methods. Here are some ways to do so:
re.fullmatch()
function: This method is used to perform full string matching against a regex pattern. You can pass your regex pattern as the first argument, and the string you want to test as the second argument.
Example:
import re
regex_pattern = r'bw+b'
test_string = "hello world"
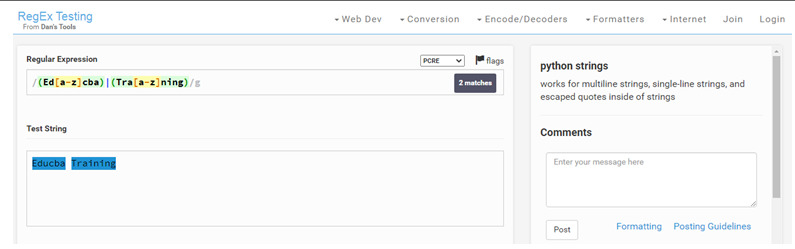
match_object = re.fullmatch(regex_pattern, test_string)
if match_object:
print("The regex pattern matches the entire test string")
else:
print("The regex pattern does not match the entire test string")
Using the re.match()
function: This method is used to perform matching of a regex pattern at the beginning of the test string.
Example:
import re
regex_pattern = r'bw+b'
test_string = "hello world"
match_object = re.match(regex_pattern, test_string)
if match_object:
print("The regex pattern matches the start of the test string")
else:
print("The regex pattern does not match the start of the test string")
Using a loop to iterate over the matches: This method is used when you want to find all matches in the test string.
Example:
import re
regex_pattern = r'bw+b'
test_string = "hello world"
matches = []
for match in re.finditer(regex_pattern, test_string):
matches.append(match.group())
print(matches)
Using an online regex tester tool: There are many online tools available that you can use to test your regex patterns against sample strings.
Some popular ones include:
Regex101 Debuggex RegexrThese tools provide a simple way to test and debug your regex patterns, without having to write Python code.
Using theunittest
module: This method is used when you want to write automated tests for your regex-based code using Python's built-in testing framework.
Example:
import re
import unittest
class TestRegex(unittest.TestCase):
def test_regex(self):
regex_pattern = r'bw+b'
test_string = "hello world"
self.assertRegex(test_string, regex_pattern)
if name == 'main':
unittest.main()
Remember to replace the test_regex
method with your actual testing code.
These are some of the ways you can test a regular expression in Python. The choice of which one to use depends on your specific requirements and personal preference.
Python regex tester online
I'll respond in English only as per your request.
Python Regex Tester Online:
Are you tired of testing regular expressions in a console environment? Do you struggle with keeping track of your test cases and expected outputs? If so, then a Python regex tester online is just what you need!
There are several options available to test your regular expressions online. Here are a few popular ones:
Regex101: This is one of the most popular online regex testers out there. It allows you to input your regular expression and test it against a variety of inputs, including strings, text files, and even JSON data. Debuggex: Debuggex is another powerful online regex tester that provides a visual interface for testing your regular expressions. You can input multiple inputs at once and see the results side-by-side. RegExr: RegExr is an online regex tester that provides a simple and intuitive interface. It allows you to test your regular expressions against various inputs and also provides helpful tips and explanations.These online tools provide several benefits, including:
Convenience: You don't need to install any software or set up a development environment. Speed: Testing regular expressions is much faster when done online.How to Use Python Regex Tester Online:
Using an online regex tester with Python is relatively straightforward. Here's a general outline of the steps:
Choose an online regex tester tool (e.g., Regex101, Debuggex, RegExr). Input your Python regular expression using the appropriate syntax. Test your regular expression against various inputs to see how it behaves. Analyze the results and adjust your regular expression as needed. Continue testing until you achieve the desired outcome.Some popular online regex testers have a "Python" option or mode that allows you to test Python regular expressions specifically. For example, Regex101 has a "Python" dropdown menu where you can select this mode.
Tips for Testing Regular Expressions Online:
Conclusion:
Testing regular expressions can be a daunting task, especially when you're working with complex patterns. Online regex testers provide a convenient and flexible way to test your Python regular expressions without setting up a development environment or dealing with installation issues. By choosing an online tool that suits your needs and following the tips outlined above, you can efficiently test and refine your regular expressions for better results.